Over the 20 years I’ve been building email marketing software, I’ve used or come across almost every SMTP service on the market. While plenty of articles exist comparing the pricing and other facets of SMTP relay services, none offer an in-depth technical comparison, which is why I’ve done a deep dive to give you my expert insights below. It’s high time someone that is a technical expert in the SMTP protocol reviewed the popular commercial SMTP services.
If you Google “SMTP service,” you’ll find a plethora of companies offering services, and in this article, we’ll examine the technical aspects of the most popular ones. This review does not take into account pricing, simply because you can easily look that up on each provider’s website.
The technical aspects I’ll examine are:
- The sign-up process: Can you sign up and start sending right away? Is there a human-review required before you’re allowed to start sending?
- Sending limits: Can you send a small 1,000-person campaign right away, or does the service throttle you until you’re a trusted user? (I’ll actually send 1,000 emails to my own users through the service and see what happens.)
- Pass-through or not? Is the SMTP service a pass-through SMTP service that sends your email along just as it was relayed to it, or does it alter the MAIL-FROM headers and add any other information to the headers or body before it transmits the email to the recipient’s MTA? Some services force their own open-tracking pixel into the emails.
- Out-of-the-box SPF and DKIM setup and which domains are used for SPF and DKIM compliance. If it’s a pass-through service, this won’t apply, because it’s impossible to have a pass-through service that is out-of-the-box SPF and DKIM compliant. If it’s not a pass-through service, does it make sure your emails are SPF and DKIM complaint off the bat by using the service’s own domain rather than yours?
- “From” line flexibility: Will it let you send “from” an address @gmail.com?
- Custom DKIM: Is it possible to DKIM-sign the emails myself with my own domain? Is it possible to control the MAIL-FROM header used?
- Shared vs. Dedicated IPs: Will they let you send high-volume email through their shared IPs, or do they aggressively push you towards a dedicated IP?
- The User Interface: For any SMTP service, the SMTP relay system is the most important consideration, but it’s nice when the web UI for reporting and lookups is well designed. Is it slick and modern, or does it look like it was built in the 90s? Most importantly, does it provide three key features: a) the ability to search by recipient and by Subject line, b) the ability to see the exact SMTP conversation with the recipient, and c) the ability to export your data? Does it do thoughtful things like, when it provides DNS records for you to add, give you a “copy to clipboard” widget so you don’t have to drag your cursor over a super long DKIM public key, hoping you get every necessary character but not any extra ones?
- Shared IP reputation: What’s the reputation of their shared IP pool?
- Support competency: Is their front line support personnel competent? Sometimes I find that people performing technical support for email service providers are grossly incompetent, answering basic questions about how email works, entirely wrong. An example awaits you below.
- GMass fit: Finally, we’ll conclude whether the SMTP service is suitable for use with GMass.
First, what is a “pass-through SMTP service?”
Only an SMTP geek would care about this, and if you Google that term, you won’t find anything because I invented it just now. When you relay an email, and your “from” address is [email protected], and then the SMTP service relays the email to the @gmail.com server, does the MAIL-FROM remain “[email protected]”, or does it alter the MAIL-FROM to be “bounce friendly,” so that it becomes [email protected]? A pass-through SMTP server sets the MAIL-FROM to exactly what the “from” address is when you relayed the email. A non-pass-through SMTP server alters it, based on its desire to control bounces for you, or based on your own customization of the service inside the settings.
Why do these things matter?
Depending on what you’re using an STMP service for, the attributes that matter to YOU might differ from the attributes that matter to me. For example, if all you care about is getting your website to send a confirmation email after someone fills out a form, you probably don’t care about the out-of-the-box DKIM setup or whether you’re initially throttled below your paid plan’s sending limits (unless you’re getting thousands of form submissions/day — then you might care). But if you’re like me, and you’re using an SMTP relay to service thousands of users, some of whom are sending broadcast email and some of whom are sending transactional email, and you want the process of onboarding the users onto the SMTP relay as seamless as possible, then factors like these matter, along with the process (or hopefully lack thereof) of verifying a new “from” address.
What do I look for in the ideal SMTP service?
Sadly, no SMTP service, not even the 600-pound gorilla known as SendGrid, meets all of my strict criteria for perfection. Here they are:
-
- The web UI matches what happens over the SMTP relay instantly. Meaning, if I relay an email through the service, and then go to the service’s Reporting page on the web app, that email should immediately show as having been received by the service, even if hasn’t been delivered yet. Even SendGrid sometimes has a one minute+ delay.
- The ability to sign up and send “from” any address, without verification, and by using the provider’s own domain for the MAIL-FROM and DKIM signing.
- The ability to send high volume immediately, knowing that an AI-based algorithm is watching and can shut off spammers.
- The web UI should provide access to the SMTP log for each delivered email.
- The ability to test a platform without putting in a credit card.
- Letting you send “from” a gmail.com address.
- The ability to set whether the service should be pass-through or not.
- The ability to set one domain as a default domain, where all mail, regardless of the “from” address uses a MAIL-FROM and DKIM signature based on that one domain.
The big list of SMTP services
We’ll review these SMTP services suitable for relaying broadcast emails:
- SMTP.com
- Sendinblue
- SendGrid
- Mailjet
- Mailgun
- SMTP2Go
- SocketLabs
- Amazon SES
- Mandrill
For each, I will subscribe to the lowest priced option, so that I’m not a “free” user subject to free account restrictions, but I’m hoping that there won’t be any sending limitations imposed on me other than my paid plan’s limits. I do think that letting people test a platform for basic functionality and UI aesthetics is important though for free, and without having to tender a credit card.
Did your favorite SMTP service not make the list? Comment below to let me know, and I’ll get around to reviewing it eventually.
A Summary of Capabilities
Service |
Send from @gmail.com |
Out-of-the-box DKIM/SPF compliance |
Can send right away without human verification |
SMTP.com |
YES |
N/A |
NO |
Sendinblue |
YES |
YES |
YES |
SendGrid |
YES |
YES |
YES |
Mailgun |
YES |
NO |
YES |
Mailjet |
YES |
YES |
YES |
SMTP2Go |
YES |
NO |
YES |
SocketLabs |
NO |
YES |
NO |
Amazon SES |
YES |
YES |
NO |
Mandrill |
NO |
NO |
YES |
SMTP.com – Built like Fort Knox and still it barely works
(back to top)
As an SaaS platform developer, I’m partial to platforms that let me sign up and start using the service right away, without any manual review.
SMTP.com is NOT like that.
Being able to relay even a test email through SMTP.com required a multi-day approval process that started with a manual review of my account and ended with the rep asking me to place a new page on my website to prove that I owned my website’s domain. Gosh! Only then was I given credentials to SMTP.com’s server.
Here’s the first email asking for more information.
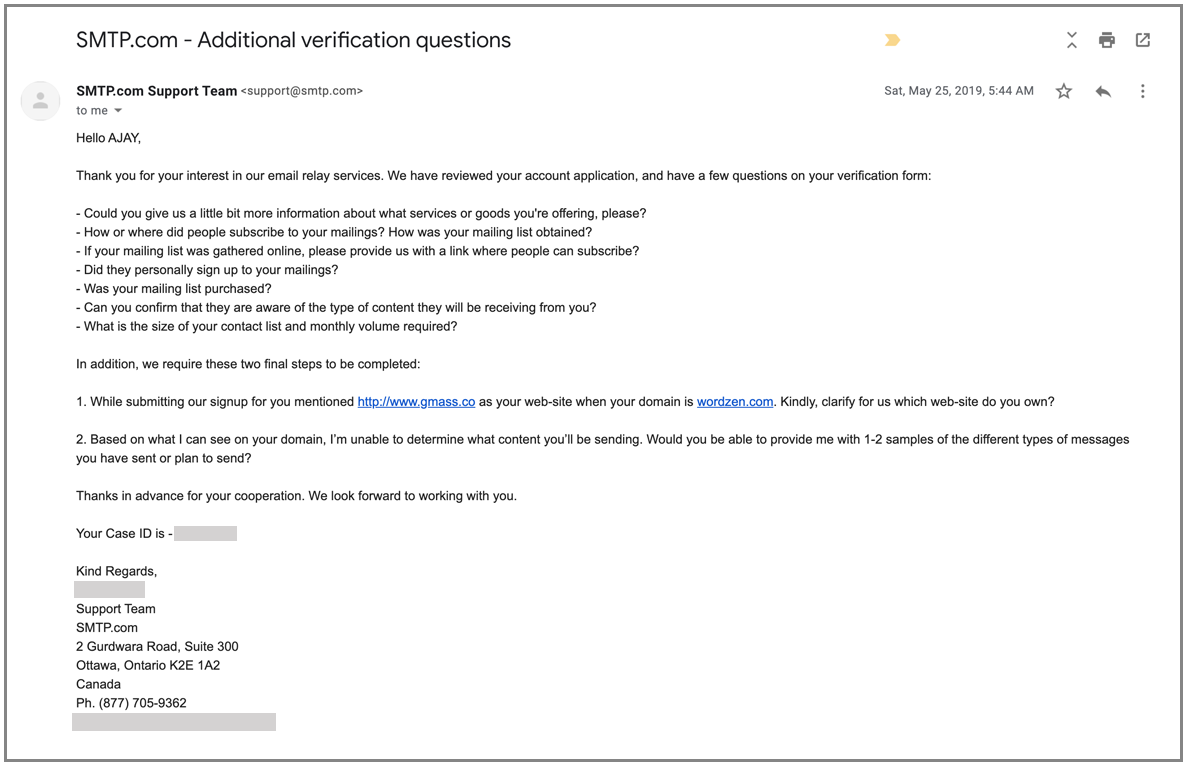
After answering the questions, here’s the request to place a page on my website. This goes down as the most work I’ve ever had to do to verify myself for an SMTP service. I mean, they could have just asked to send an email to some-user@my-domain, like SSL certificate issuers do to confirm ownership of a domain.
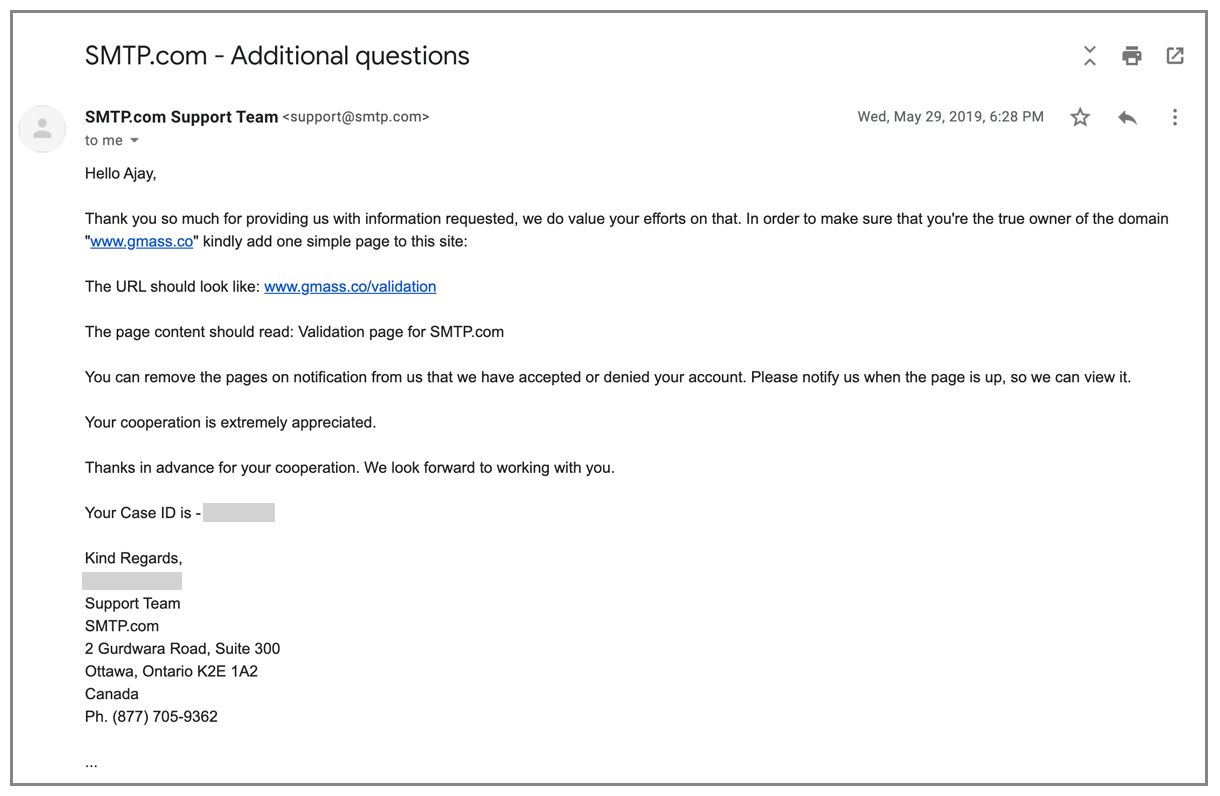
This is also one of the few services that requires a credit card to try.
After jumping through all these hoops, one would hope for a seamless user experience, but that wasn’t to be. Most of my test emails never arrived.
Here is proof that I’ve sent an email:
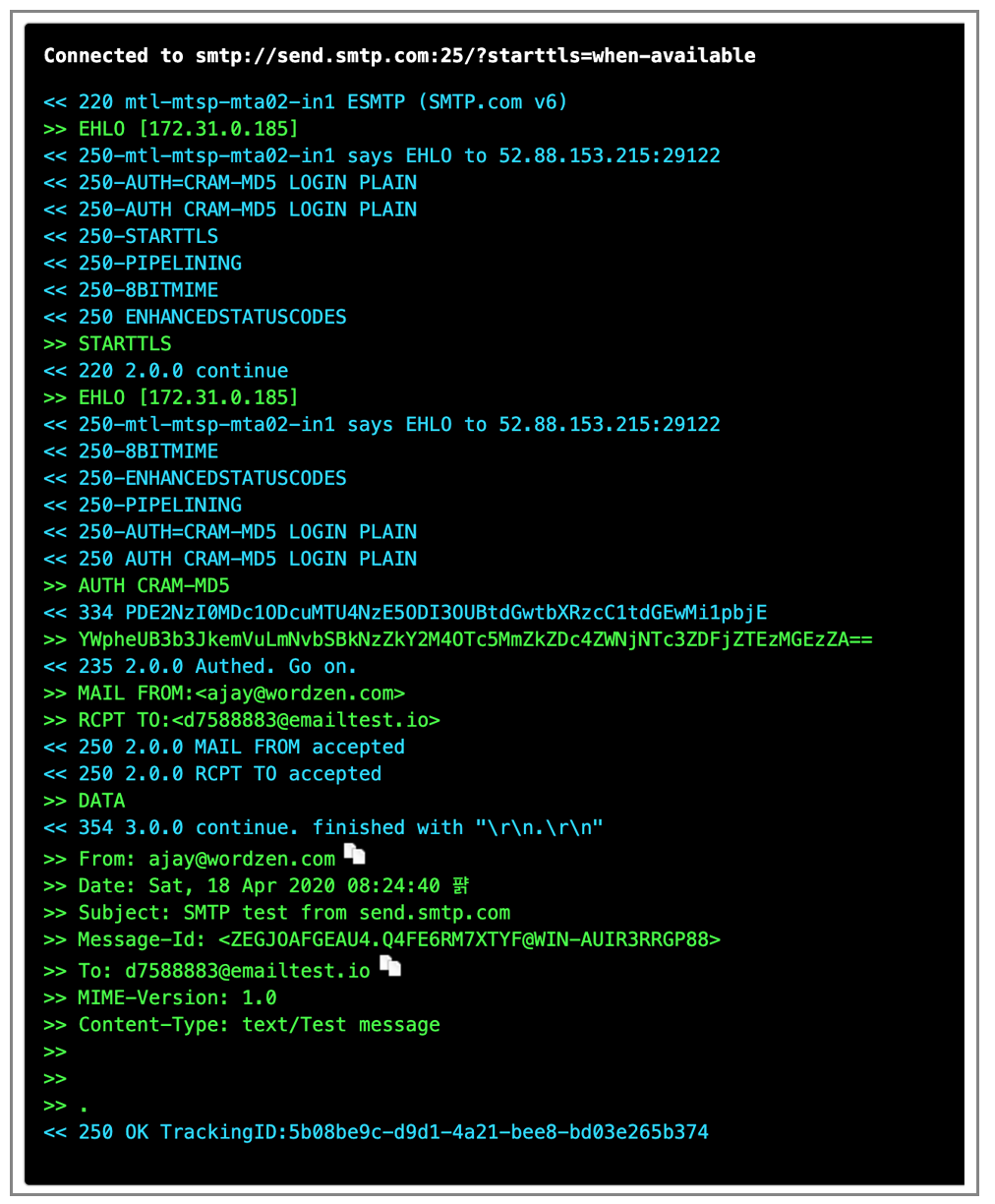
Not only did the email not arrive to the email box, it doesn’t even show up in their reporting:
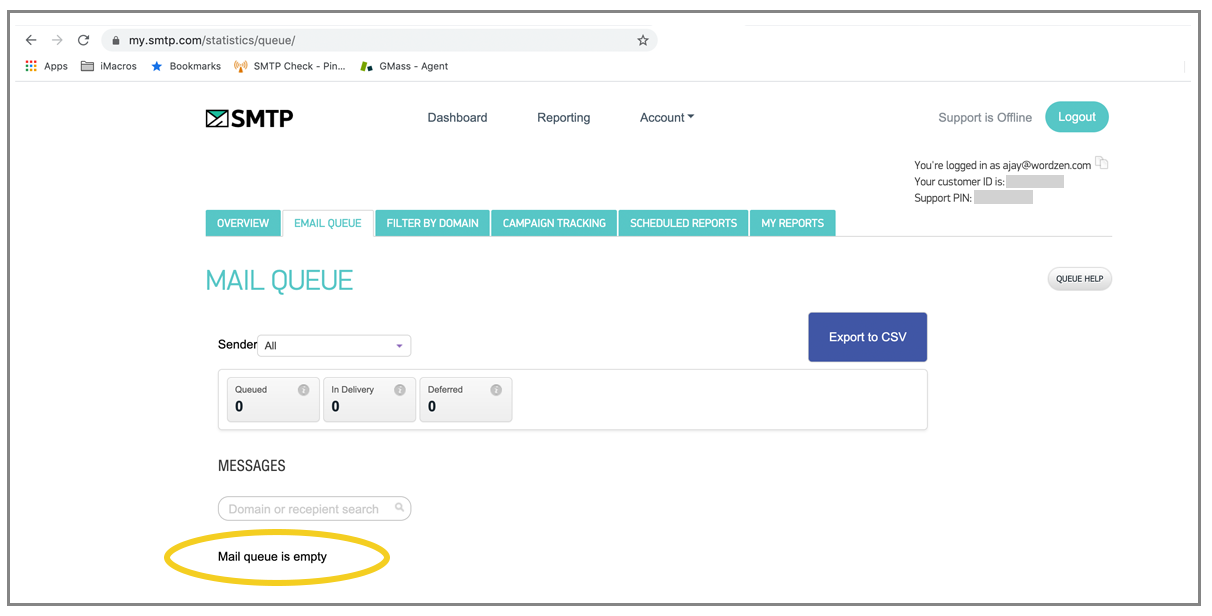
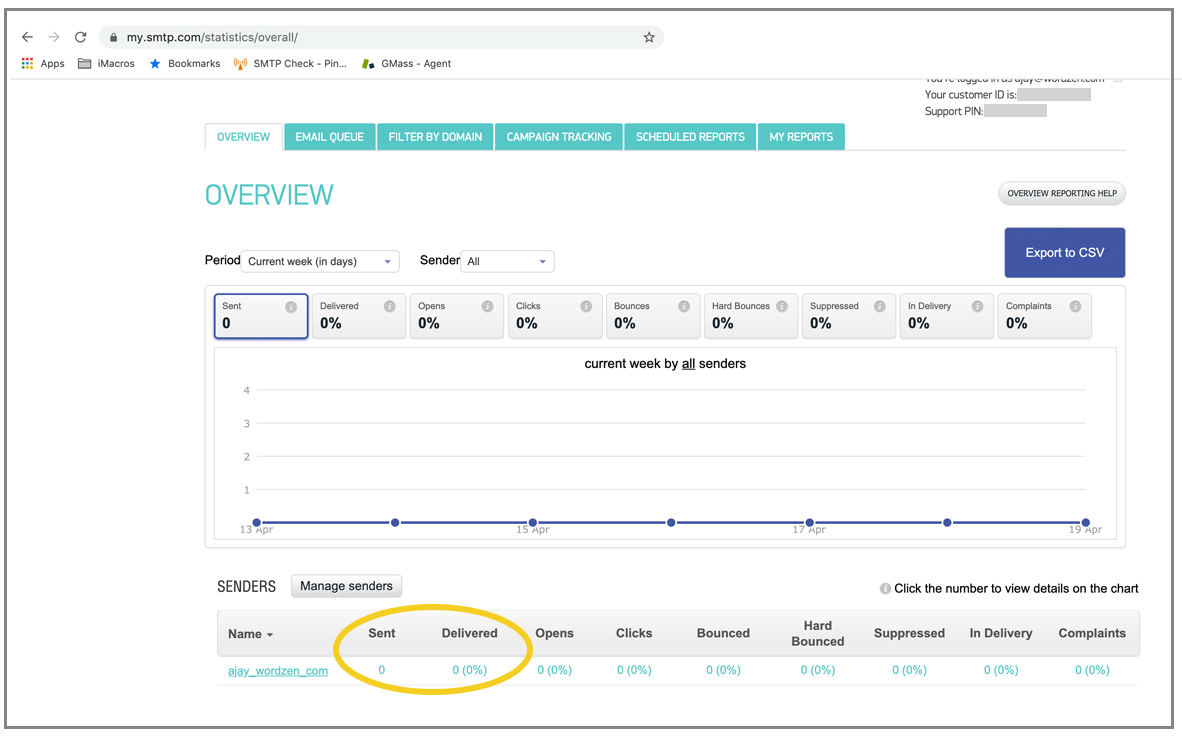
We are at a dead end.
Oh wait, one of my two test emails arrived. The other is in the mail queue:
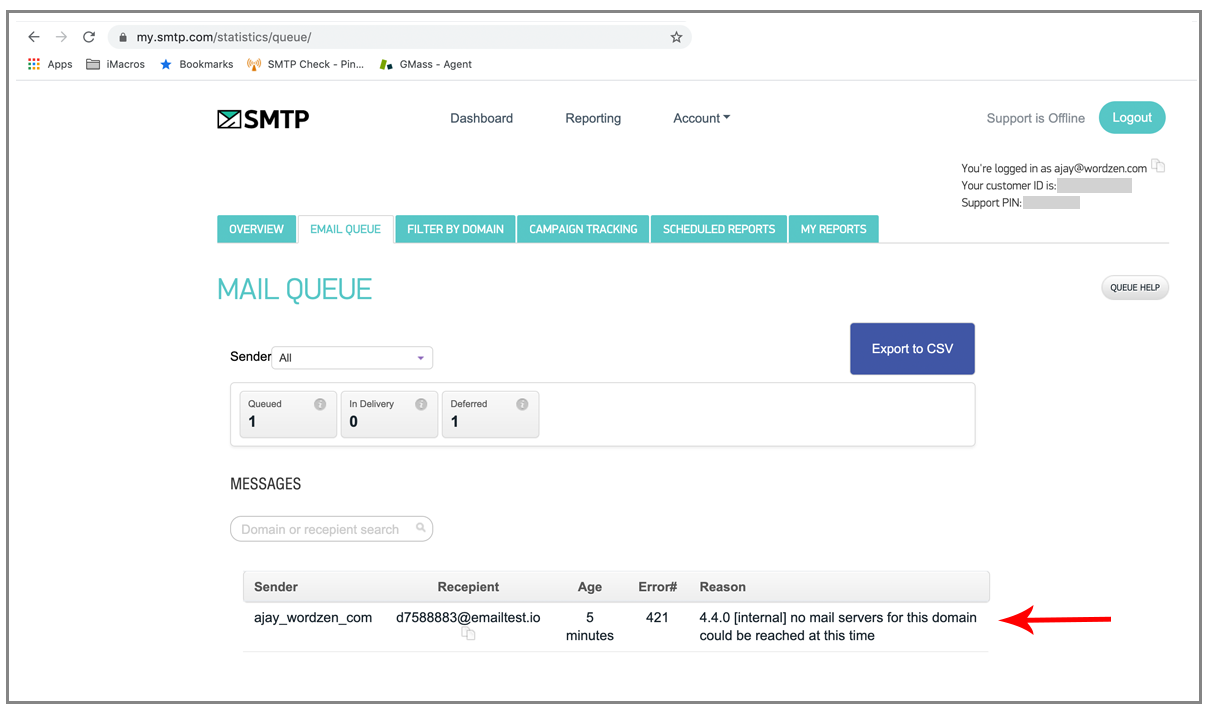
It’s claim that the mail server for emailtest.io couldn’t be reached simply isn’t possible, because that email server is receiving a constant flow of emails from around the world, 24 hours a day. I suspect the bigger issue at hand is that it does it not know how to look up an MX record for a domain that’s not already in its cache.
SMTP.com requires a manual account review before you can send anything or even access your portal. Their verification email landed in spam. And you have to enter a credit card upon signup and choose a paid plan, because there’s no free trial.
The missing email shows as “queued for delivery,” but several minutes later, the relay accepts it.
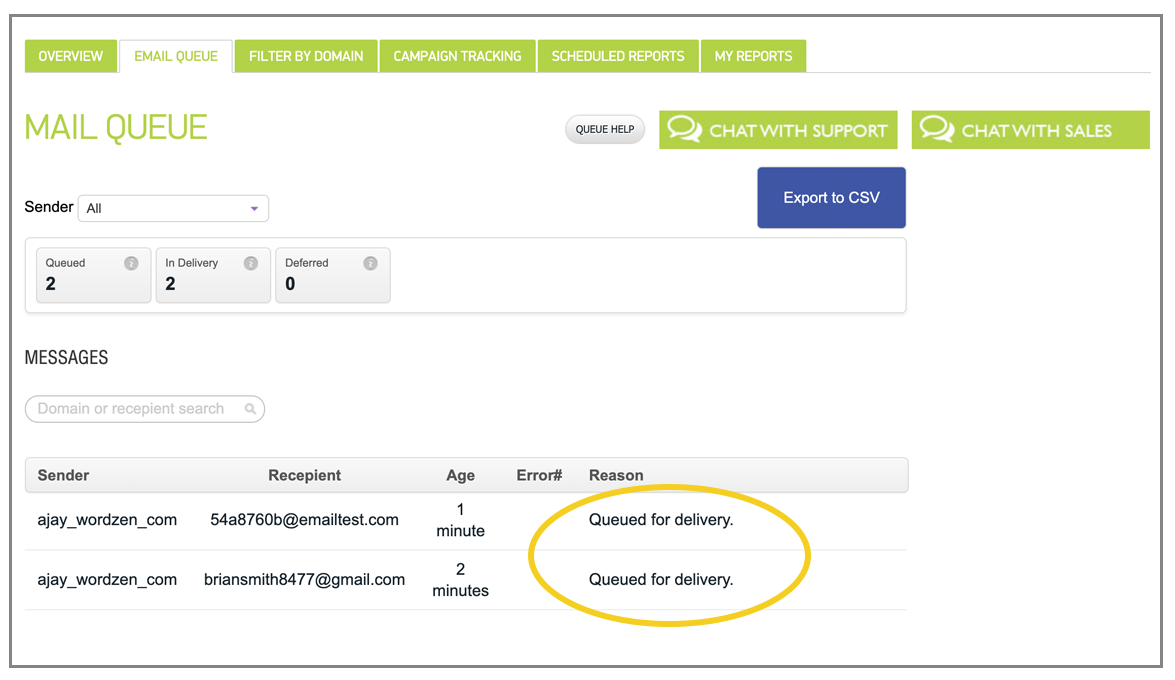
My own SMTP tester shows the email is properly delivered to SMTP.com:
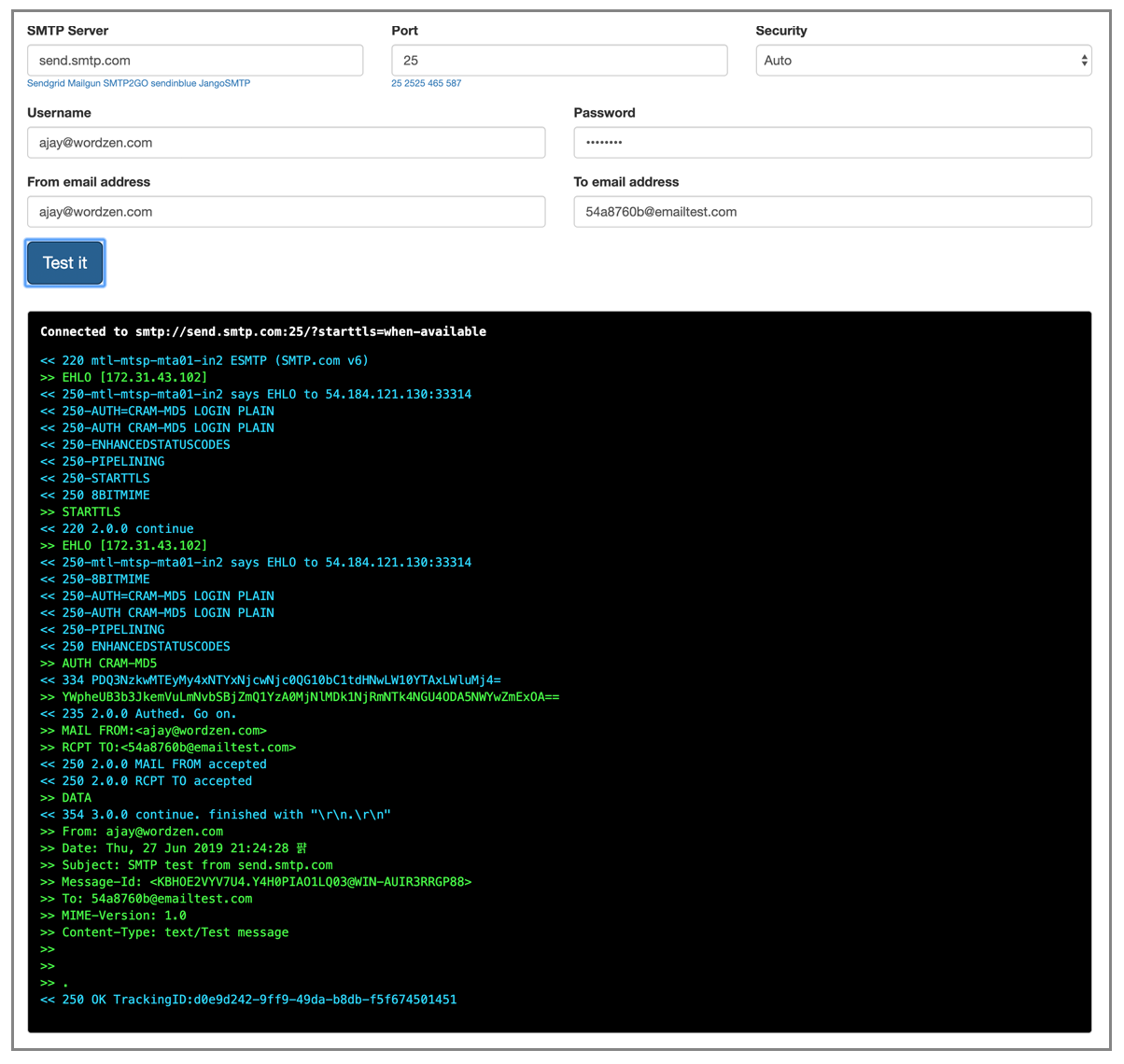
Three minutes in, and still nothing.
Furthermore, I expected that if I clicked on a number in its User Interface, I’d get to see the detail behind that number. Sadly, that isn’t the case. Clicking on “Delivered” here would be useful to see exactly which emails have been delivered:

Another example: If I click the one under Hard Bounced, I’d like to know which one address that is, and it doesn’t tell me.

SMTP.com’s unique model allows you to create multiple “senders,” and each one comes with its own SMTP username/password. Each “sender” is NOT restricted, which means each one can send from more that that one “from” address, which is nice; but ultimately, it doesn’t matter because nothing is being delivered.
Even after a few minutes, it is still not delivering:
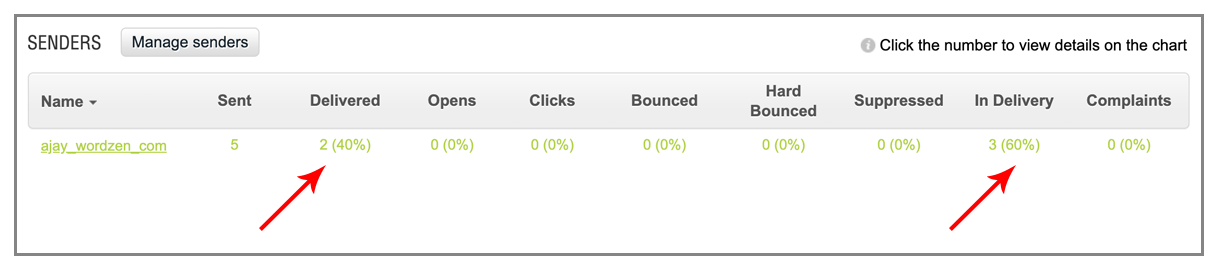
And 19 hours later, my test email is still in the queue:
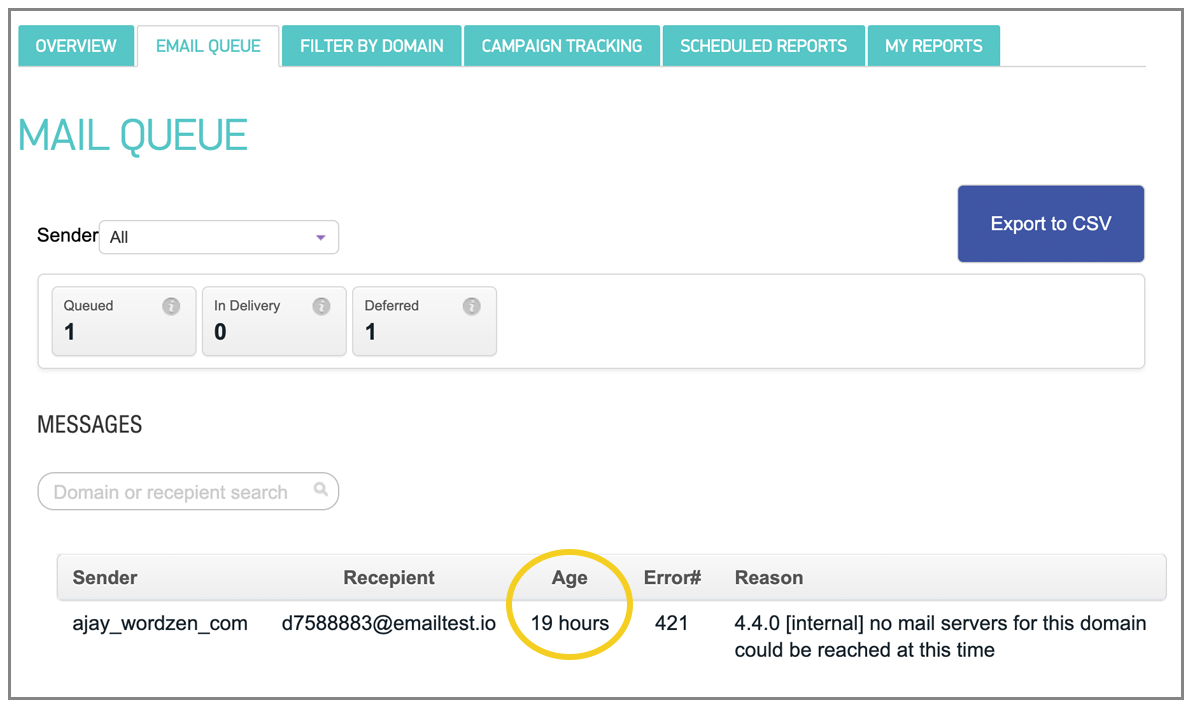
Also, I see this, but it doesn’t tell me anything. Do I really have to get in touch with support to solve this?

The most frustrating thing about testing SMTP.com is that there’s a significant lag between when you relay messages and when the messages appear anywhere in the UI, whether as “queued” or as “delivered.” In my test cases, messages were queued for no apparent reason.
By default, SMTP.com adds an open tracking pixel and modifies URLs to be click-tracked, but unlike Sendinblue, it can be turned off:
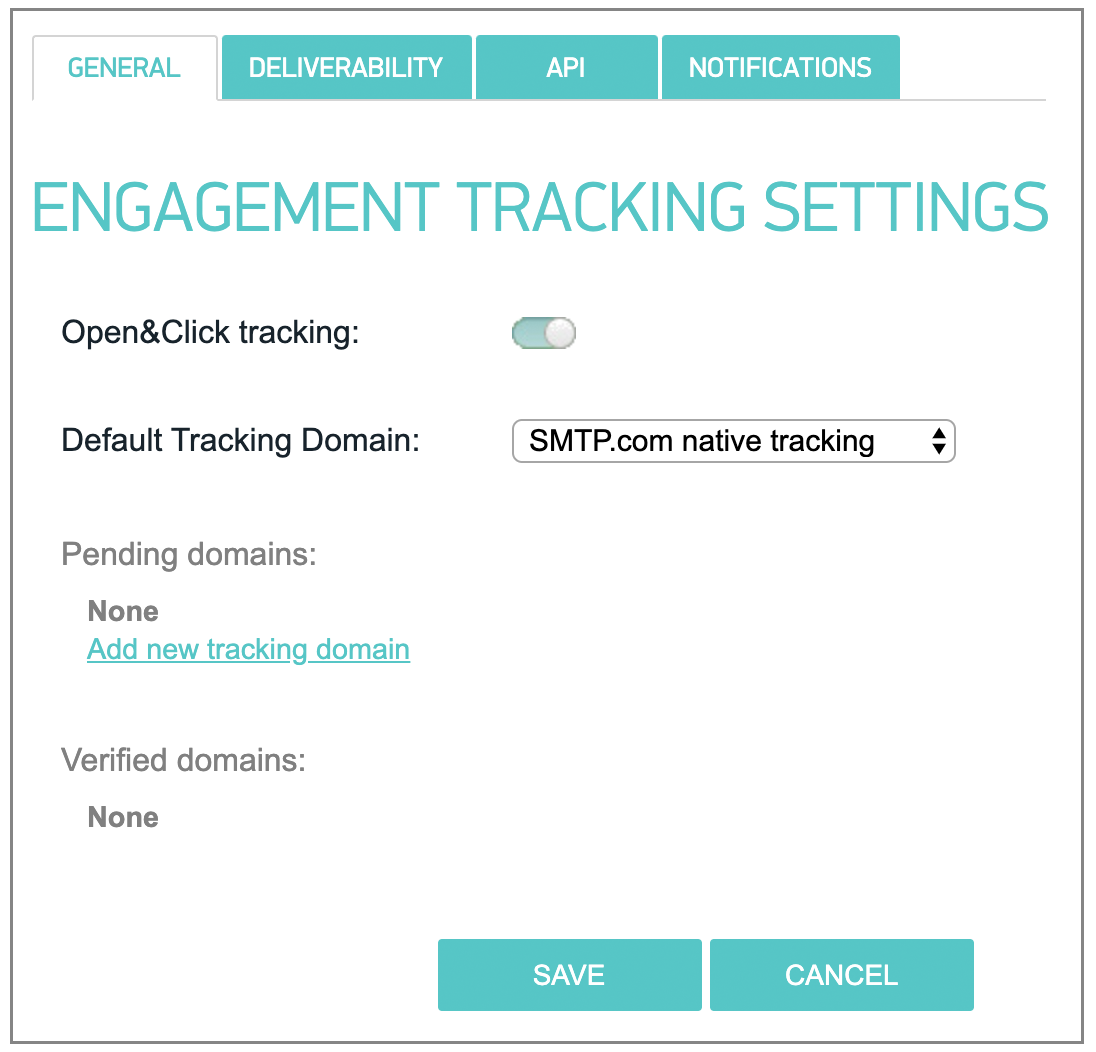
Interesting factoid: SMTP.com has an interesting history. Not only does it have the ultimate domain name for an SMTP service, but also it used to be a small public company trading on the OTC market. I remember following its stock price back in the day, but now it’s no longer public.
The skinny on SMTP.com
UI: Old, clunky, and can’t click on a number to see its detail. The UI is updated long after an email is relayed to the service.
Email delivery: Most of my emails went missing.
Signup: The most painful signup and verification process of any SMTP service.
Grade: D
Sendinblue – an easy, efficient solution with one major flaw
(back to top)
I was impressed with the ease of sign-up! With sendinblue, I could send right away, my test emails made it to the INBOX, and SPF passes out-of-the-box because it uses its own domain in the MAIL-FROM area and signs mail using its own domain, sendinblue.com:
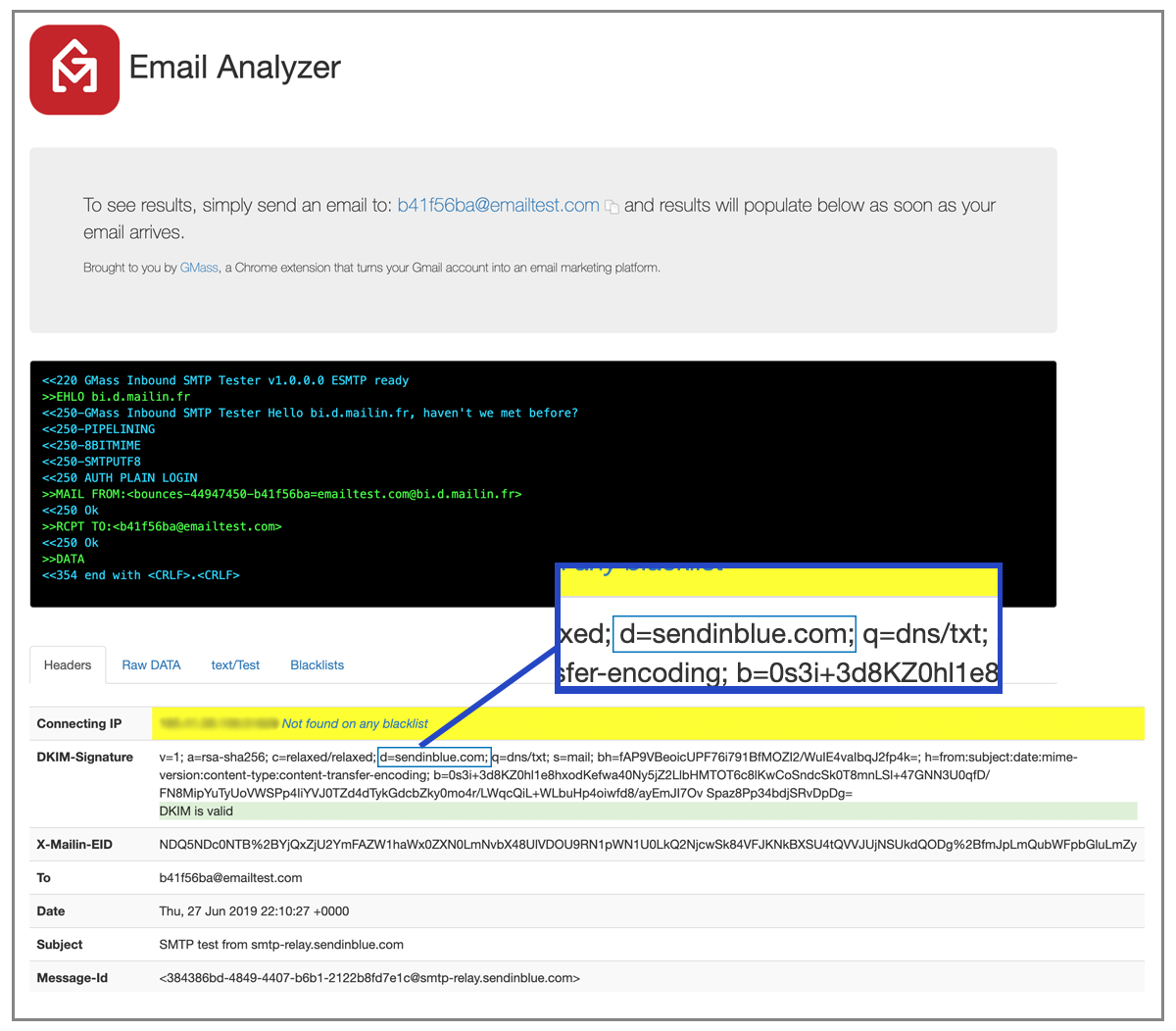
Sendinblue is not a pass-through SMTP service like SMTP2Go is.
Sendinblue allows you to create an account and start sending without any manual review.
Additionally, out of the box, it uses its own domain for SPF and DKIM verification, making it easy to start sending right away with minimal technical setup. What’s the one major flaw, however? Sendinblue forces an open-tracking pixel into all emails relayed through the SMTP service, and open-tracking can’t be turned off unless you meet some stringent sender requirements. This poses a problem if you’re using a solution like GMass or any other desktop email marketing app which requires an SMTP service to be used. GMass and every other major email marketing app inserts its own open tracking pixel to provide campaign-level reporting, so if the SMTP service also inserts its own open-tracking pixel, the email will be double-tracked. This doesn’t pose any technical issues on the surface, but a savvy email recipient might see this as nefarious, and open-tracking pixels, sometimes referred to as “web bugs,” can be a trigger for spam filters. Most importantly, though, it’s just silly to force that pixel and prevent the user from turning it off.
What the receiver sees:
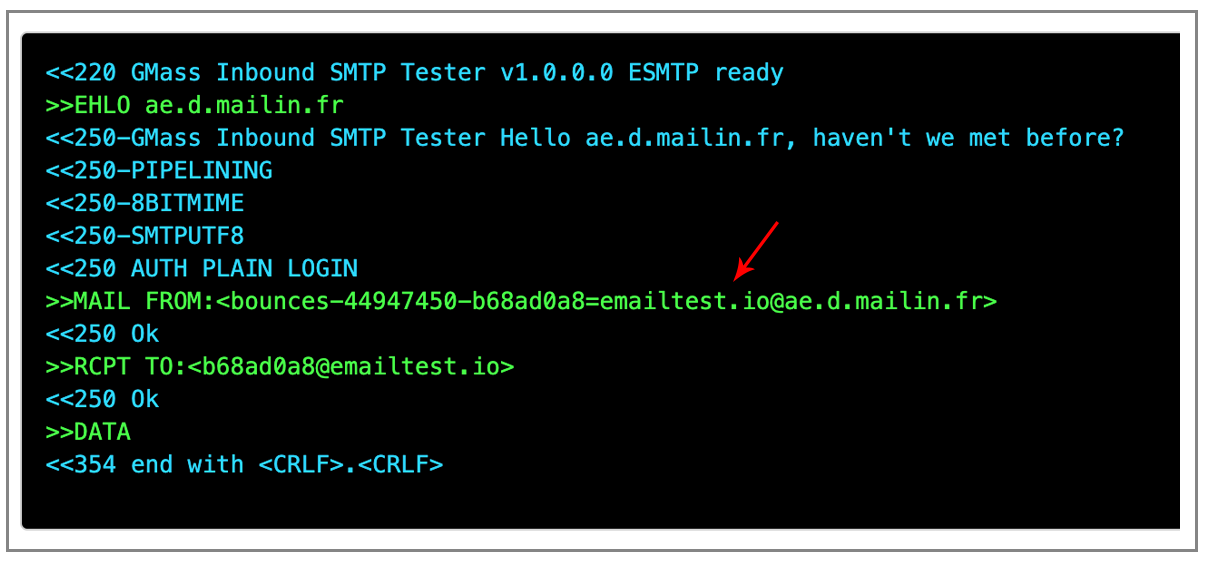
Note that the MAIL-FROM that sendinblue uses is [email protected].
That makes this service automatically SPF compliant.
Additionally, they sign the emails with their own domain, sendinblue.com, and this is what the DKIM signature looks like:
DKIM-Signature: v=1; a=rsa-sha256; c=relaxed/relaxed; d=sendinblue.com; q=dns/txt; s=mail; bh=fnXDWgomUIbb+pTCU+UYvgSEaOR9AguPfISJbxLOyjQ=; h=from:subject:date:mime-version:content-type:content-transfer-encoding:x-csa-complaints; b=daAN/k9814CLN57y2vaVO0e+5kbmiQj+PevO2hxAz9W6Y/1li3C6oiH3X3BY+/Z1mvUxvrHzyUcv TZBqQ3oU3Q4pPrAB15lMGyblmWiokMTP87vaQfKlnp4epSQDnO4/8DBmVHFdj5revifsxiGbC98i vScv7Ezf9e2CGNj610Y=
This is great (and unique) because it doesn’t require you to set up your own domain, and it still lets you send “from” any domain. The fatal flaw? They force that open tracking pixel:
<img width="1" height="1" src="http://bdahhda.r.af.d.sendibt2.com/tr/op/xUUXgMOgBixJy1E8_ZzQniCsvk8urceI0ErIEWuQMaicc4uwCQBaTG3tNrvq2VithC3TlBnEPeFotR7gyBW8Bl8f0k84SDT6sNLWScoUUrP-MU7O3WhduoUlQryvTz88t4yB1bf0z598wQ" alt="" />
I inquired with Sendinblue’s tech support, and was told this:
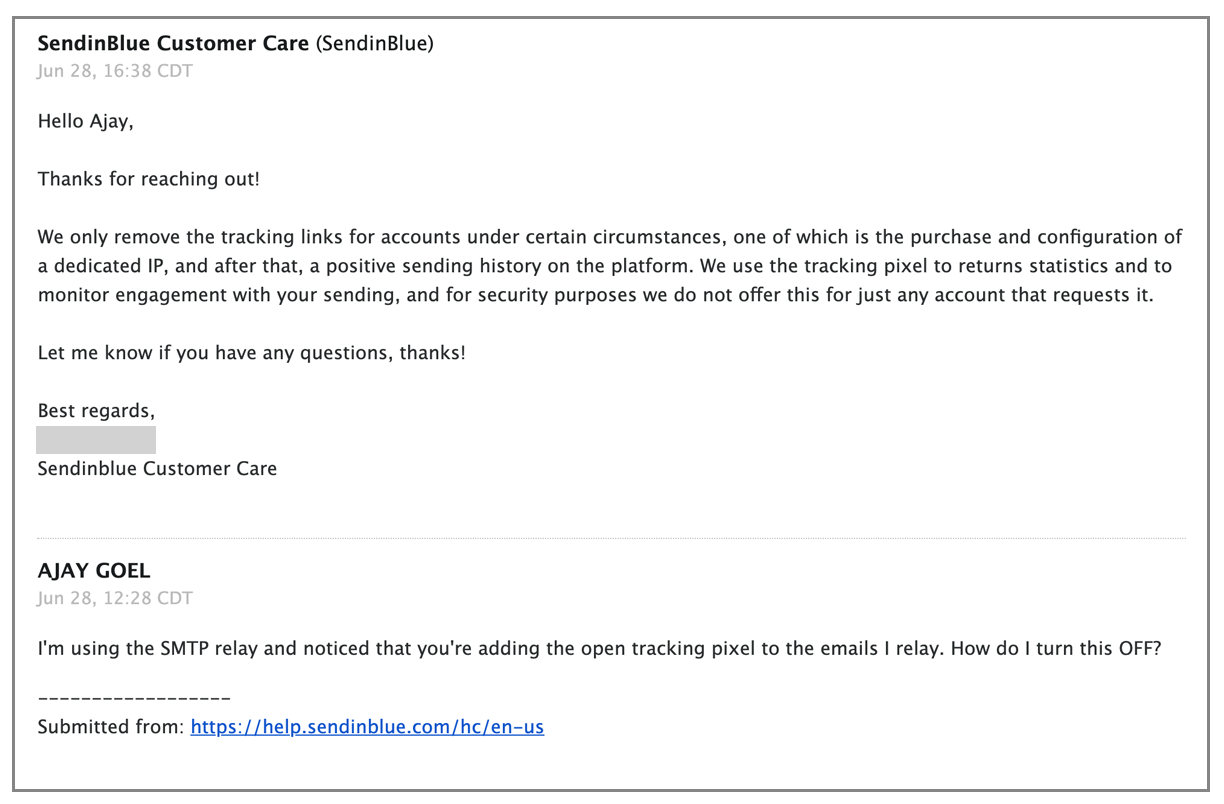
They also have a strange sending quota of measuring your hourly emails. I’ve had my account for about a year, and over its lifetime, I’ve sent 3,164 emails with a 41% open rate. Still, though, my quota is set to just 78 emails/hour. In fact, a few days ago I went over this quota and received this notification:
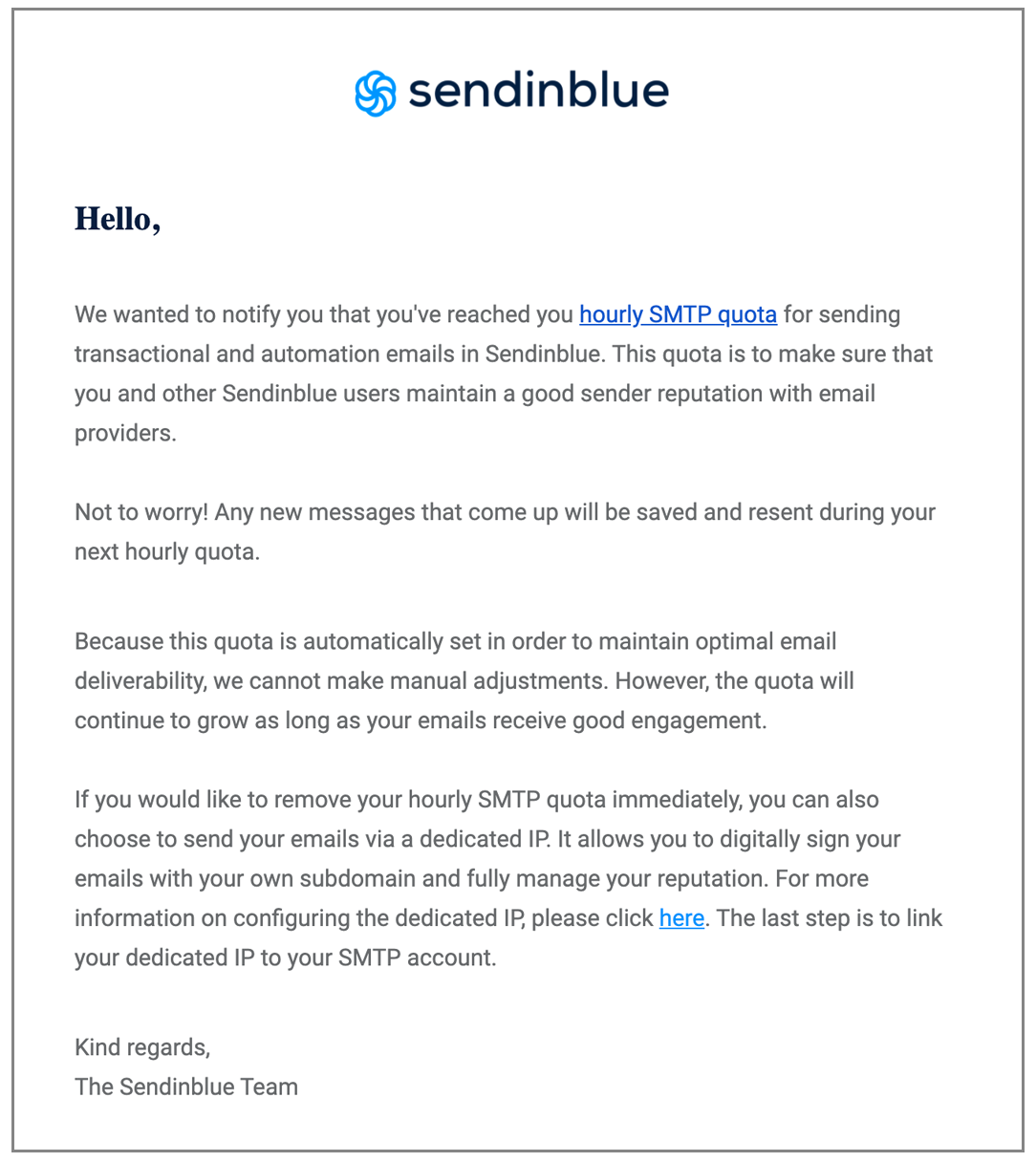
This means that they aren’t employing artificially intelligent systems to monitor their mail flow. By setting a hard quota, it’s easier on them, but it’s harder on the user. So, what happens if I try to send 1,000 emails through the service? Let’s find out.
The User Interface
Strange instructions for SPF setup:
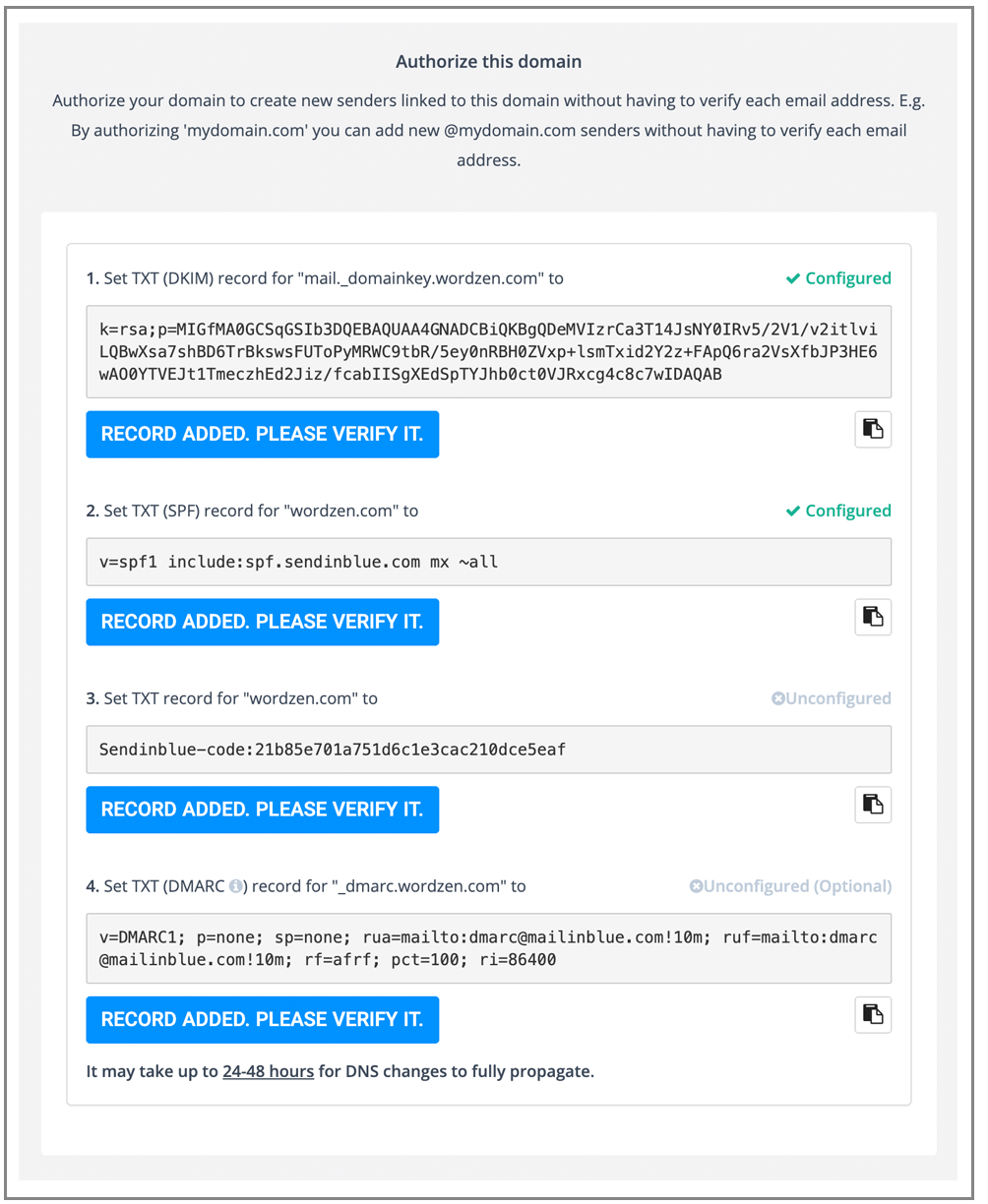
They don’t bother to mention that this will overwrite any existing SPF settings I already have in place, like the default G Suite SPF record.
The UI is a little clunky. For example, even though I verified that I own wordzen.com by modifying the SPF and DKIM records, the option to verify the domain still appears:

Also, when you’re on this screen, and you want your SMTP credentials, you click “Get your SMTP key.”
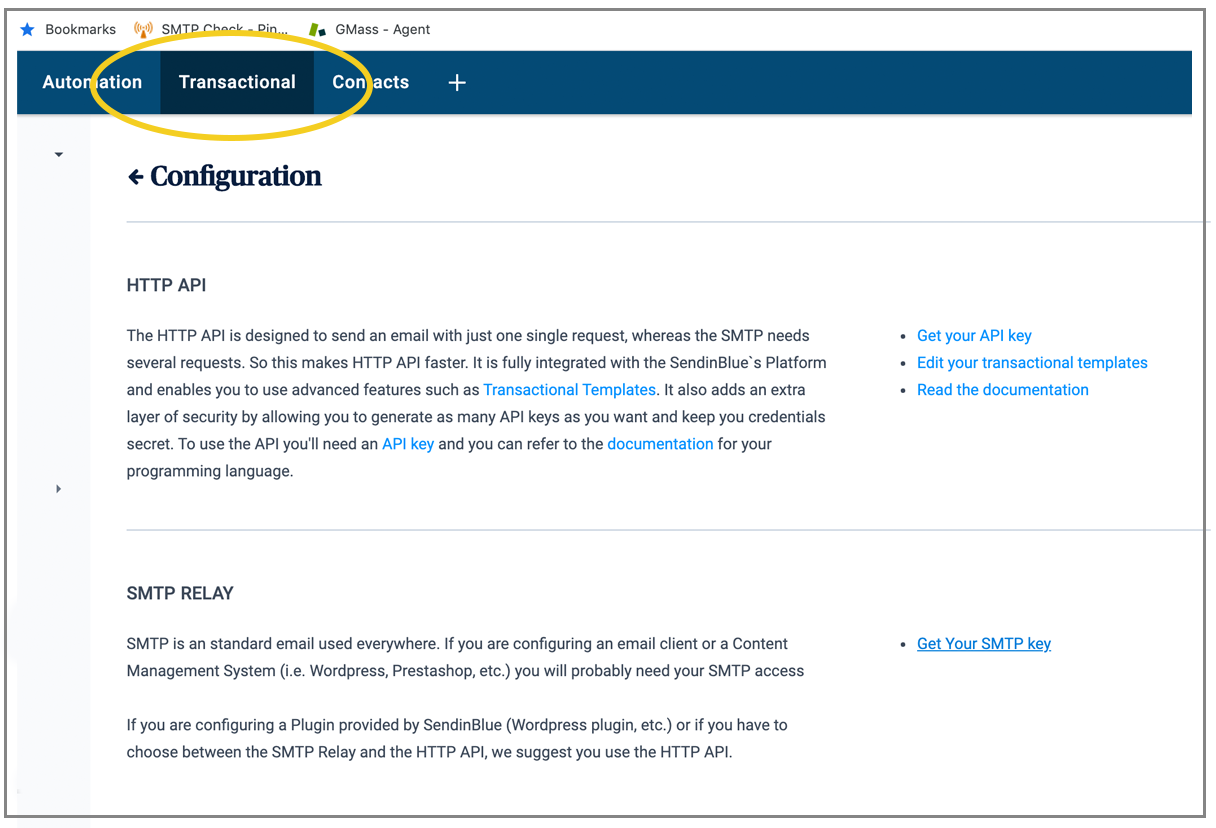
But that takes you to the transactional API page, not the SMTP credential page.
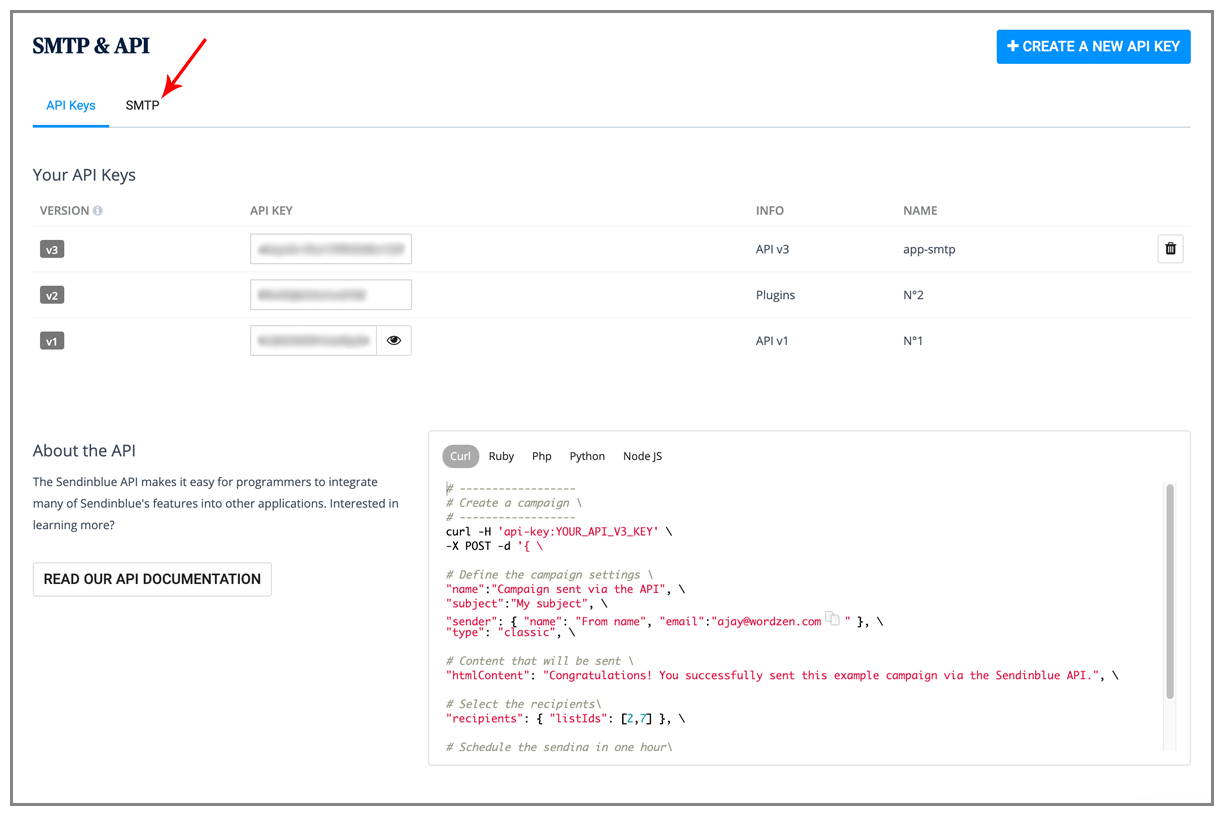
You have to click over to the SMTP tab to get the info you need.
DKIM and SPF
Can I DKIM-sign with my own domain and be in control of the MAIL-FROM header?
With out-of-the-box setup, the From line looks like this:
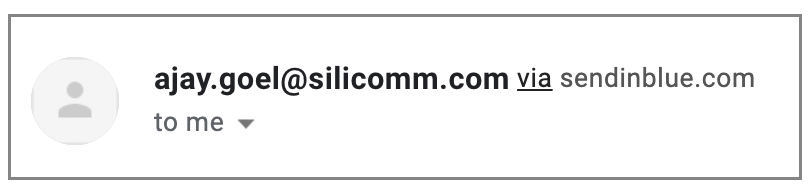
It is sent “via sendinblue.com” since that is the domain used in the DKIM signature.
You can add your domain, but then they have a verification process to make sure you own it:
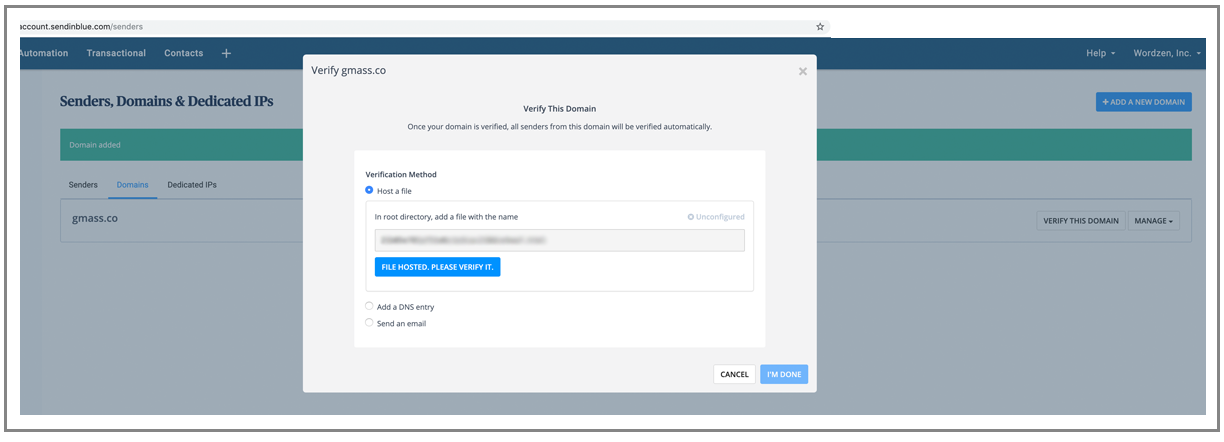
There are a couple flaws with how they verify and use the customers’ domain. First, regardless of what I do, I can’t get the MAIL-FROM to be my domain, wordzen.com. In this case, I’m sending from “[email protected],” and wordzen.com is fully authenticated in their UI:
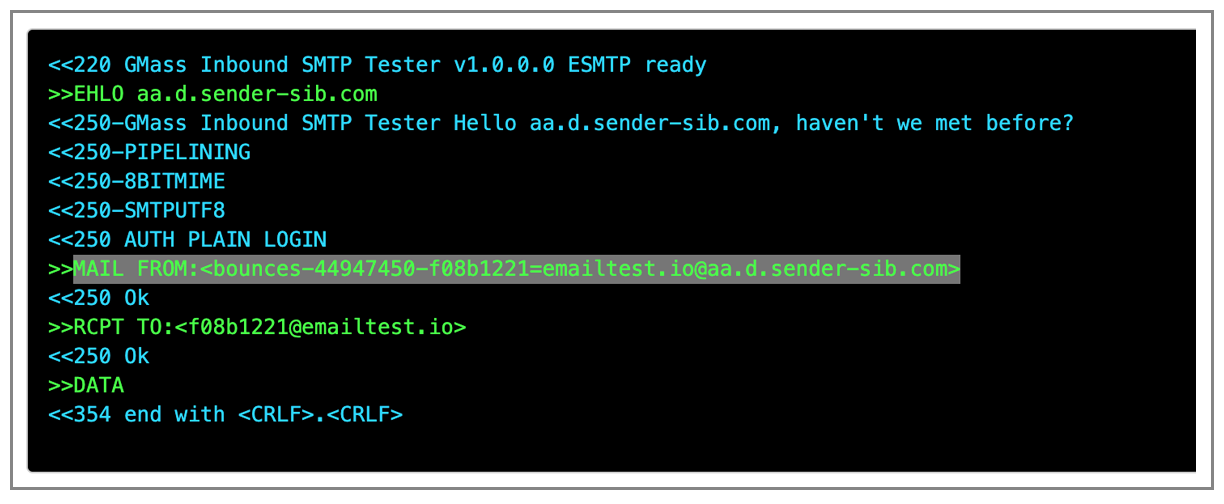
So what’s the point of making me modify my SPF record?
Second, even though I’ve fully verified wordzen.com, only emails sent from [email protected] will have the wordzen.com domain as the signer. I should be able to send from any domain and have wordzen.com be the signer. It tells me as much, though:
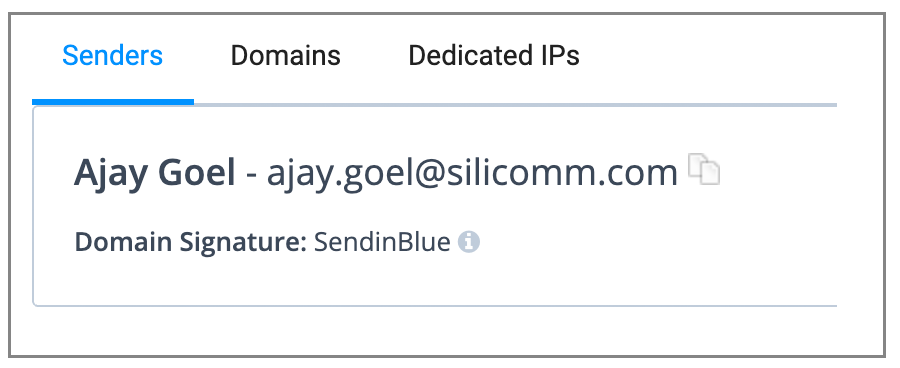
Third, they ask for extra DNS records that aren’t necessary to prove ownership, and they force the DMARC reporting to go straight to them! What if I’m using multiple providers? What if I want to own the process and have reports emailed to me?
UI actions matching the settings in the SMTP relay
Even more concerning, though, is what comes after deleting my domain entirely from their system as an authenticated domain. When I relay an email, it is still signing it from wordzen.com. I even remove the DNS records. Cached for 1800 fine, but still!
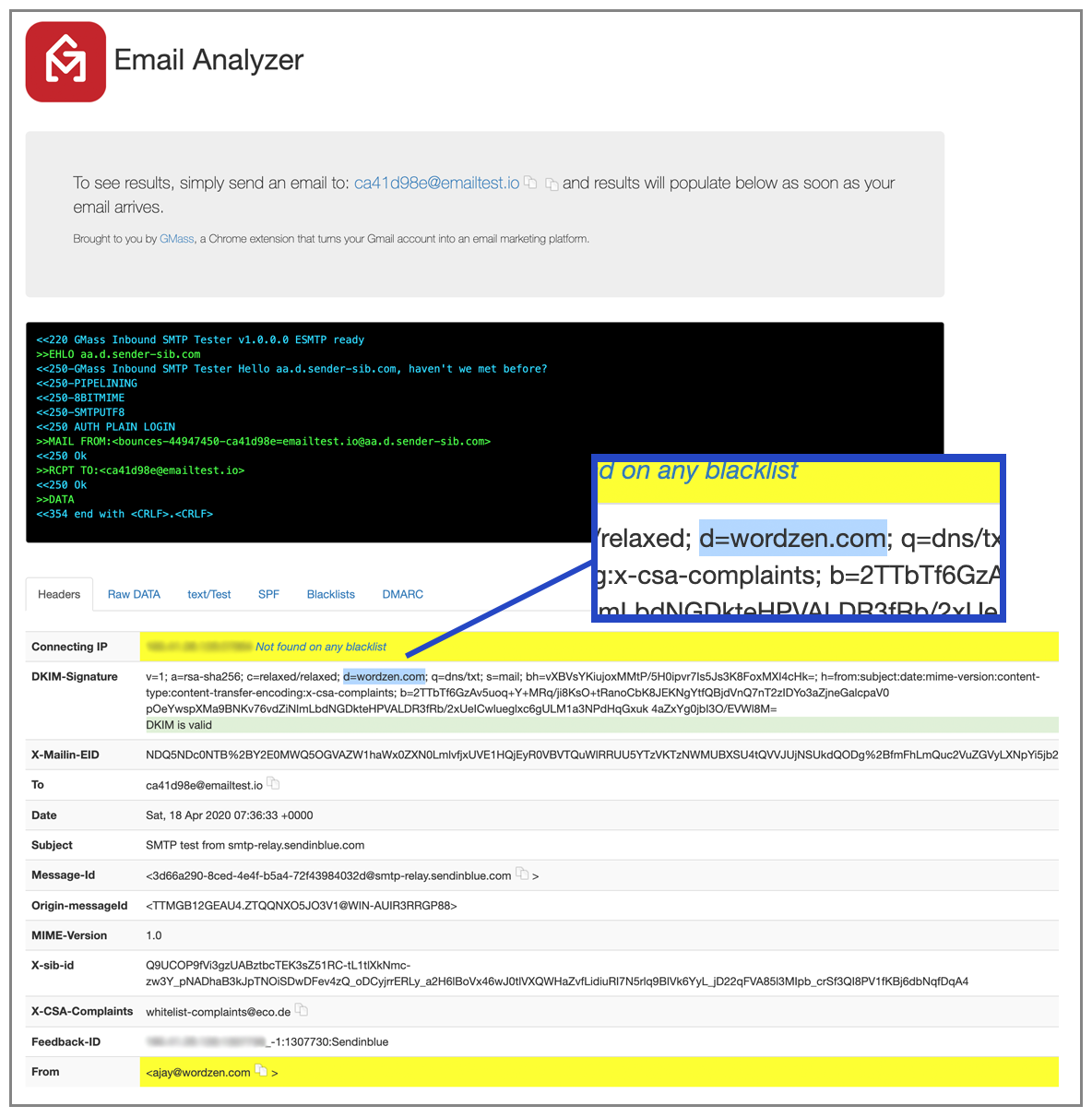
See, look: domain gone!
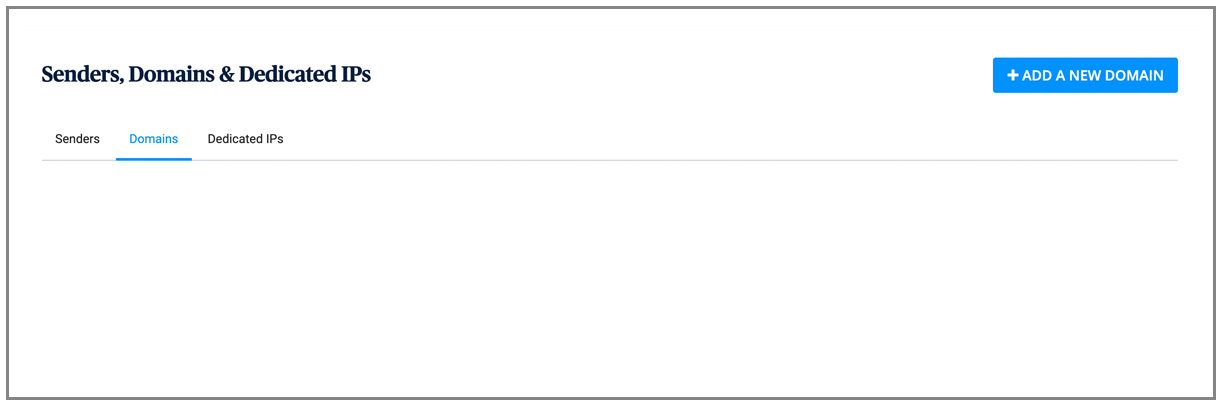
Ten minutes later, it went back to being signed by sendinblue.com:
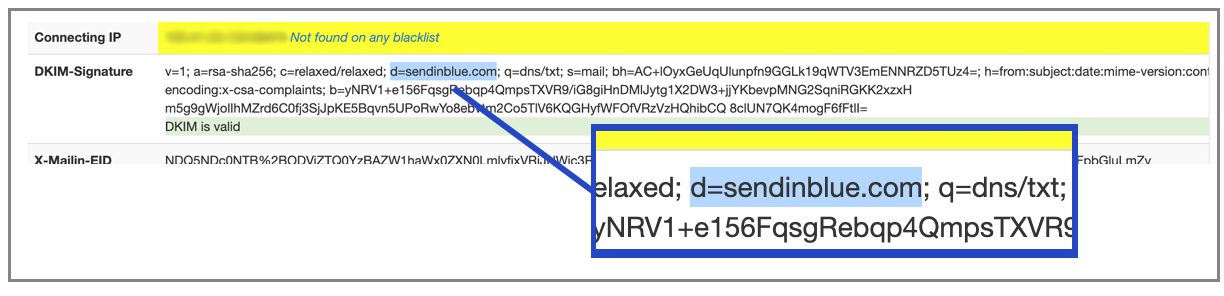
It turns out, it’s just a case of the SMTP service not reflecting changes to the web UI immediately.
Support
Normally I don’t evaluate a provider’s support team, but in the case where a tech completely misunderstands a question or answers it wrong, it’s important to highlight it.
My question was: How do I control MAIL-FROM and set it to my domain?
Support responded, and got the answer completely wrong.
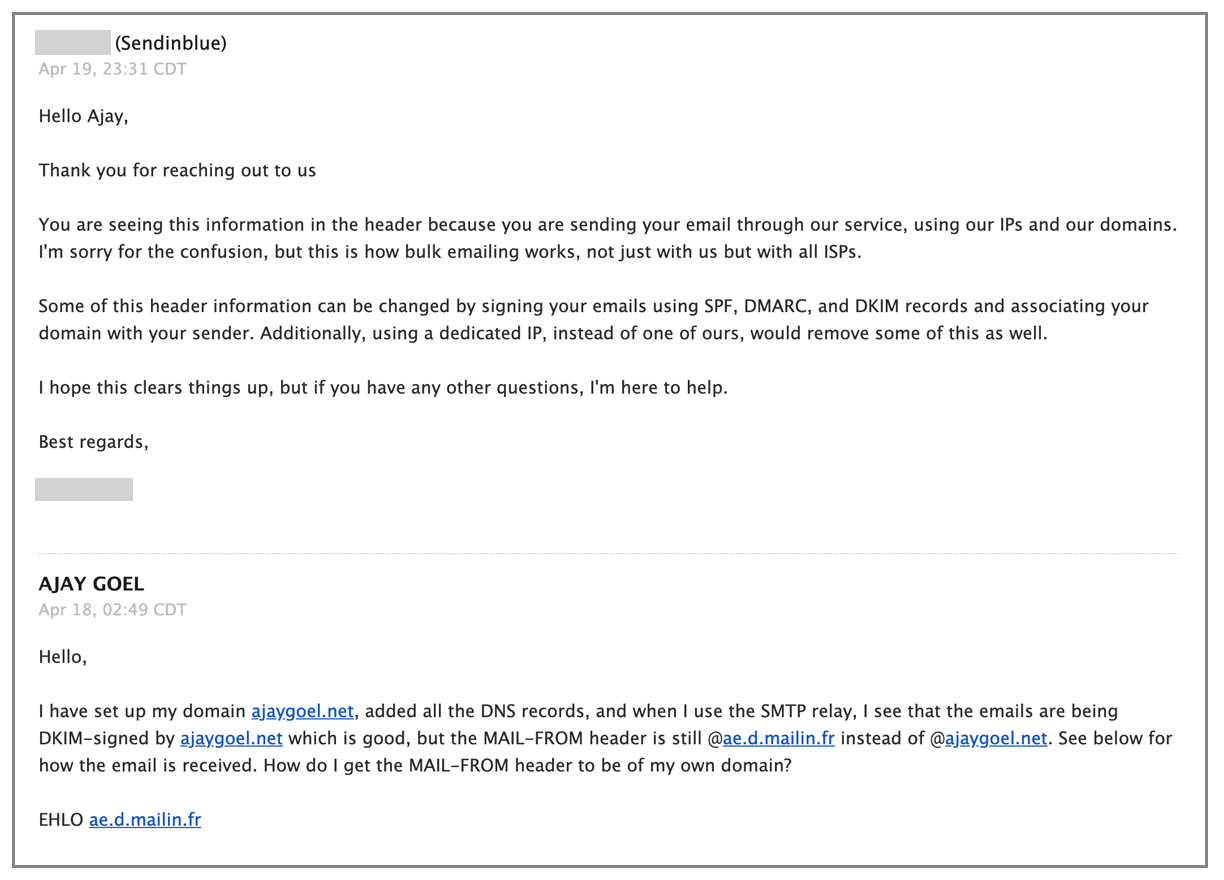
The skinny on Sendinblue:
Sendinblue is a decent service that delivers mail quickly and has an easy out-of-the-box setup. A few flaws exist that prevent me from taking the service seriously:
- They force an open-tracking pixel.
- They don’t let you control the MAIL-FROM line even though they have you set up an SPF record, which is then pointless.
- Their front line support team seems clueless.
- Their throttling of emails is too stringent and doesn’t relax quickly enough, even after proving you’re a reputable sender.
Grade: C+
For more, read my non-technical Sendinblue review.
SocketLabs
(back to top)
The first thing you’ll notice when logging into SocketLabs is that their UI is overloaded:
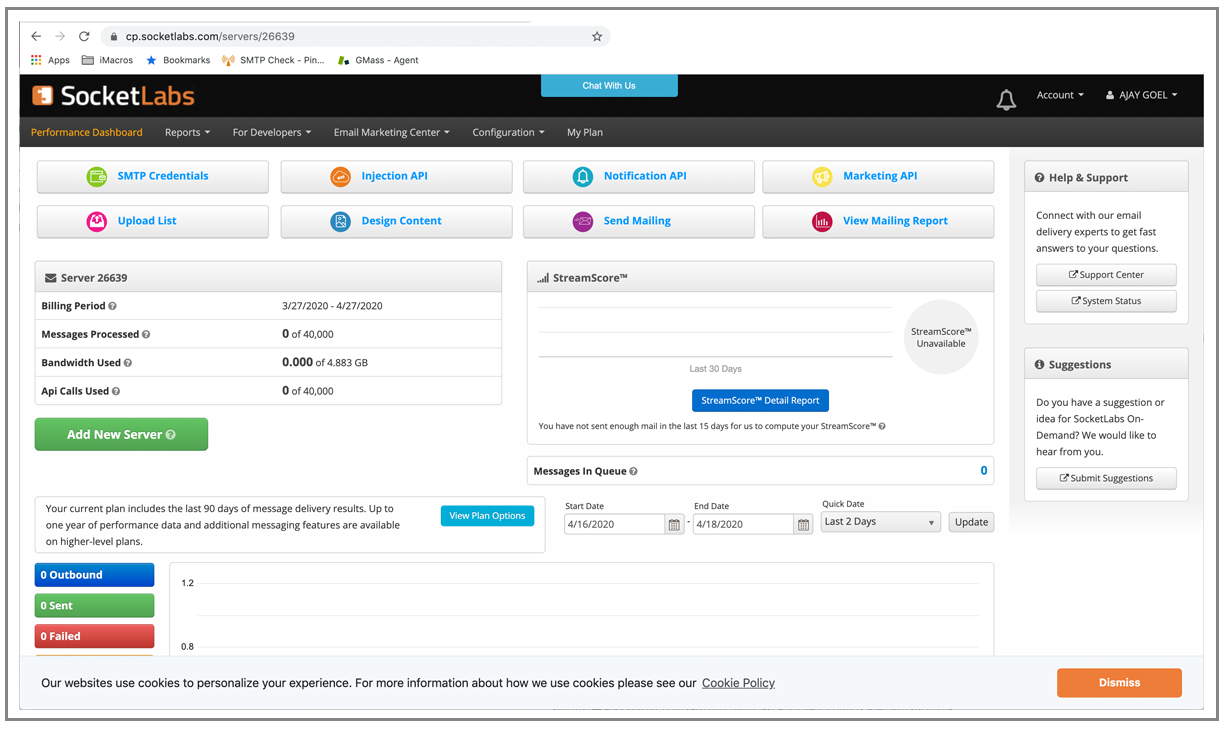
The second thing you’ll notice is that to start sending, you need to launch a “server” and it takes 30 minutes to provision that server:
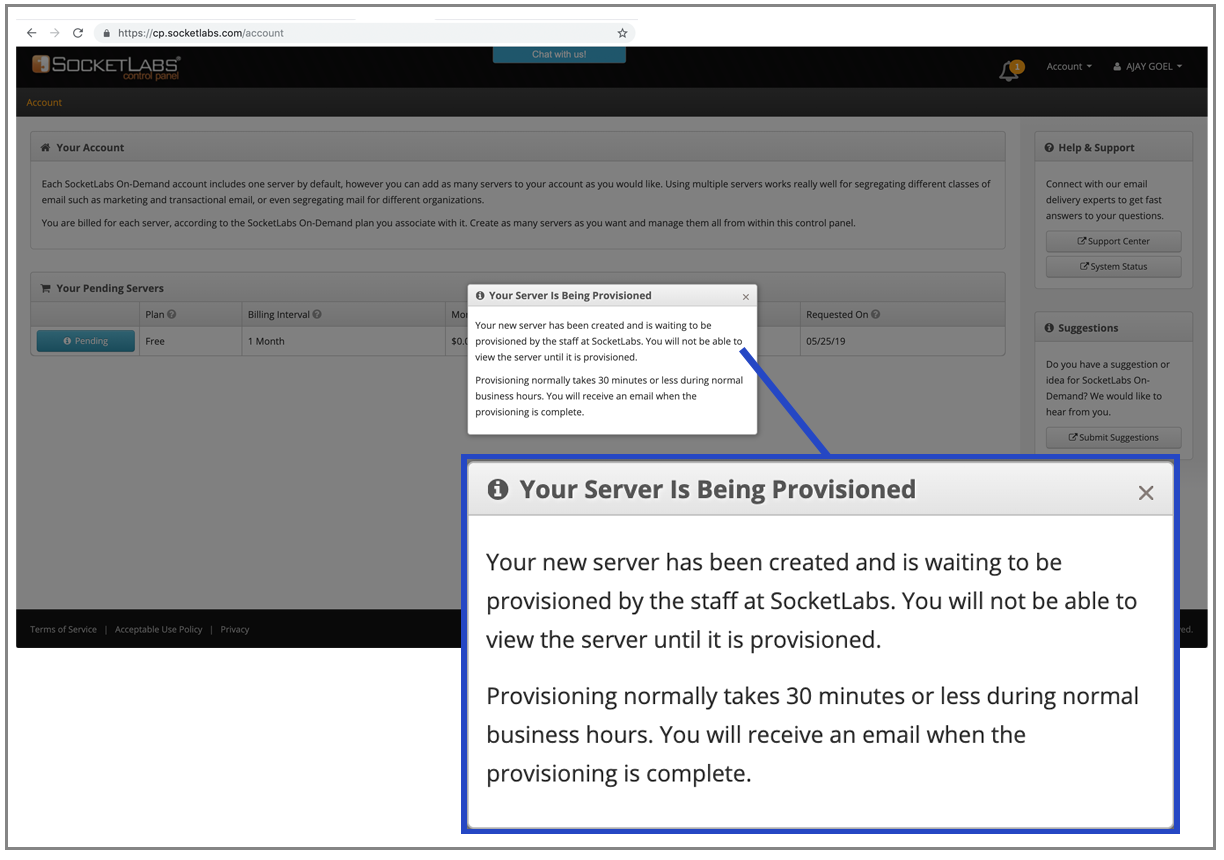
SocketLabs is the only SMTP provider with a concept of a “server” that has to be provisioned.
And unfortunately, you can’t send “from” a gmail.com address.
The SocketLabs Acceptable Usage Policy specifically requires that your mail must be sent from an address at a domain that is currently registered to you and under your control.
“For example, you may not use a from address including a domain from consumer email addresses such as Yahoo, Hotmail, AOL or Gmail, etc. or any other domain not under your full ownership and control.”
Additionally, you have to designate all domains that will be used as a MAIL-FROM domain when you relay an email to its service. The MAIL-FROM will ultimately be modified when the email is transmitted from SocketLabs to the remote server, so all this means is that any domains you send “from” need to be listed and verified. Since when I signed up for the service, I used my address of [email protected], it made wordzen.com the default domain from which I’d send.
As the default, wordzen.com can’t be removed:
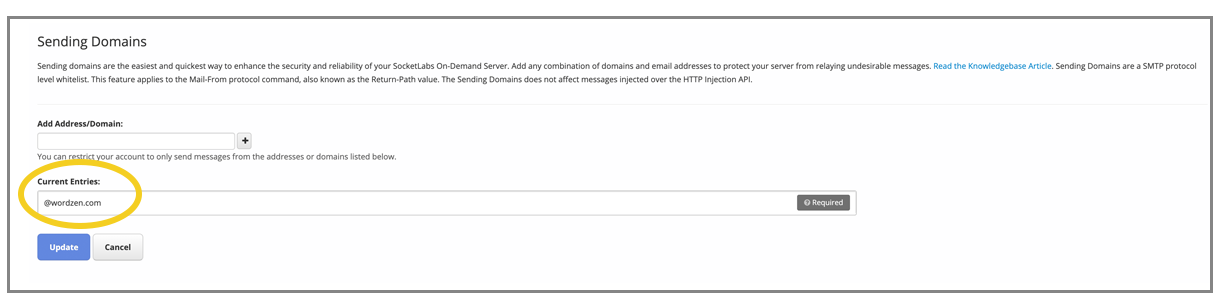
If I try to send an email “from” a domain that I haven’t listed, I get a rejection message immediately when I relay the email.
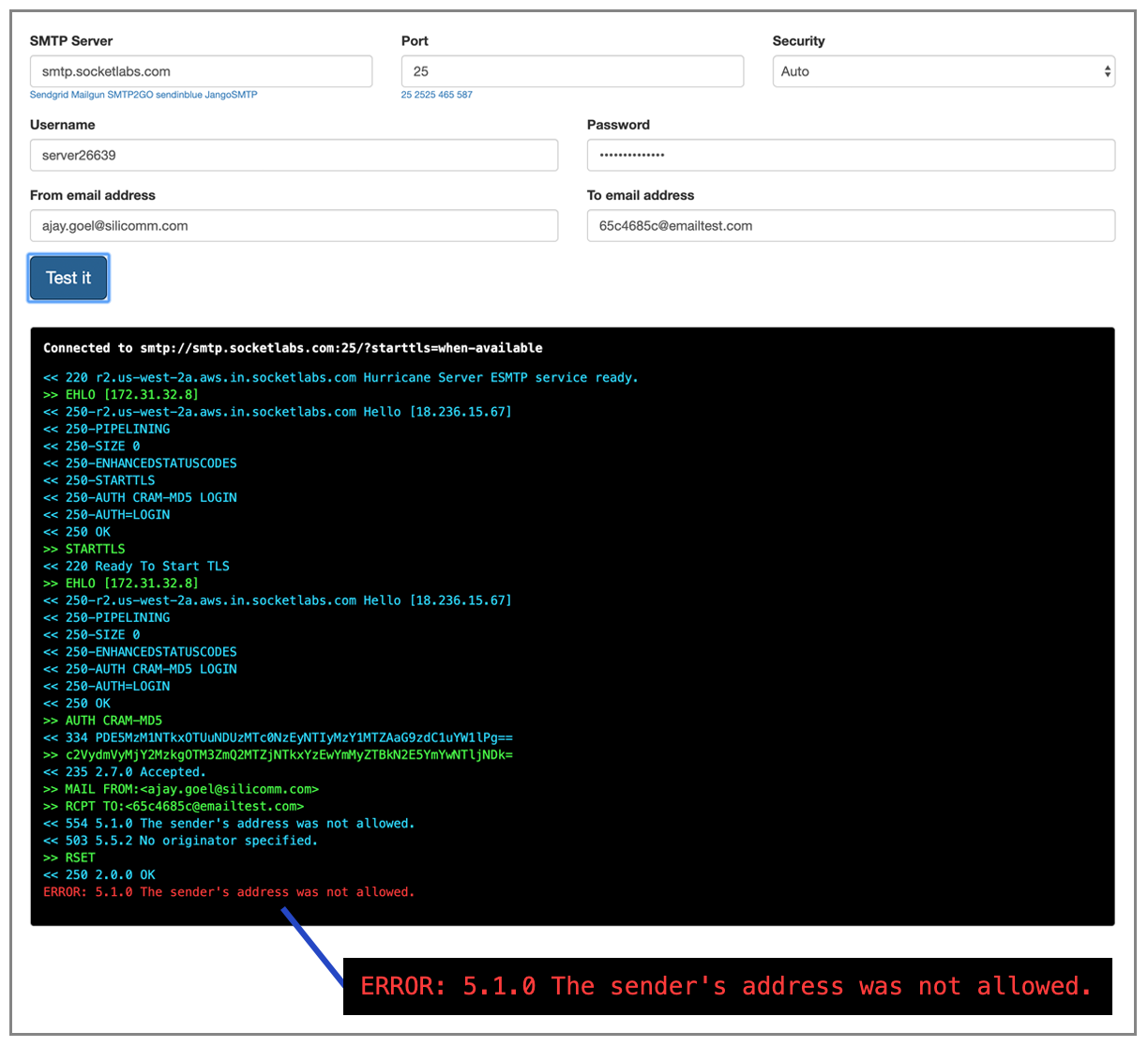
Even if you try to add an @gmail.com address, you are squarely denied:
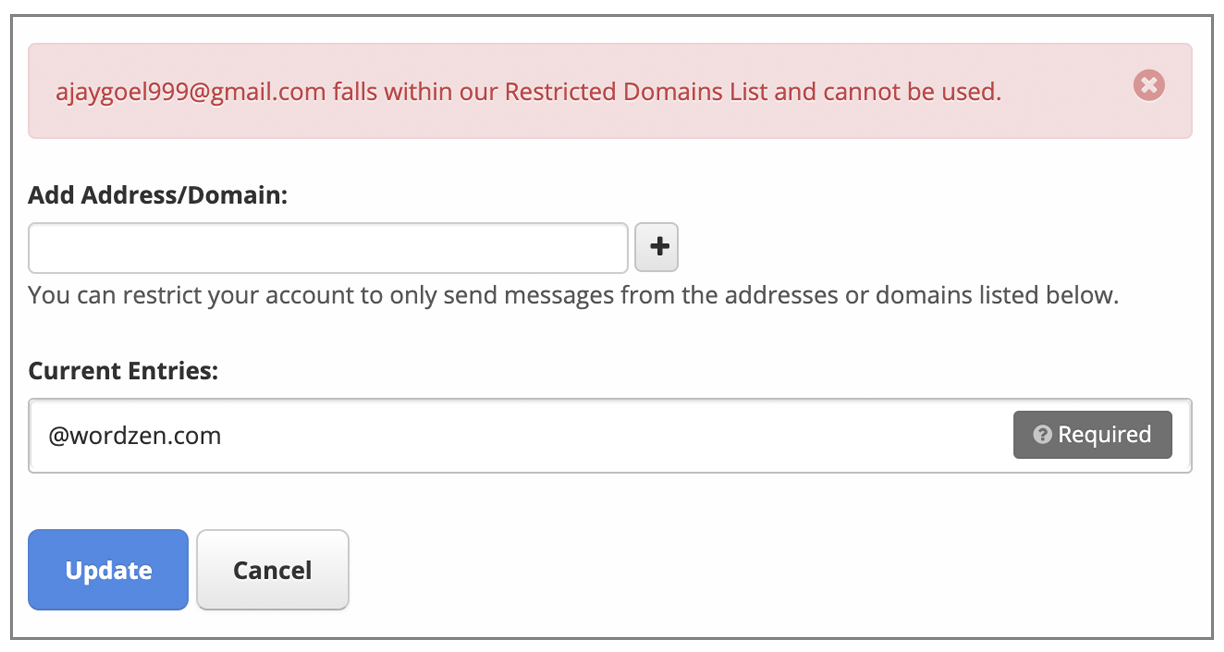
When I do send “from” [email protected] though, my email analyzer shows that it does use its own MAIL-FROM and DKIM signs with its own domain, which is useful because it makes SPF and DKIM pass out-of-the-box.
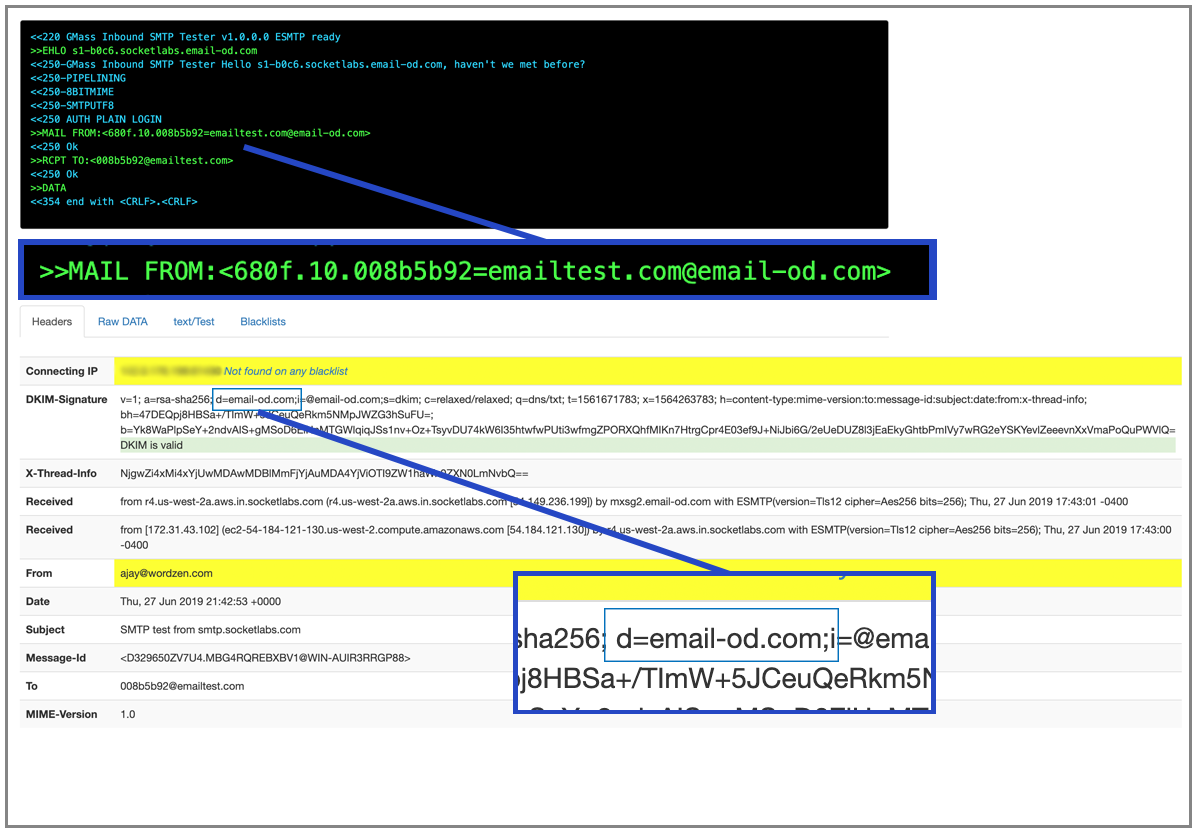
SocketLabs does offer a way to whitelist this and control the MAIL-FROM and the DKIM SIGNING DOMAIN.
Can the sending domain restriction be removed, so that I can send “from” any domain? I inquired with support and was told they would only do that if I subscribed at a higher level:
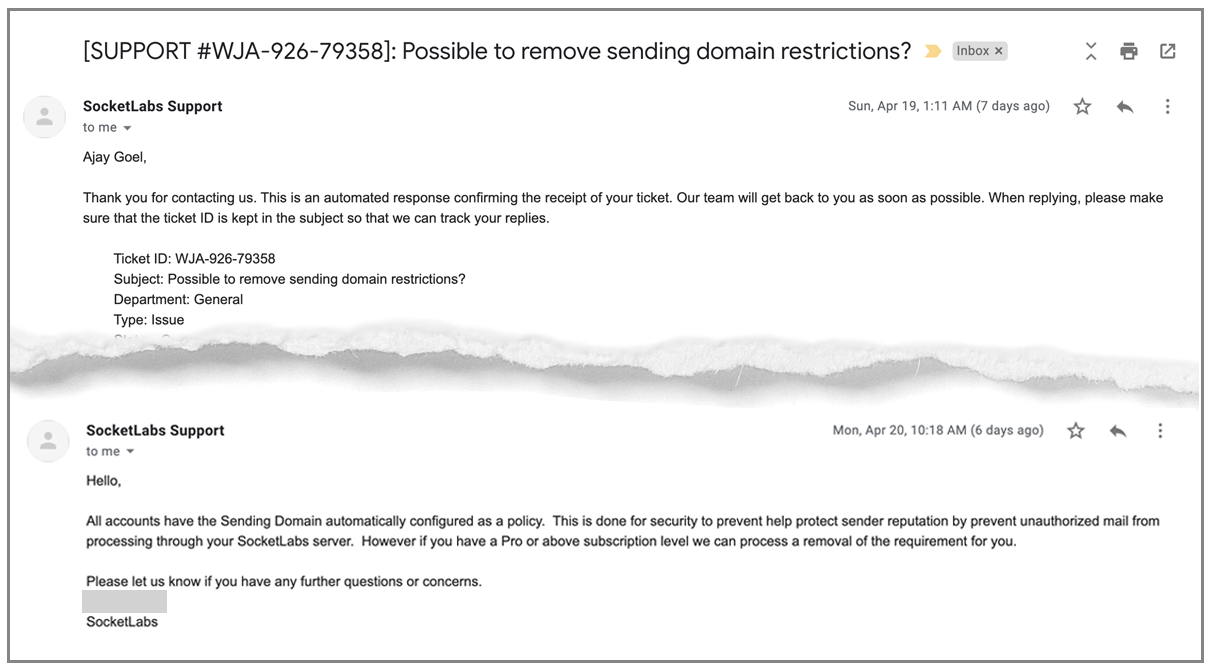
So if you’re sending from just one domain, the out-of-the-box setup is good. They’ll sign your emails for you, and you’ll pass SPF and all that.
Here is the allowed sender list:
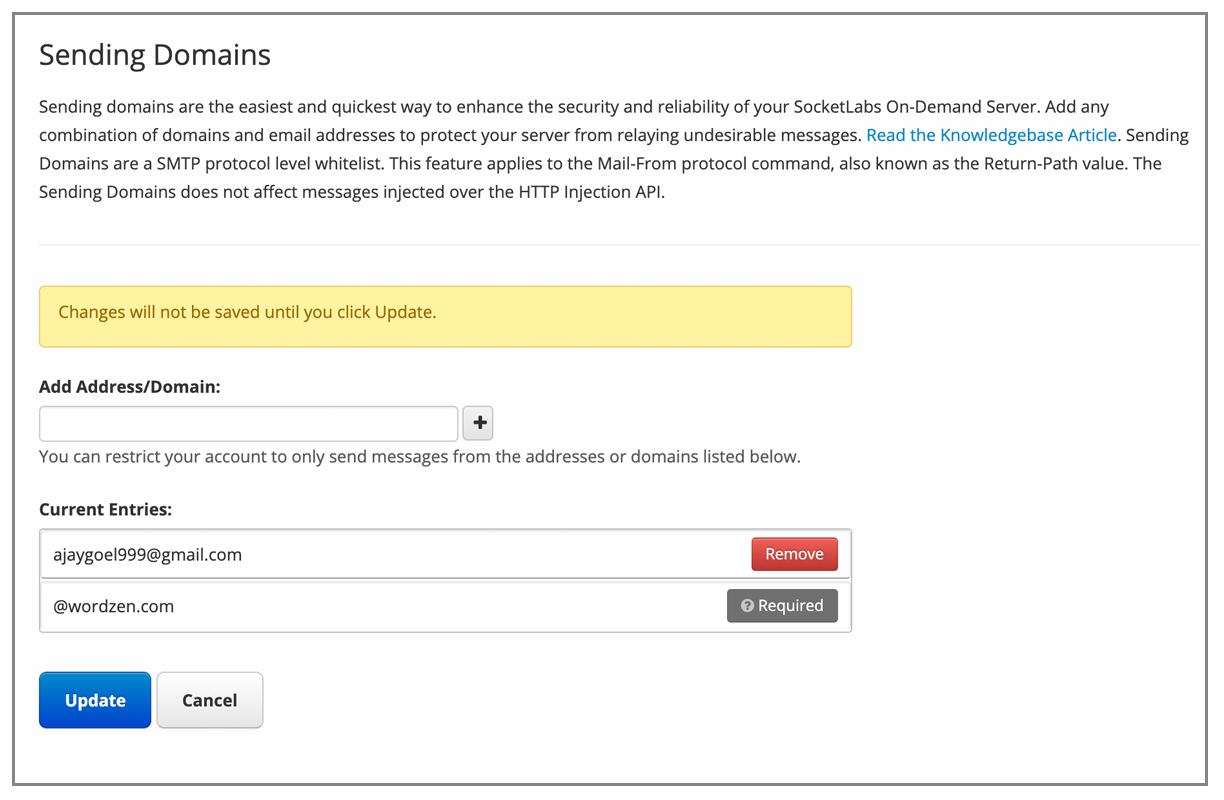
Let’s try something else, like [email protected]
Weird UI quirk
Despite the default sending being restricted to your original domain, it still makes you agree legally that you can only send from each address/domain on the list.
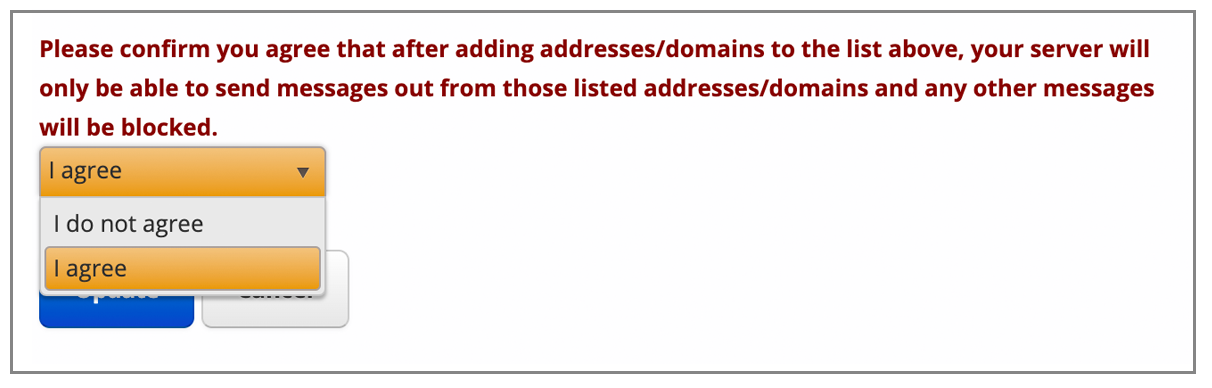
It’s like those old automative navigation systems where every time you started the car you had to agree to some bogus legal statement that you wouldn’t use the navigation system while driving.
Once you add an address, you can send from it, and they handle the MAIL-FROM and the DKIM signing. So, you don’t have to mess with any DNS records off the bat, which is beneficial.
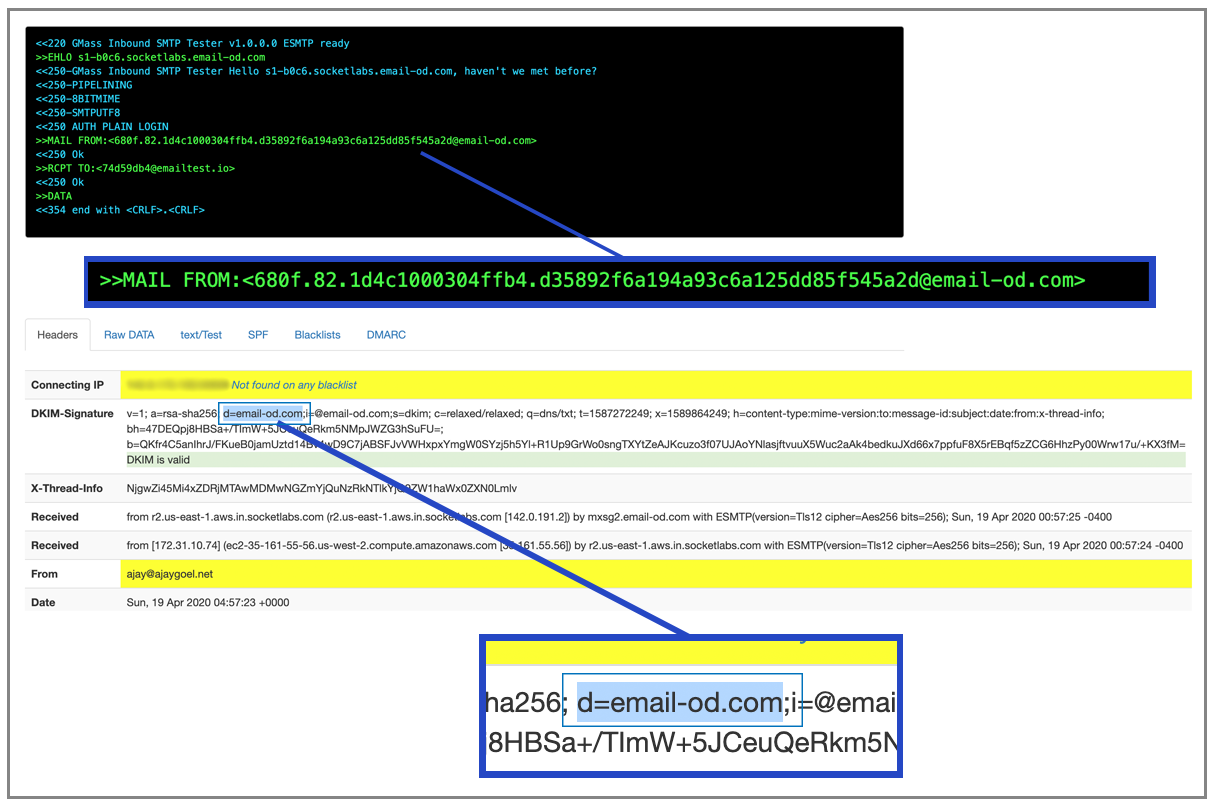
Still though, can the domain/address “from” restrictions be removed so that I don’t have to list each one? At least, I don’t have to verify each one, so I could add all my users, since I wouldn’t need to have them go through the extra step of clicking a link in their Inbox.
Fortunately if you want, you can DKIM-sign emails with your own domain:
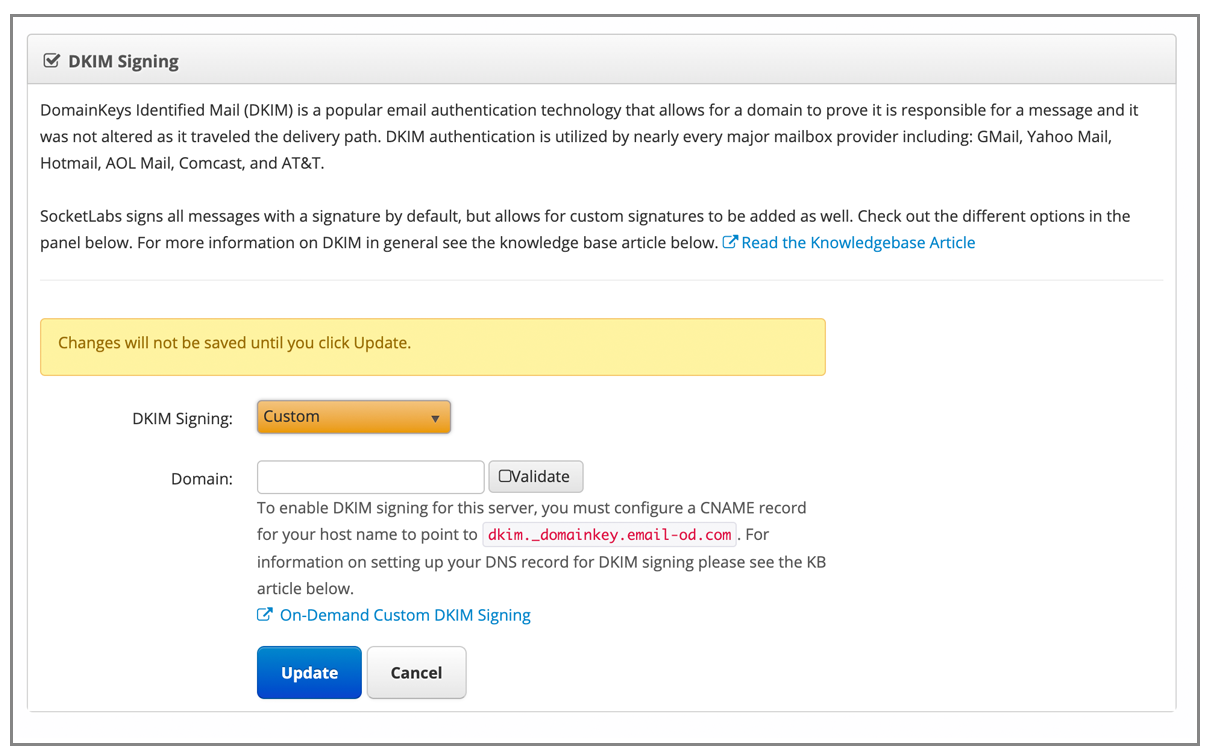
Looks like you can also control the MAIL-FROM header, referred to here as a “custom bounce domain.”
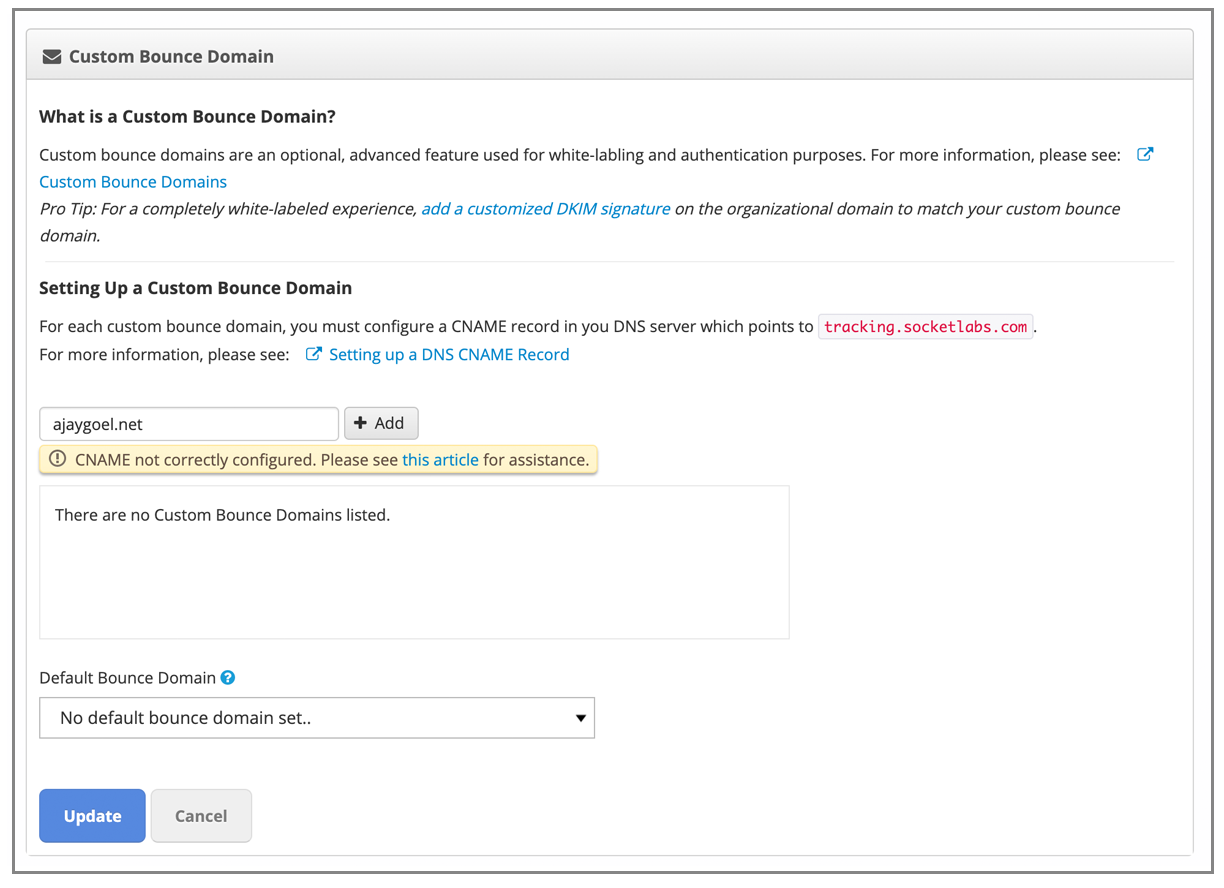
However, I still want to be able to send “from” any address. I’ll ask support if that can be done. Waiting for support to respond about that and the gmail.com issue.
IP Authentication
One thing to note to avoid confusion. SocketLabs offesr the ability to authenticate based on IP address rather than on what most services offer, which is authorization based on only username and password. That’s a nice feature, but be careful not to misconstrue what’s on their Pricing page:
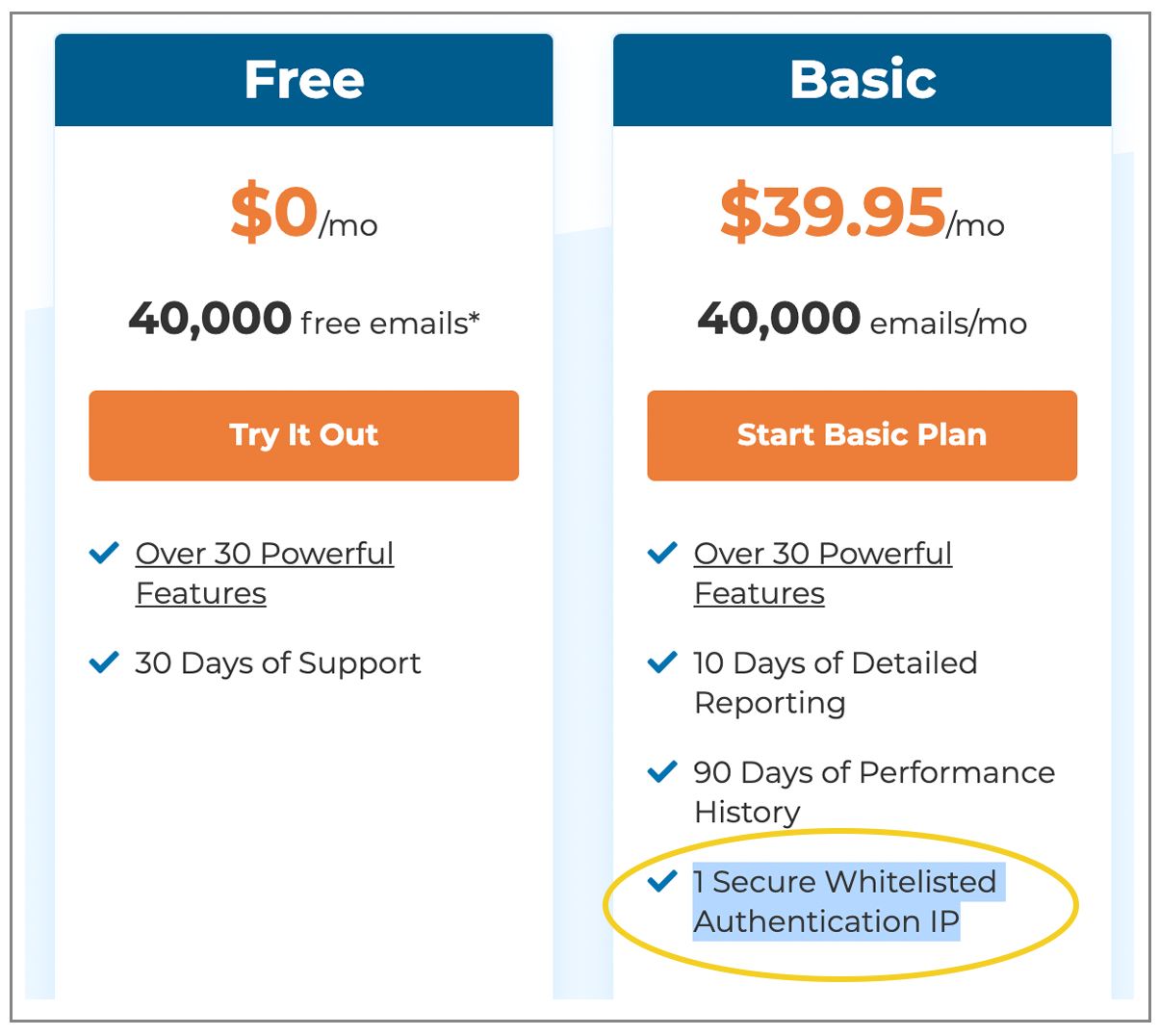
An “authenticated” IP is not a “dedicated” IP. An authenticated IP is just the IP you specify that will be relaying the emails from you to them. A dedicated IP means you have a sending IP dedicated just for your use and no other SocketLabs customers.
The skinny on SocketLabs
It’s nice that they use their own MAIL-FROM and DKIM-sign your emails with their own domain out-of-the-box, but the service is too restrictive because you can’t send “from” your @gmail.com account. Also, you have to enter every domain or address that you want to send “from,” and agree to a ridiculous statement each time you enter something new. The concept of a “server” is strange too, since it’s unlikely they’re setting up an actual physical (or virtual) server for every new customer.
Grade: B-
Read my non-technical SocketLabs review.
Mailjet
(back to top)
I like how you login, go to the Transaction section, and boom — your SMTP credentials are right there. So far, they’ve made it the easiest out of all services to find your SMTP credentials and begin relaying email.
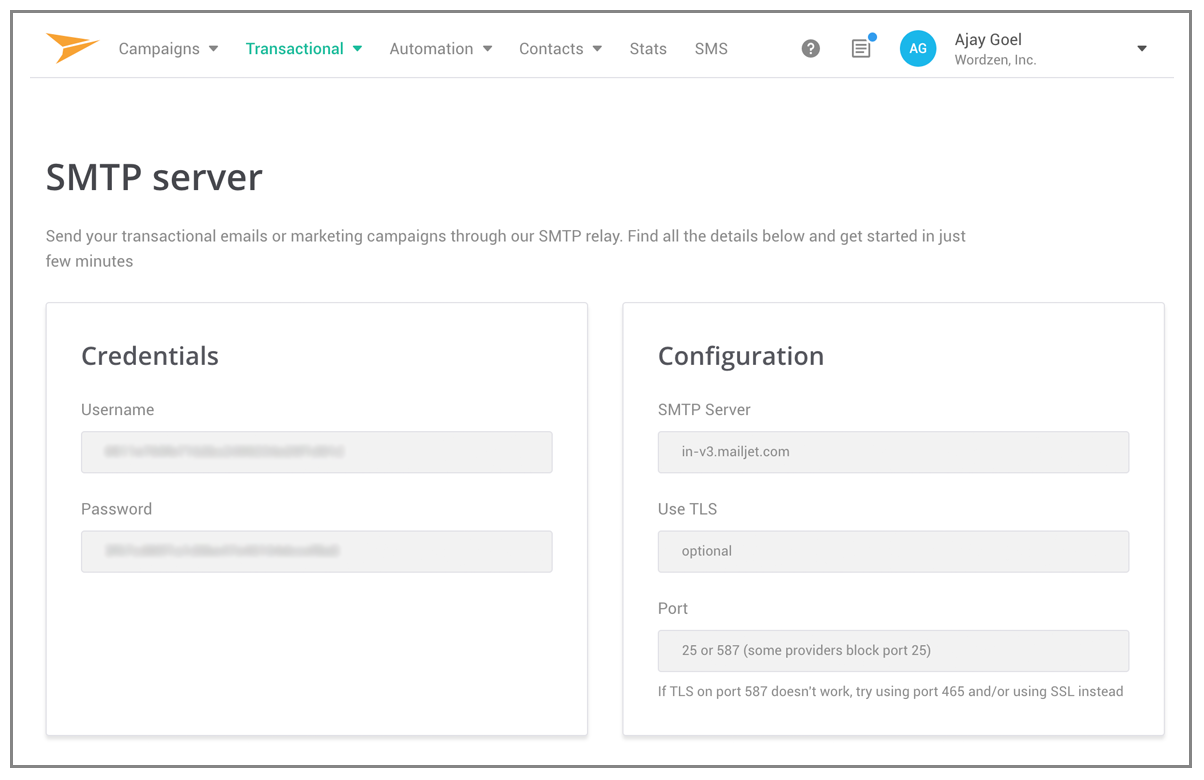
You don’t have to create a “server”, or create an “API key” or set your SMTP password. It just gives it to you. Easy.
Out of the box set-up, SPF and DKIM work since they’re using their own domain as the MAIL-FROM and as the DKIM signing domain.
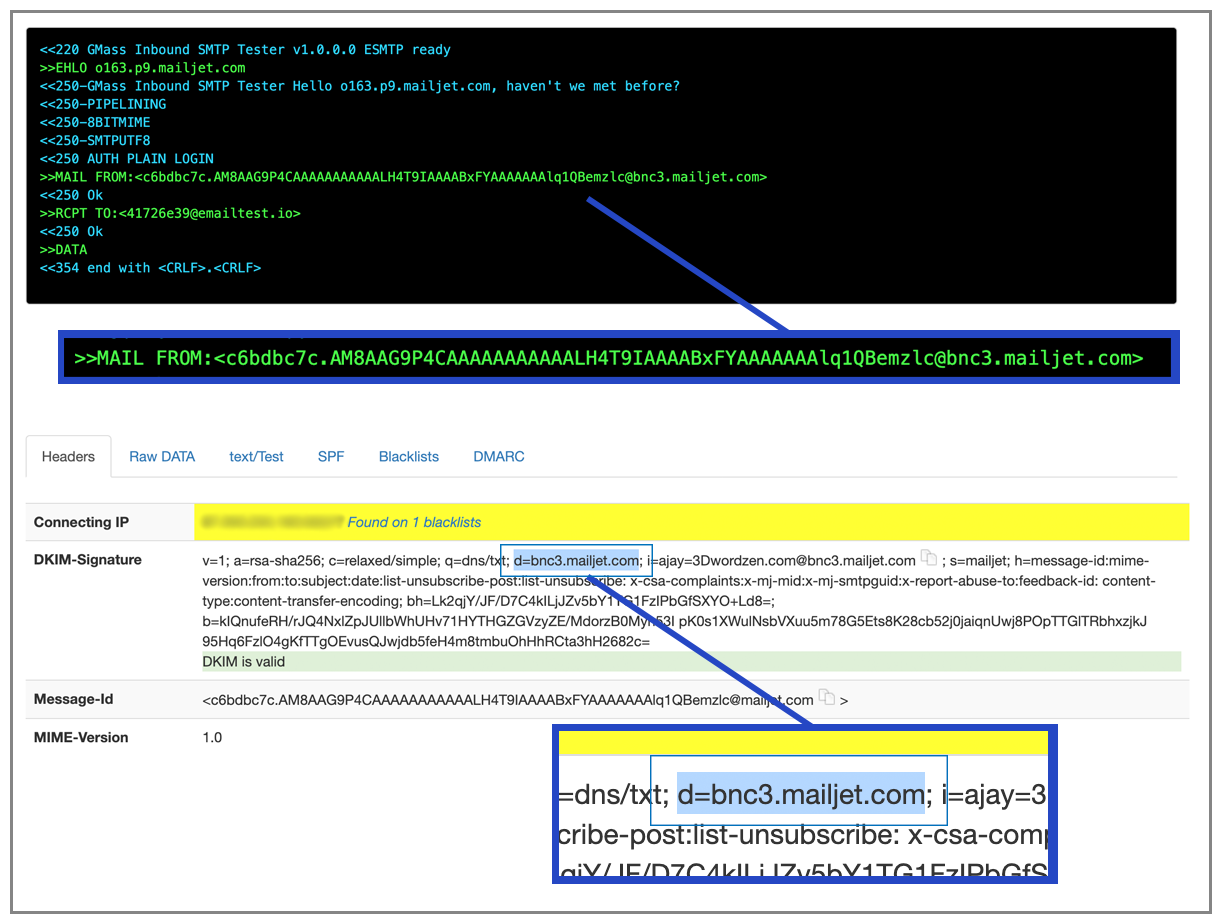
MAIL-FROM domain is: c6bdbc7c.AM8AAG9P4CAAAAAAAAAAALH4T9IAAAABxFYAAAAAAAlq1QBemzlc@bnc3.mailjet.com
The email is signed with the domain bnc3.mailjet.com.
Unfortunately, you can’t just send from any address. You have to set up each address individually and click a confirmation link, which stinks. That means I can’t just let 100 of my users use this, without putting them through a manual process of asking them to click a link in their Inbox.
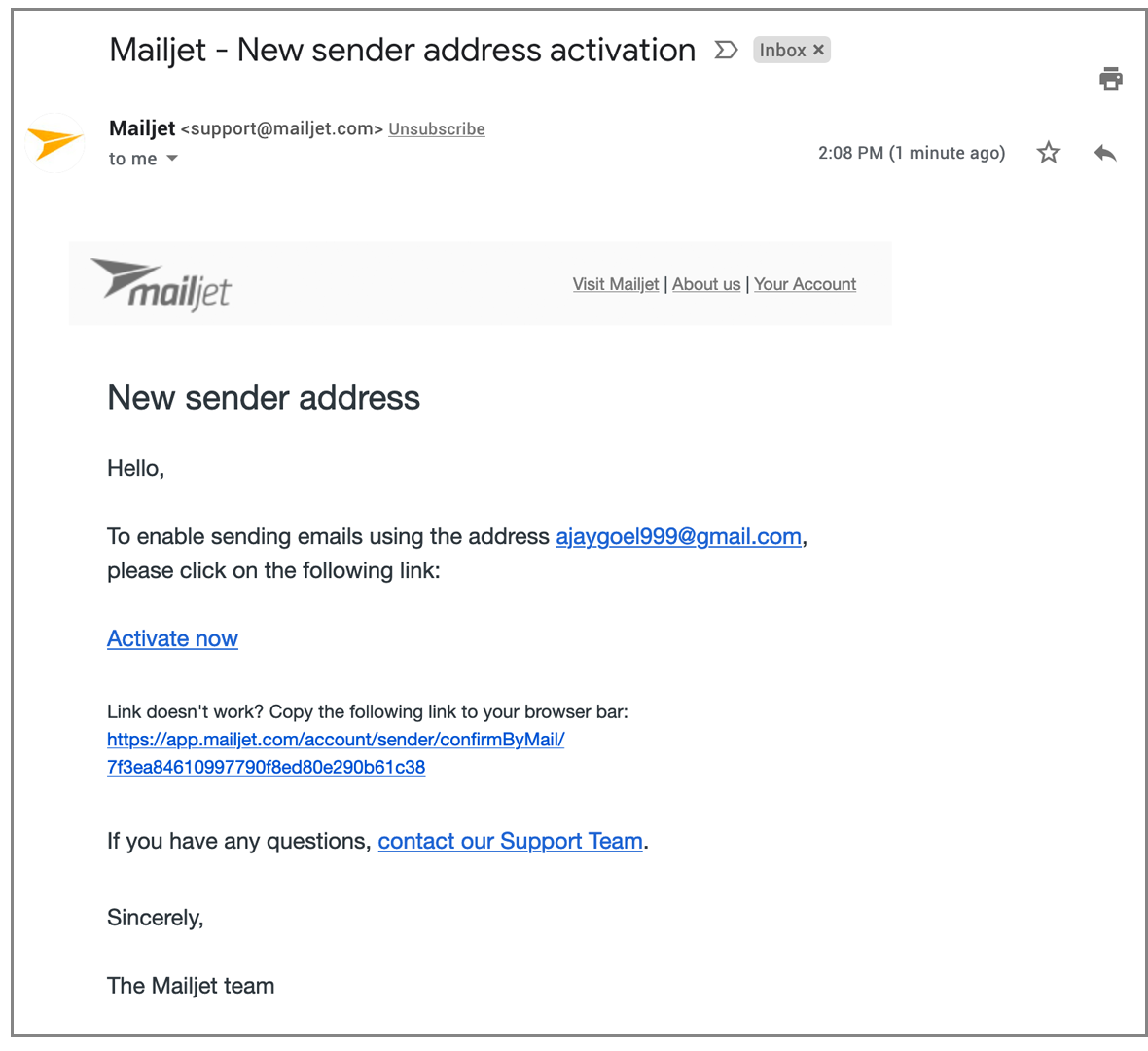
Annoying UI Quirk
Major pet-peave coming up: when you click the link in the email to verify the new “from” address, if you’re not already logged in to Mailjet, you have to log in to complete the verification. The link should include a unique token which proves I’m the proper recipient of the link. That would make verification seamless.
Here I’m sending “from” [email protected], which has not been validated as a “from” address, but the SMTP relay fully accepts it, and I don’t know anything is wrong:
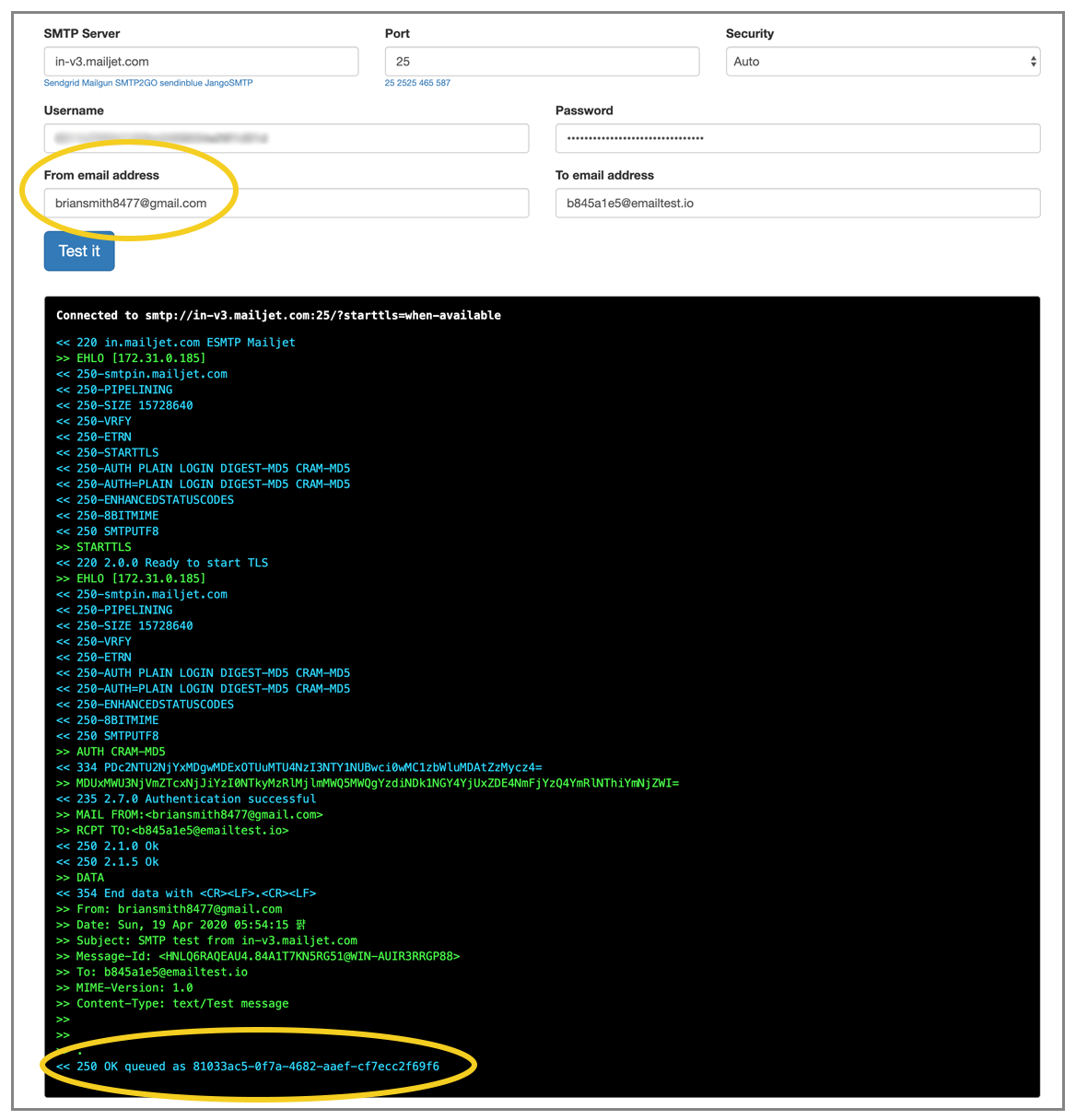
Unless I go over to “Sender domain and addresses”, which in itself is hard to find (click your name on right, then account settings, then sender domain/addresses)
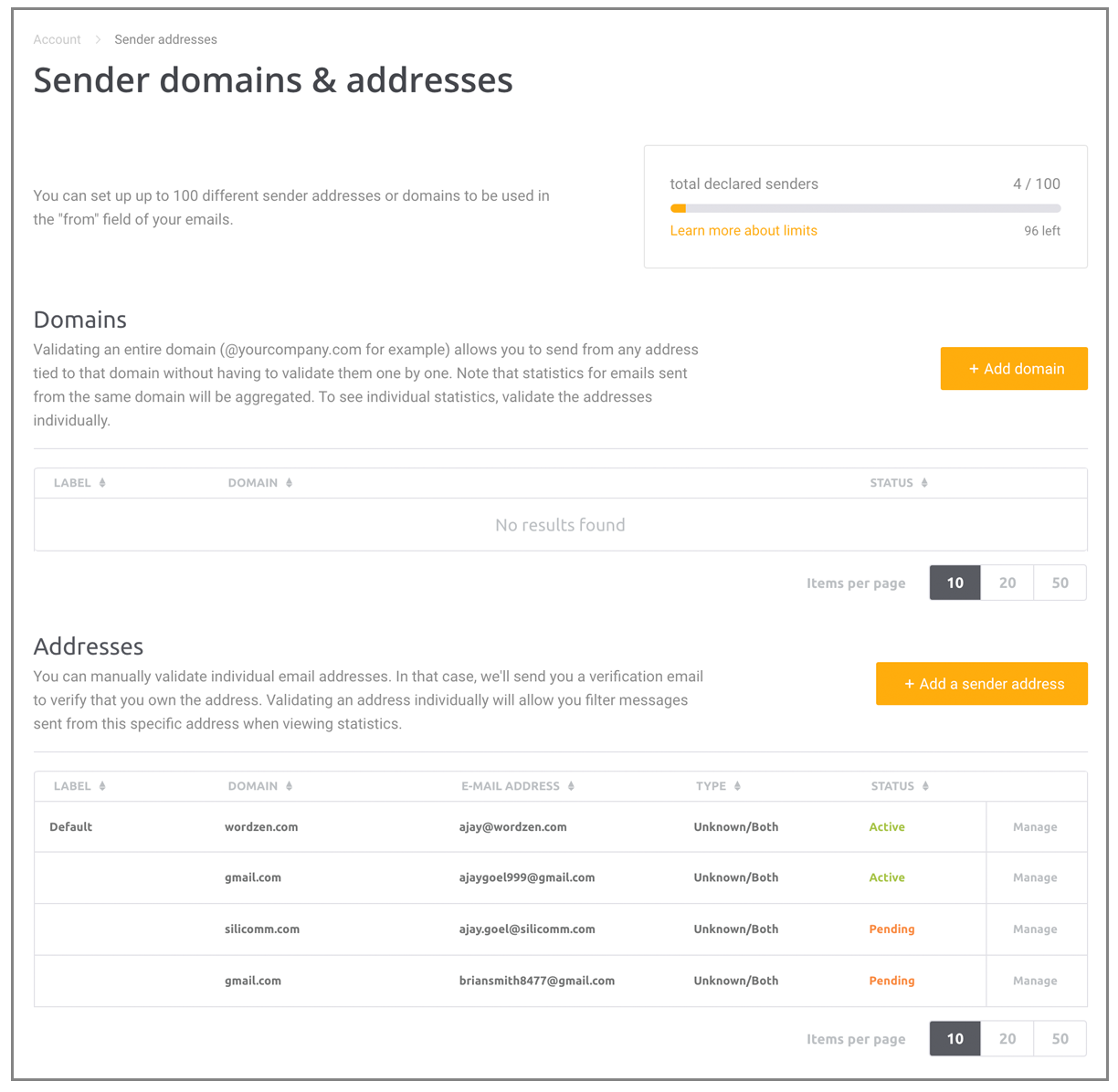
You CAN sign emails with your own domain, although I didn’t test it:
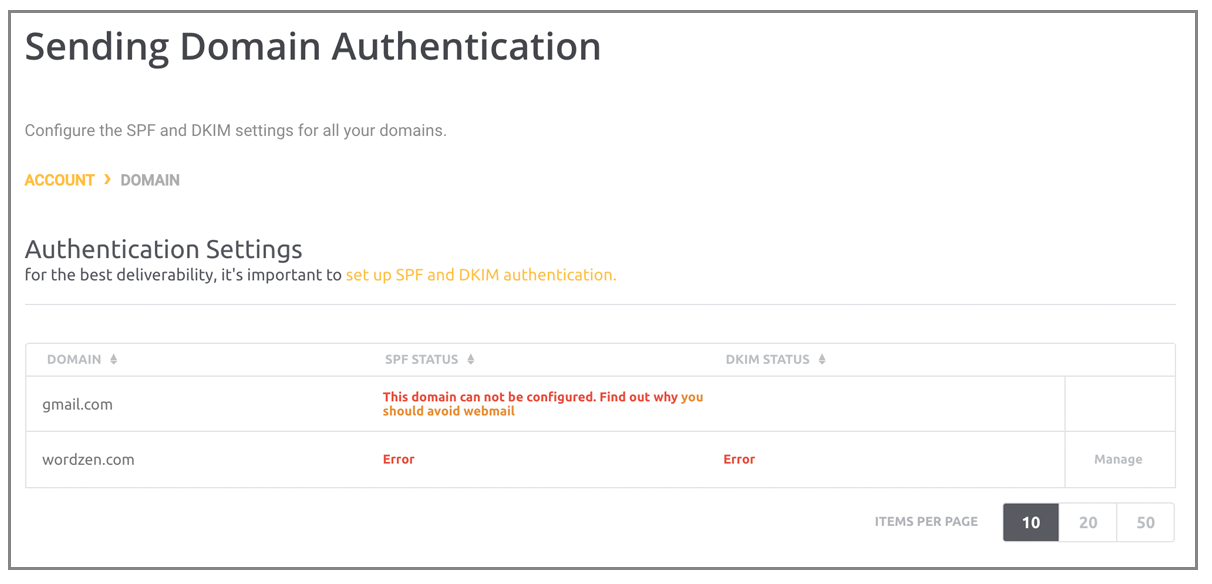
Can you control the MAIL-FROM header?
It gives you the option to set up SPF, but it’s clunky. I’ve added the text to my SPF record, as seen here:
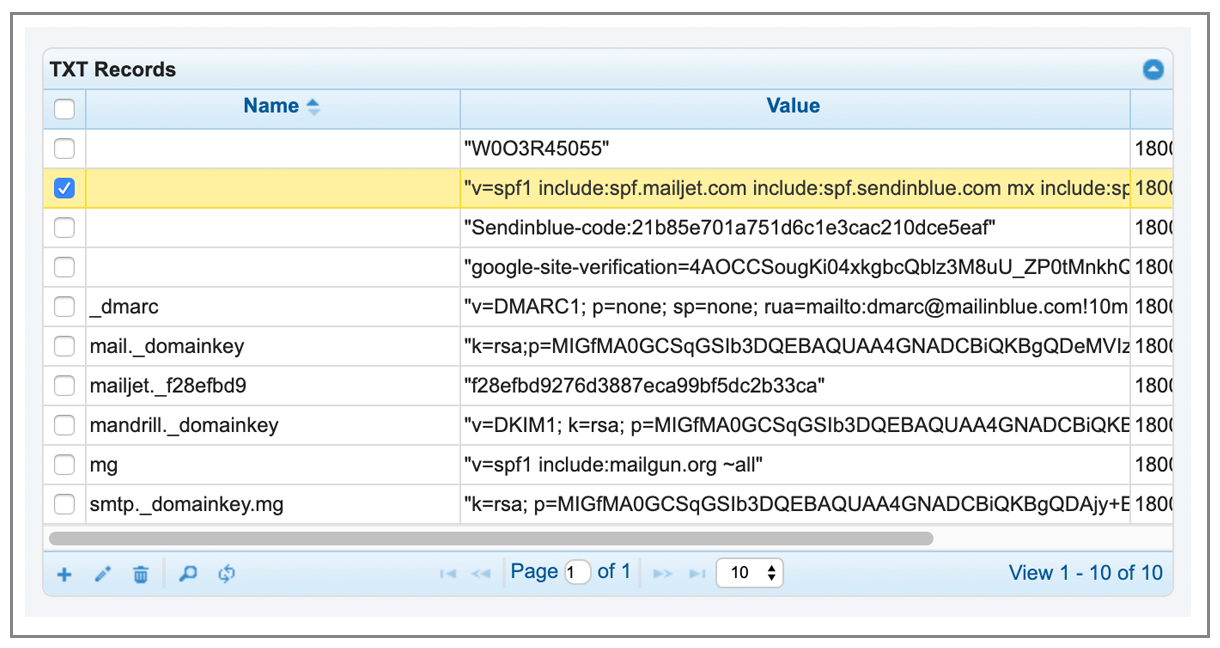
But it still “thinks” that it’s not added. It’s likely caching the DNS records rather than retrieving it freshly.
Never mind, after 3 minutes, the “refresh” validated the SPF record:
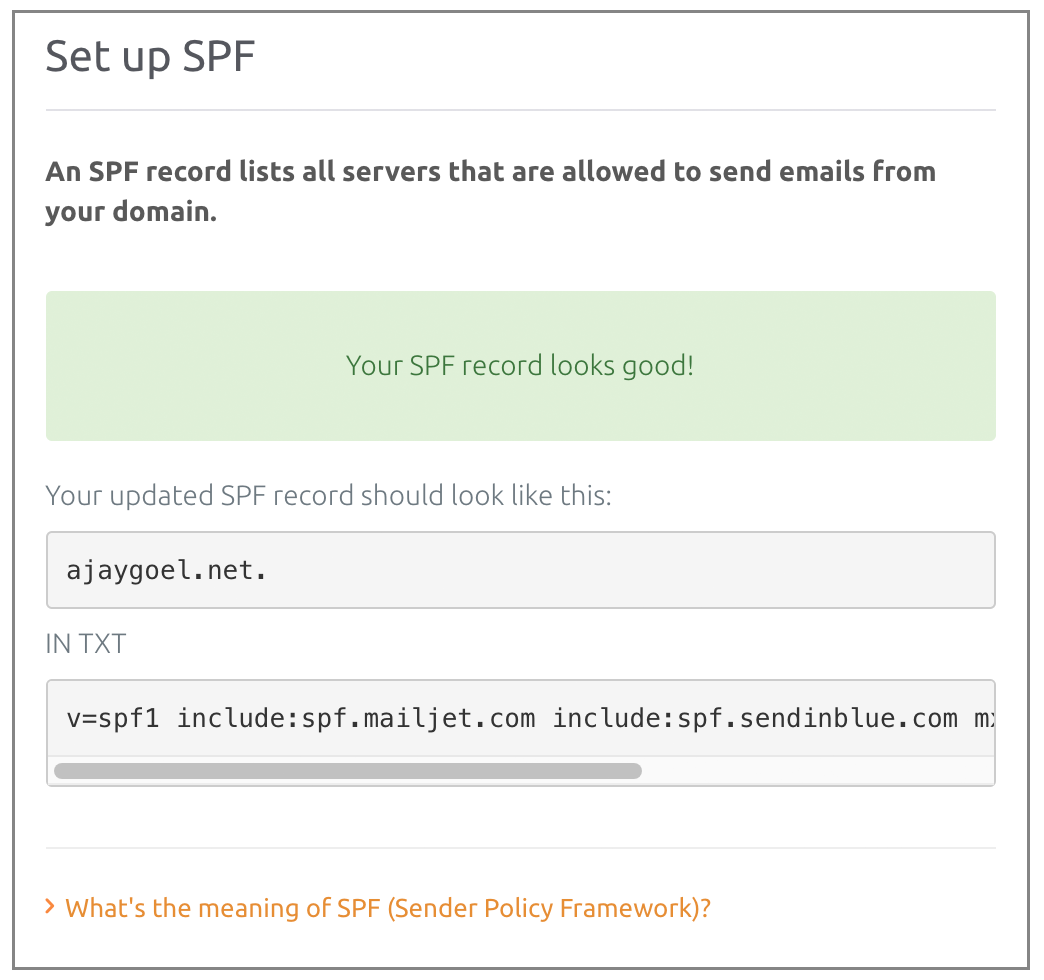
Just a typical case of the web UI not reflecting what’s happening in real time.
Ugh, after being told my SPF is good to go, it still says “Error” — UI is bad.
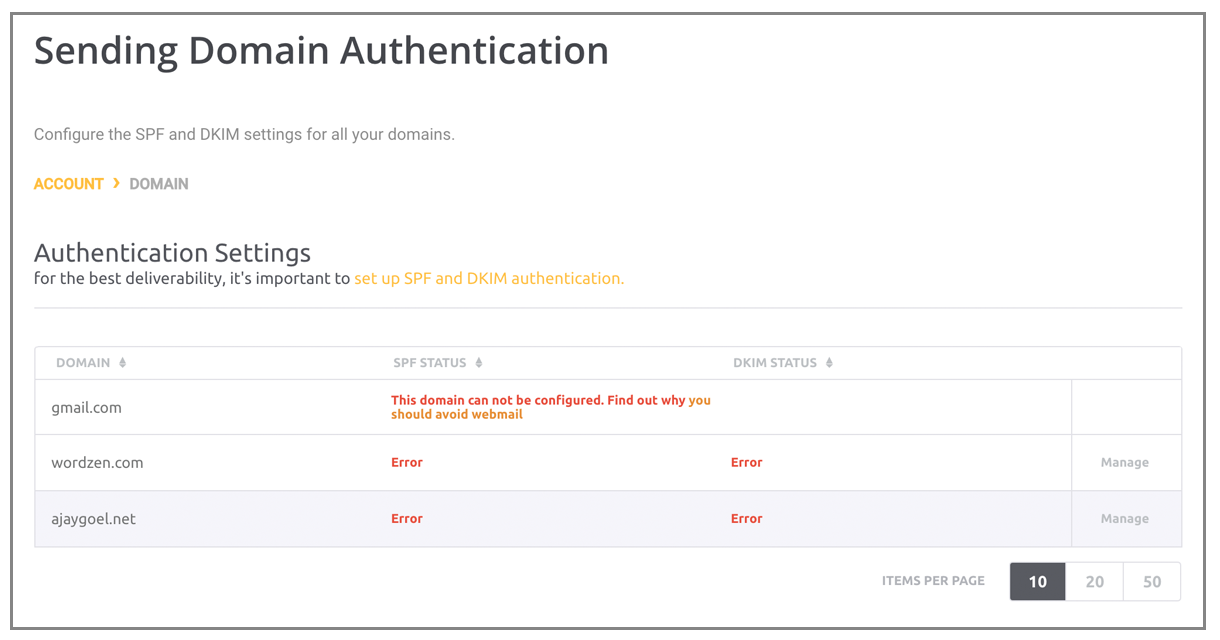
And now back to this, ugh:
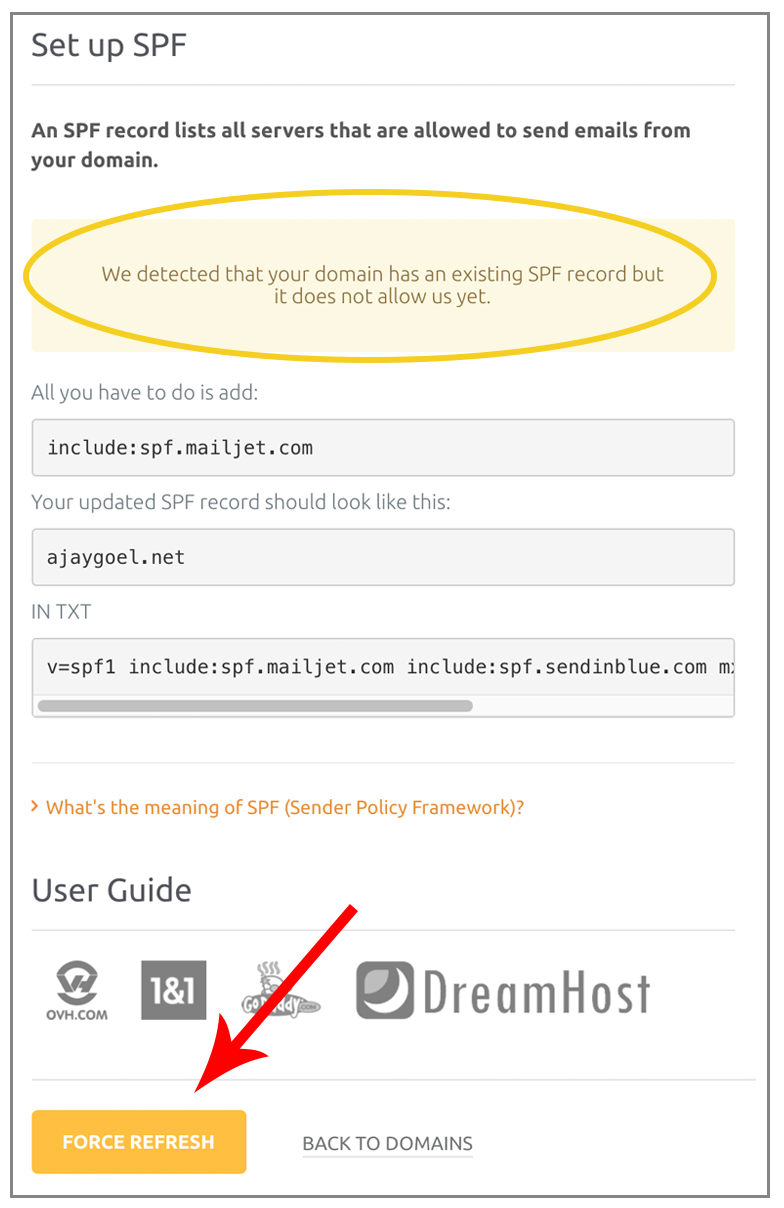
Hitting Force Refresh is a crapshoot, sometimes it says “Your SPF record looks good!” and sometimes ti says it can’t be found.
Hmm, even after setting up SPF for ajaygoel.net and sending an email through, the MAIL-FROM is still @bnc3.mailjet.com
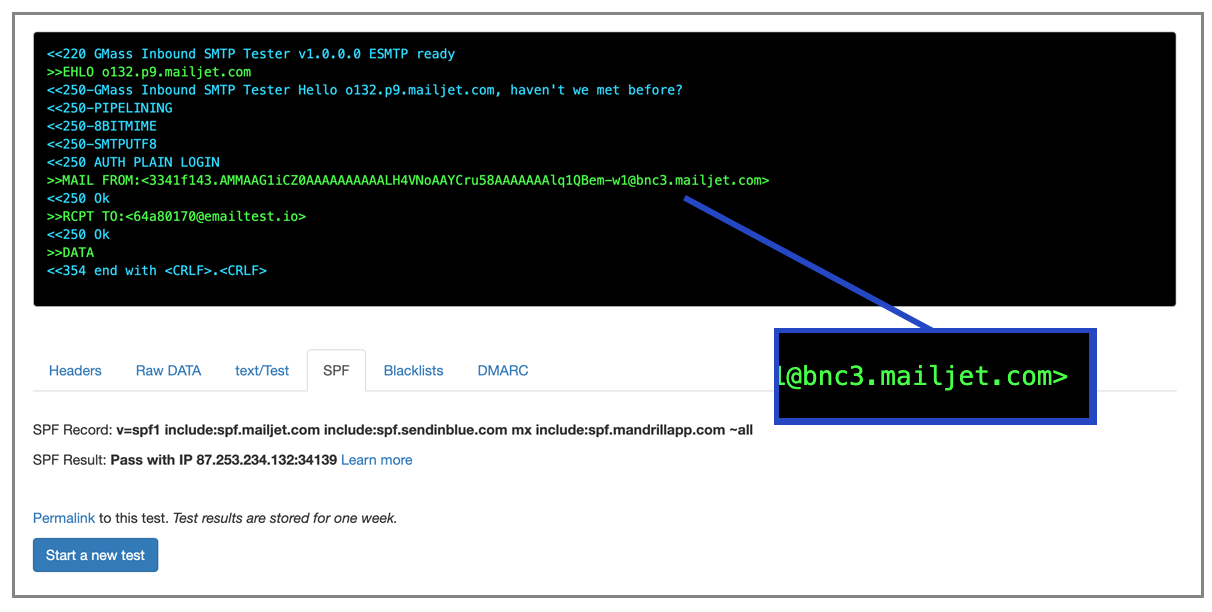
I asked support to remove the sending address/domain restrictions and I was squarely denied:
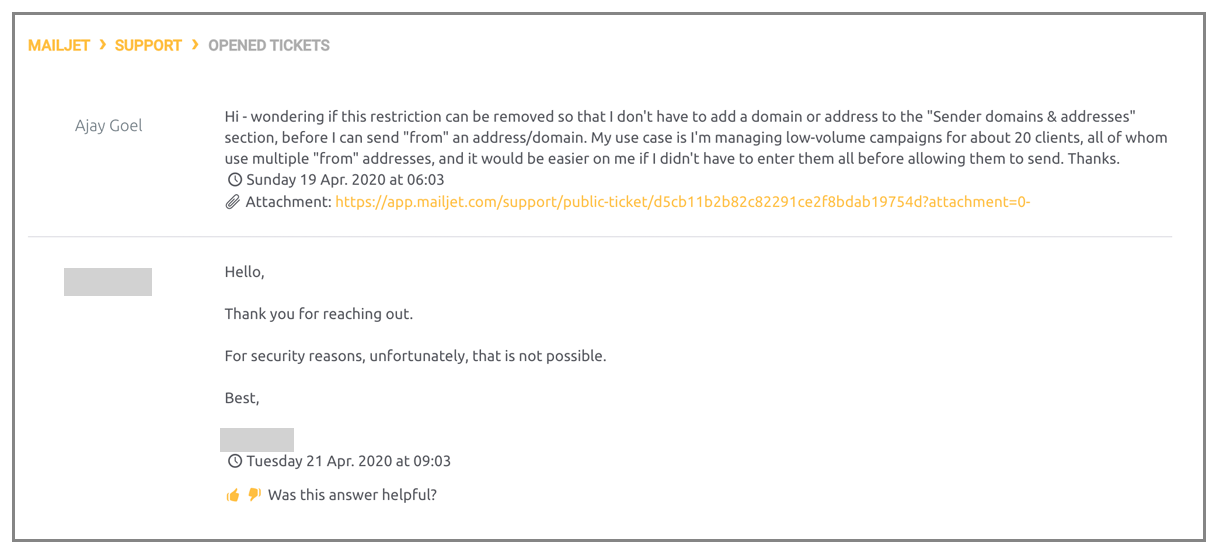
The skinny on Mailjet:
Like other services, it’s nice that SPF and DKIM work out of the box, but there are a number of UI issues, it’s painful to verify individual “from” addresses because not only does a link have to be clicked for each one, but that link couldn’t even be clicked by my random users since being logged in is also required and I wouldn’t give out the web credentials to 100 users. It is nice though that you can send “from” your @gmail.com account. When you don’t have a “from” address verified, if you try to send from it, the relay accepts it and notifies you later (over the web UI and by email) that you have a pending email that hasn’t been delivered because it’s on hold because the “from” address hasn’t been verified. This has the upside of you not having to re-transmit the email to the relay if you forgot to verify your “from” address, but it has the downside of you potentially never knowing that your account is mis-configured since the relay will still accept the email.
Grade: C
Read my non-technical Mailjet review.
Mandrill
(back to top)
The first thing I noticed about Mandrill is that to begin to use Mandrill you first have to sign up and pay for a MailChimp account, and then you have to add on a Mandrill plan. That alone is quite the barrier for someone who only wants an SMTP relay service. So if you’re not already in love with MailChimp, Mandrill is likely not the transactional email service for you.
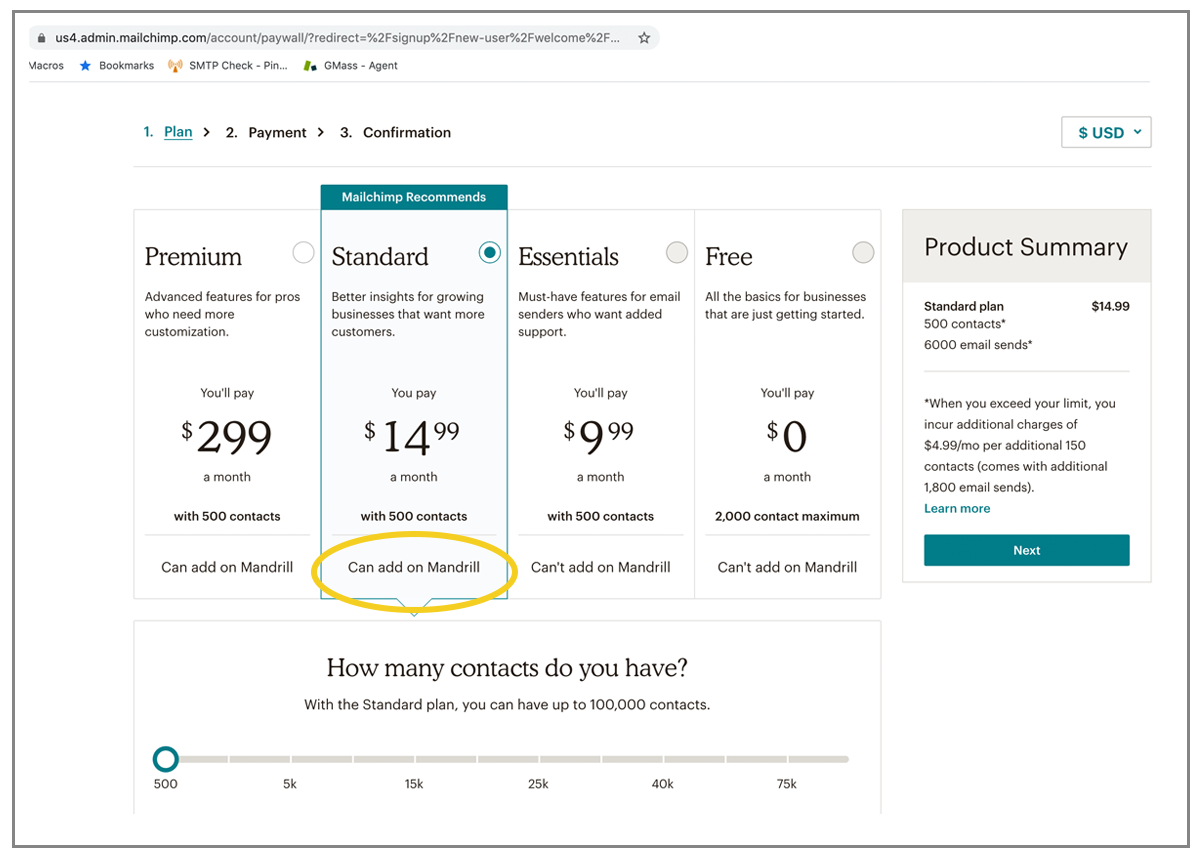
Here’s a weird quirk. Generally speaking, an SMTP username should be a string of characters without any spaces or punctuation. Notice the SMTP username that Mandrill generated for me, based on my company name. “Wordzen, Inc.” does not make for a good username to transmit over SMTP, but it turns out it doesn’t mater. It’s just for show. The username can be anything and as long as the password is correct, you’ll successfully authenticate into their SMTP service.
Now, onto the service.
First, you can’t try anything for free. Secondly first you have to sign up for a regular MailChimp account, and then yo have to pay again to add on Mandrill, and the minimum block is 25,000 emails. So you’re already about $35 in by the time you’re set up.
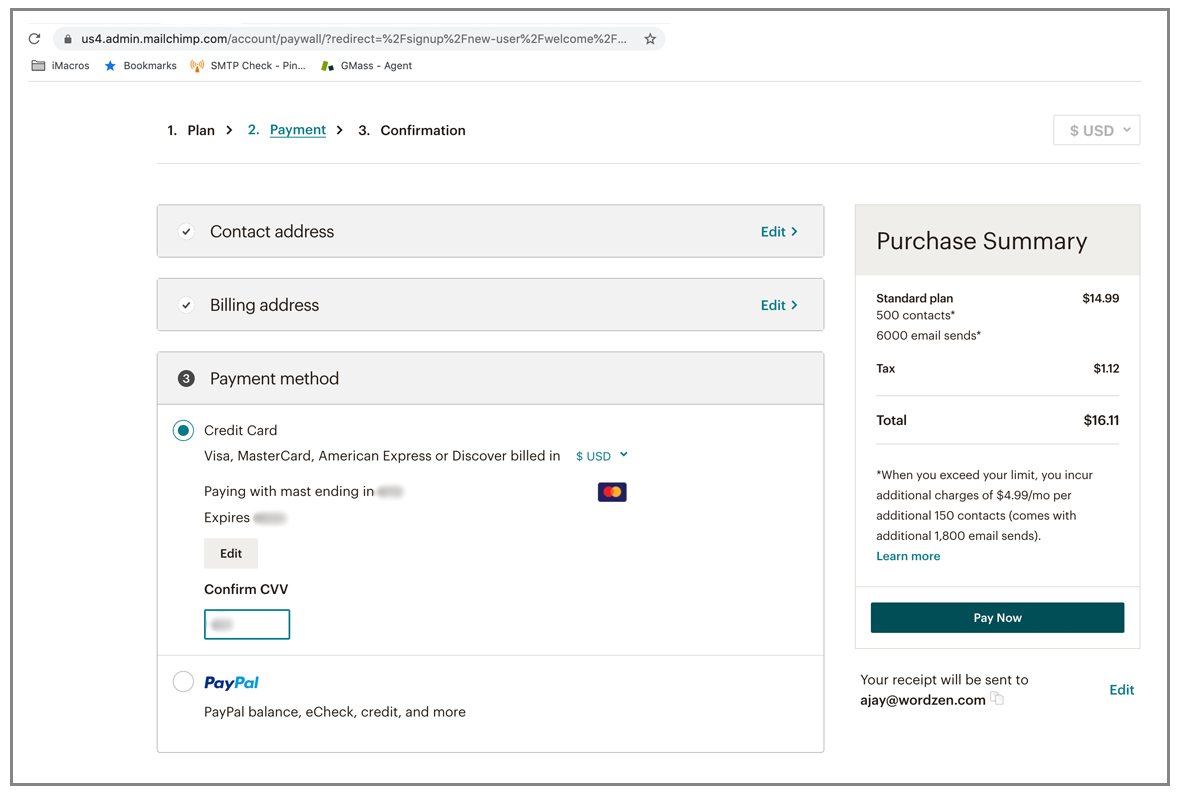
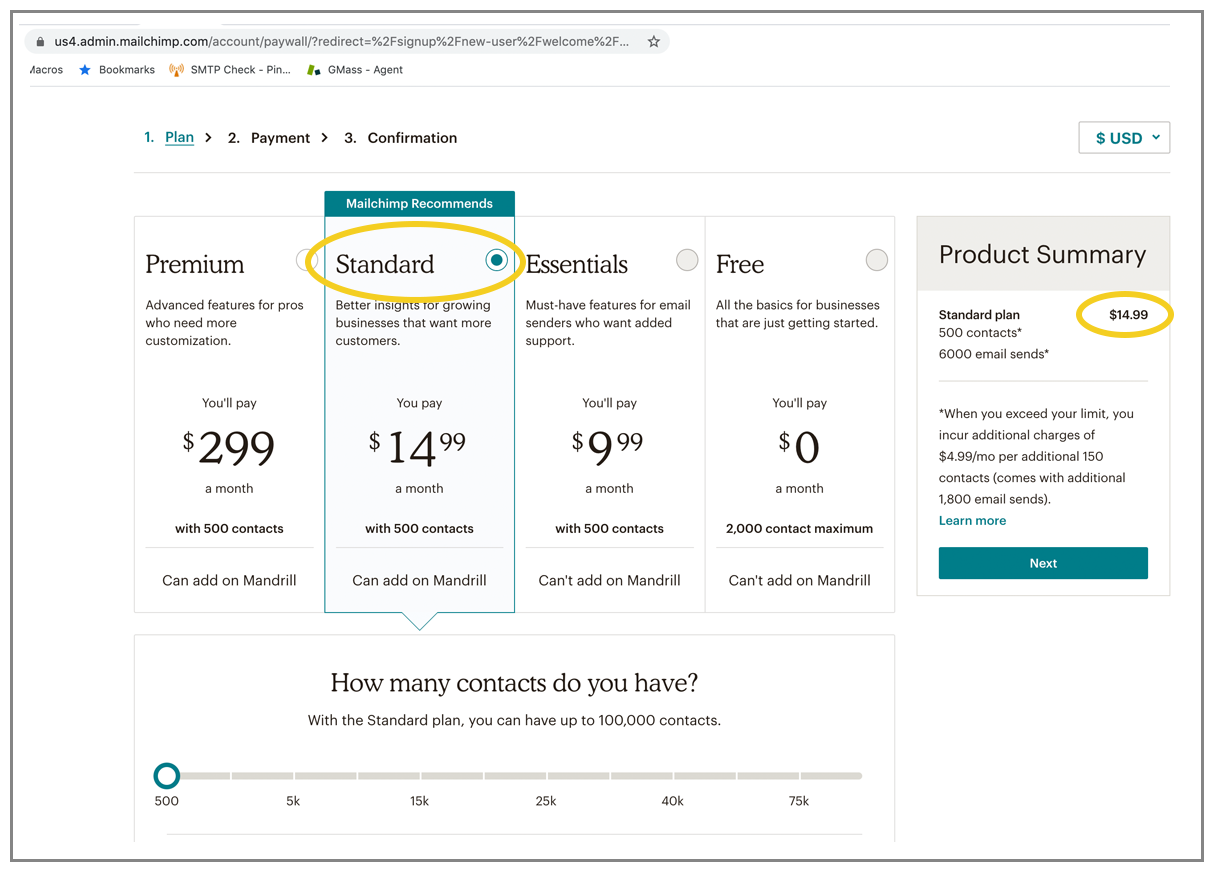
then you pay for mandrill
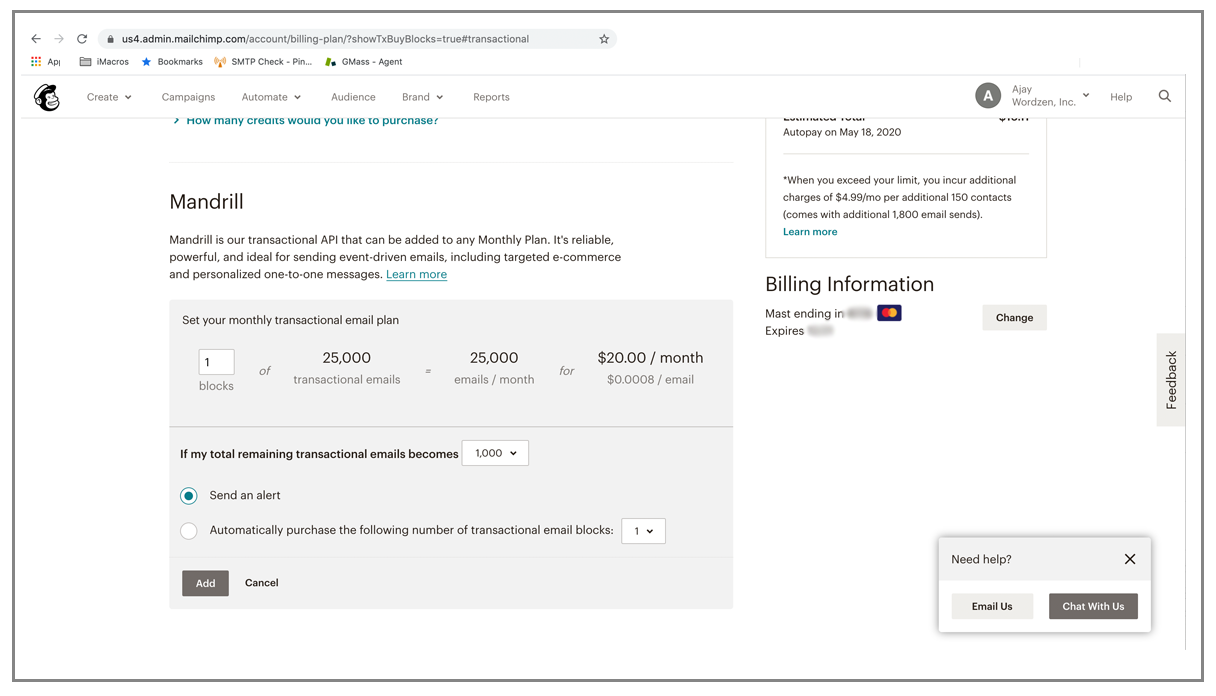
So, after signing up twice and paying twice, you MUST set up a domain with them before you can even relay a test email. This makes Mandrill the second most difficult service to sign up and begin testing, next to SMTP.com.
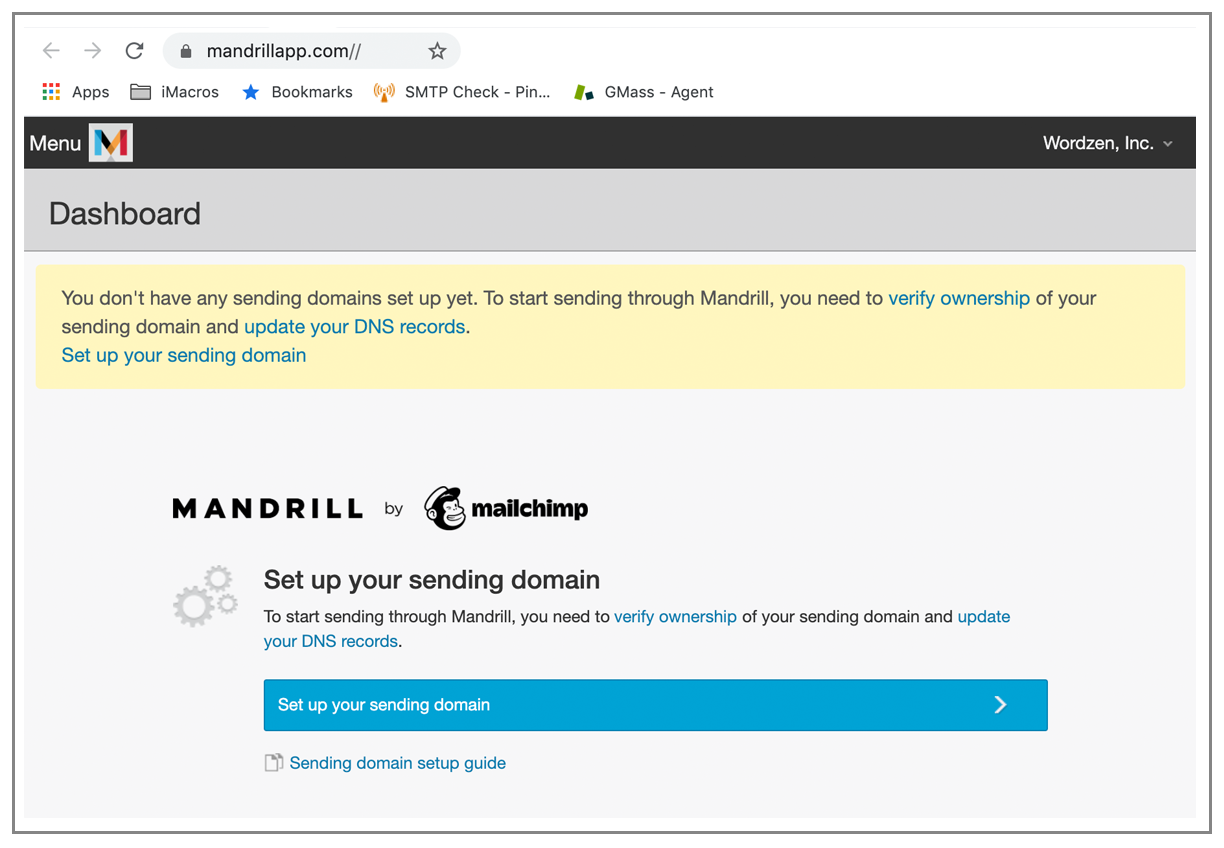
If you don’t set up a domain, the SMTP relay will actually accept your mail relays, but the emails won’t be delivered, and if you go to the “Outbound” section of Mandrill, you’ll see they’ve all been rejected
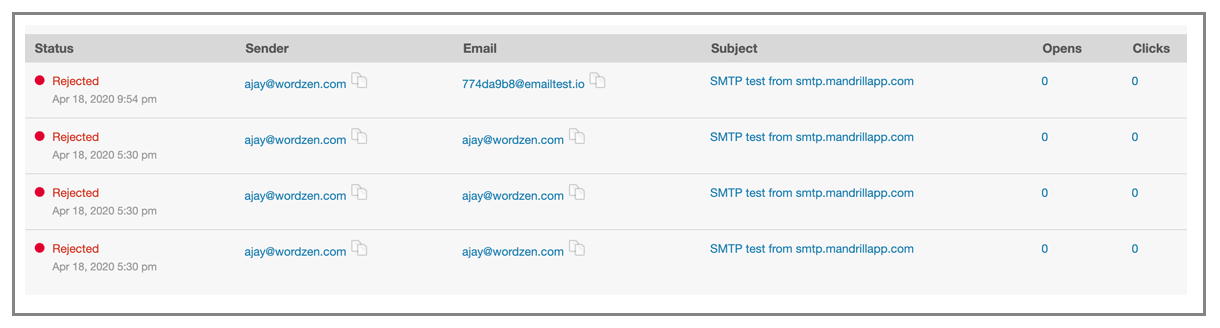
Because I’m curious, I went through with the sending domain setup process:
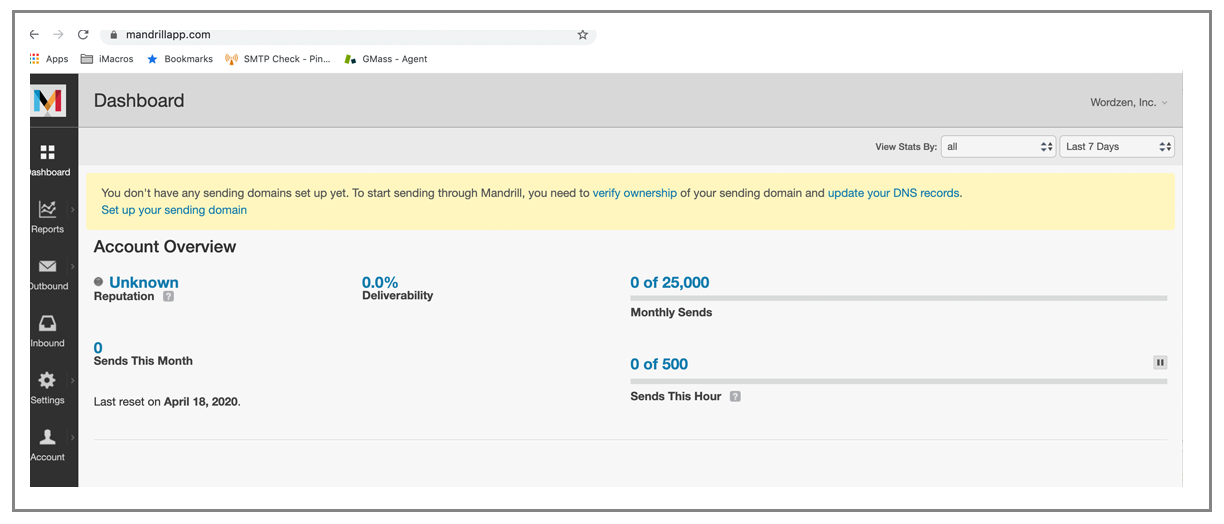
Mandrill is SMARTER than SendInBlue with its SPF setup. IT doesn’t tell me to replace my record or create one, but it looks at my existing record and tells me to modify it to include Mandrill’s IP pool:
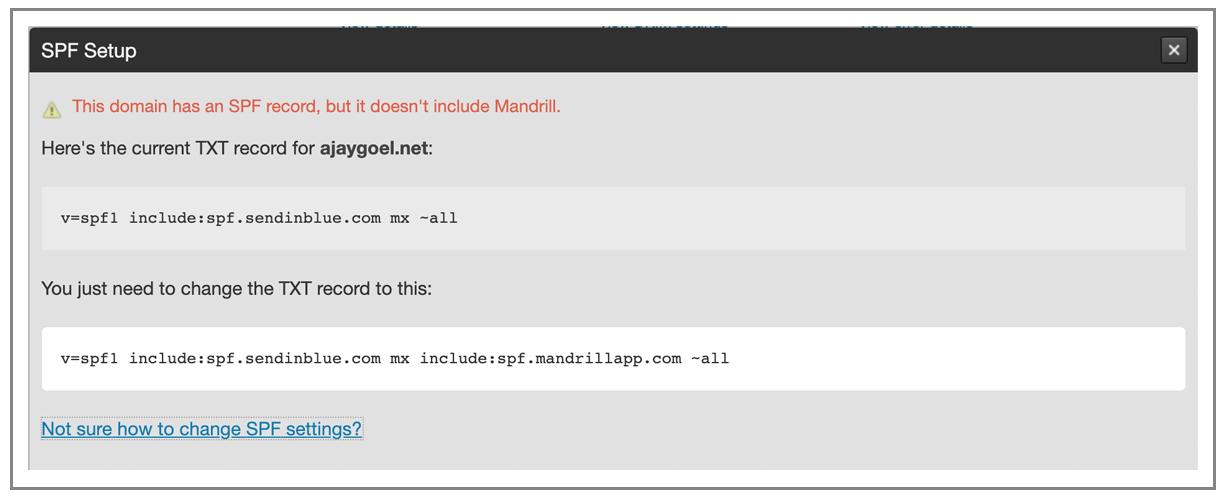
Mandrill is really a pain. Even after verifying ownership of the domain and setting up SPF to give Mandrill permission, I’m still getting rejected because the emails aren’t signed. Who knew that DKIM is a requirement? It shouldn’t be:
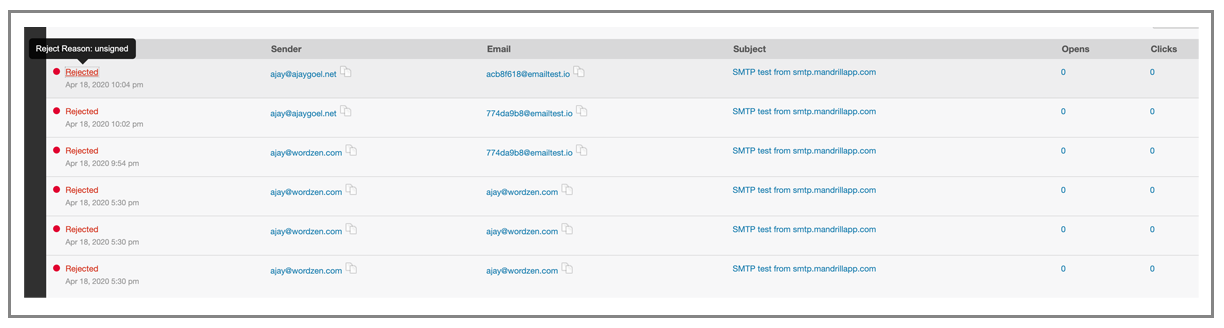
Alright, let’s set up DKIM
I did it for ajaygoel.net. And finally a test email gets through:
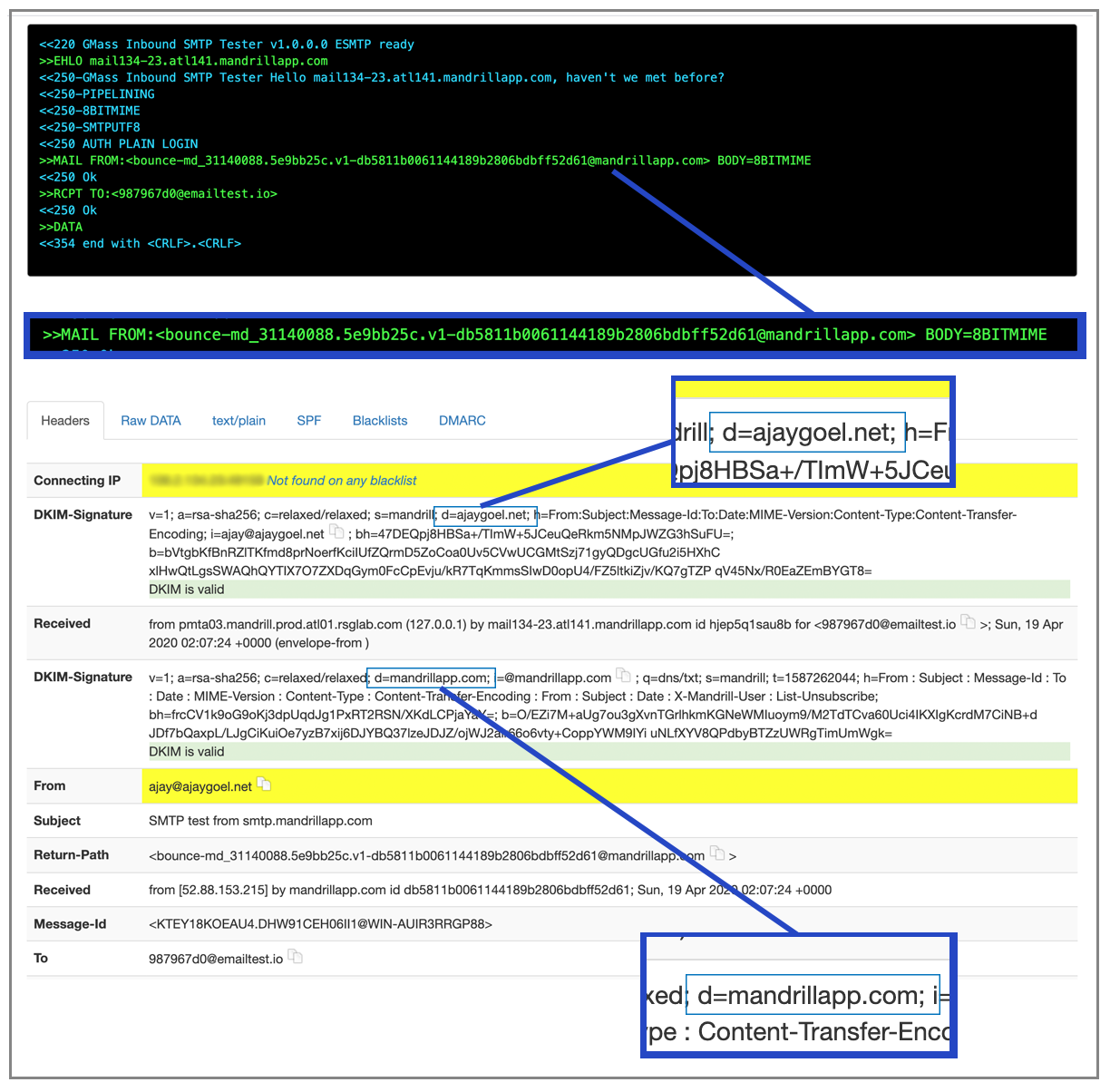
Here’s what I noticed though. The MAIL-FROM is still <bounce-md_31140088.5e9bb25c.v1-db5811b0061144189b2806bdbff52d61@mandrillapp.com and NOT @ajaygoel.net, so what was the point of having me set up SPF?
The email includes TWO DKIM signatures, one for my domain, ajaygoel.net, and another for their domain, mandrilapp.com. That’s curious, because it means they’re not AFRAID to sign your emails for you and risk their own domain’s reputation, so why make you sign emails with your domain in the first place? I’m going to have to ask support this.
Looks like you have to set up a “tracking domain” to get the MAIL-FROM to be at your domain. Weird, because we call a “tracking domain” something else.
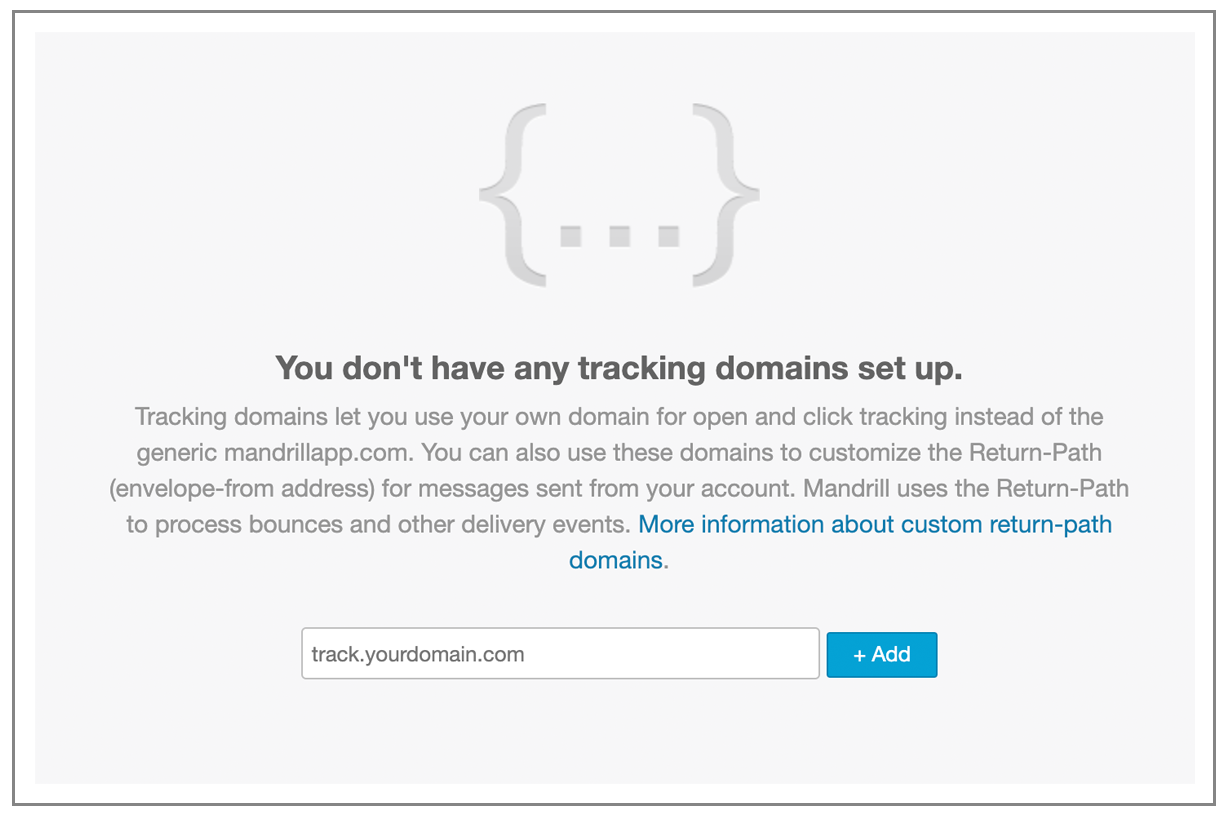
Well, this doesn’t work for me:
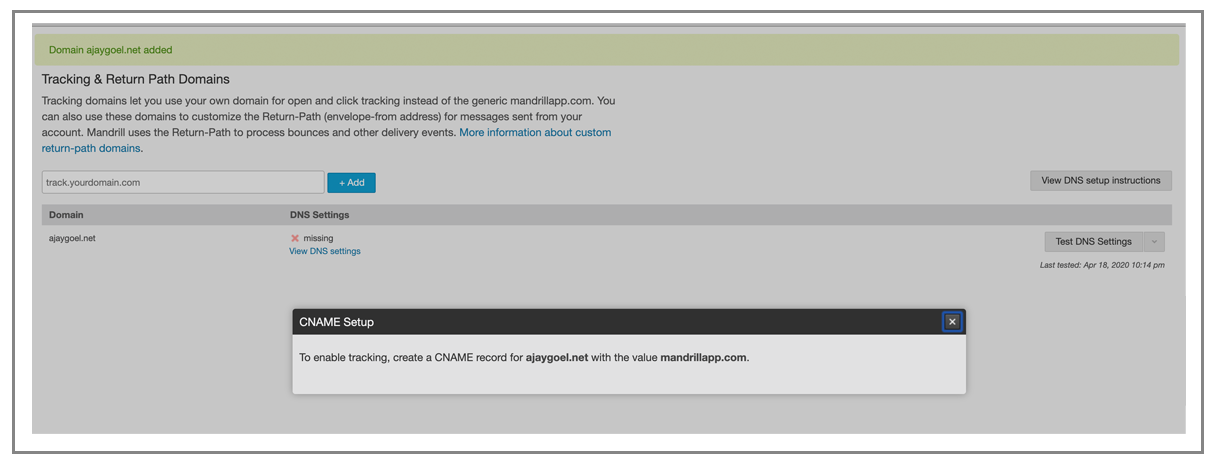
I’m not willing to alias my entire domain to you. This would be more palatable for a subdomain like transactional.ajaygoel.net, but if I want my main domain to be the MAIL-FROM, I can’t do that easily with Mandrill.
Abort, abort. I’ve already verified ownership of the domain, so at this point, it should just let me set it to be the MAIL-FROM. I understand that it wants to route bounces sent to “ajaygoel.net” back to Mandrill, but the fact is that most SMTP level bounces will still be captured by Mandrill during the SMTP conversation.
Even though I’ve now set up ajaygoel.net fully, I still can’t send from an @gmail.com account, for the same reason I initially couldn’t send from [email protected]. Mandrill considers it “unsigned” and obviously I can’t go into gmail.com’s DNS record and add a little ol’ TXT record.

The skinny on Mandrill
Unless you’re already a MailChimp user and in love with the service, Mandrill probably isn’t the best SMTP service to use. It’s difficult to to sign up, it requires a lengthy setup procedure to send, the domain verification process is overkill, and you can’t send “from” your @gmail.com address. The UI though is pretty nice. You might remember that Mandrill stirred quite a controversy a few years ago when it started charging for its service separately, prompting competitors like SendGrid to even set up a “Switch away from Mandrill” hotline to steal their customers. I doubt Ben Chestnut, the CEO, was bothered by this.
Grade: C-
Mailgun
(back to top)
I found Mailgun annoying right from the start. You have to create a domain to send anything, similar to Mandrill. They do have a sandbox domain set up, but you can only send to “authorized recipients”. Mailgun and Amazon SES are the two SMTP services that even have the concept of “authorized recipients”.
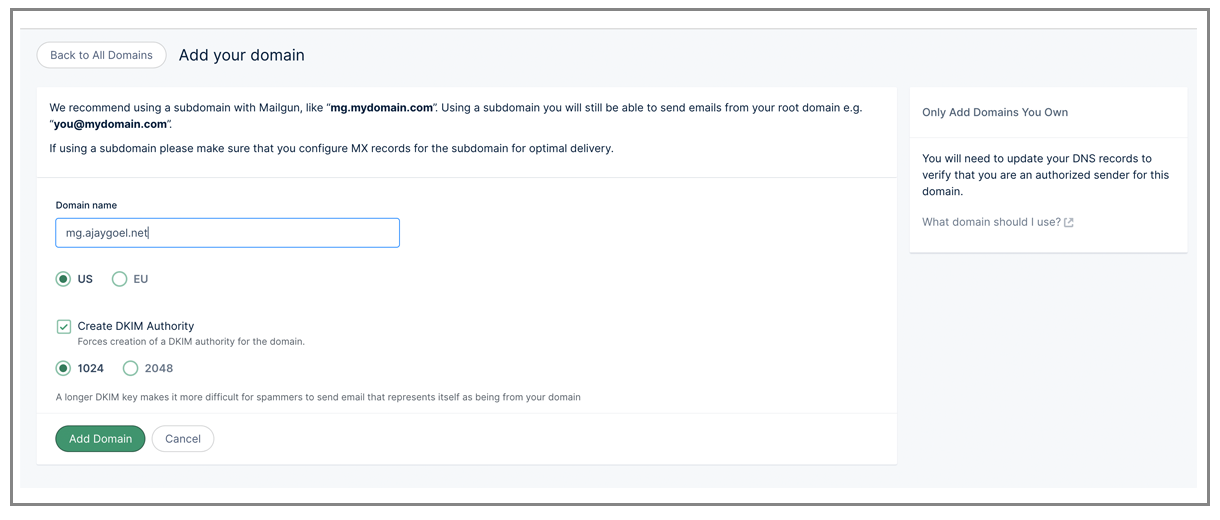
When you add a domain, it encourages you to set up a sub-domain like “mg” of your actual domain. So let’s go through the set up of mg.ajaygoel.net.
These are the DNS records it wants me to add:
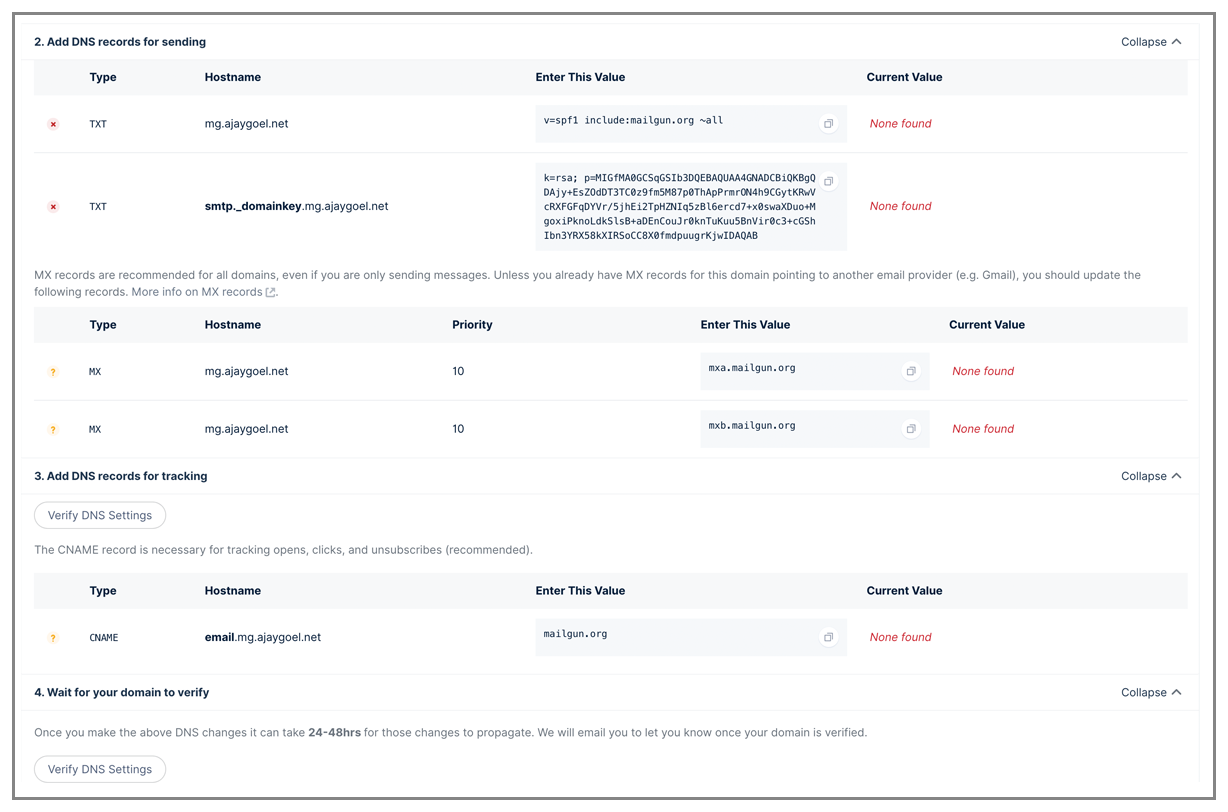
Lots of UI issues
The UI is abysmal. After clicking on my sandbox domain, I go to copy/paste my username/password into my code that relays emails through the SMTP server. Notice a problem?
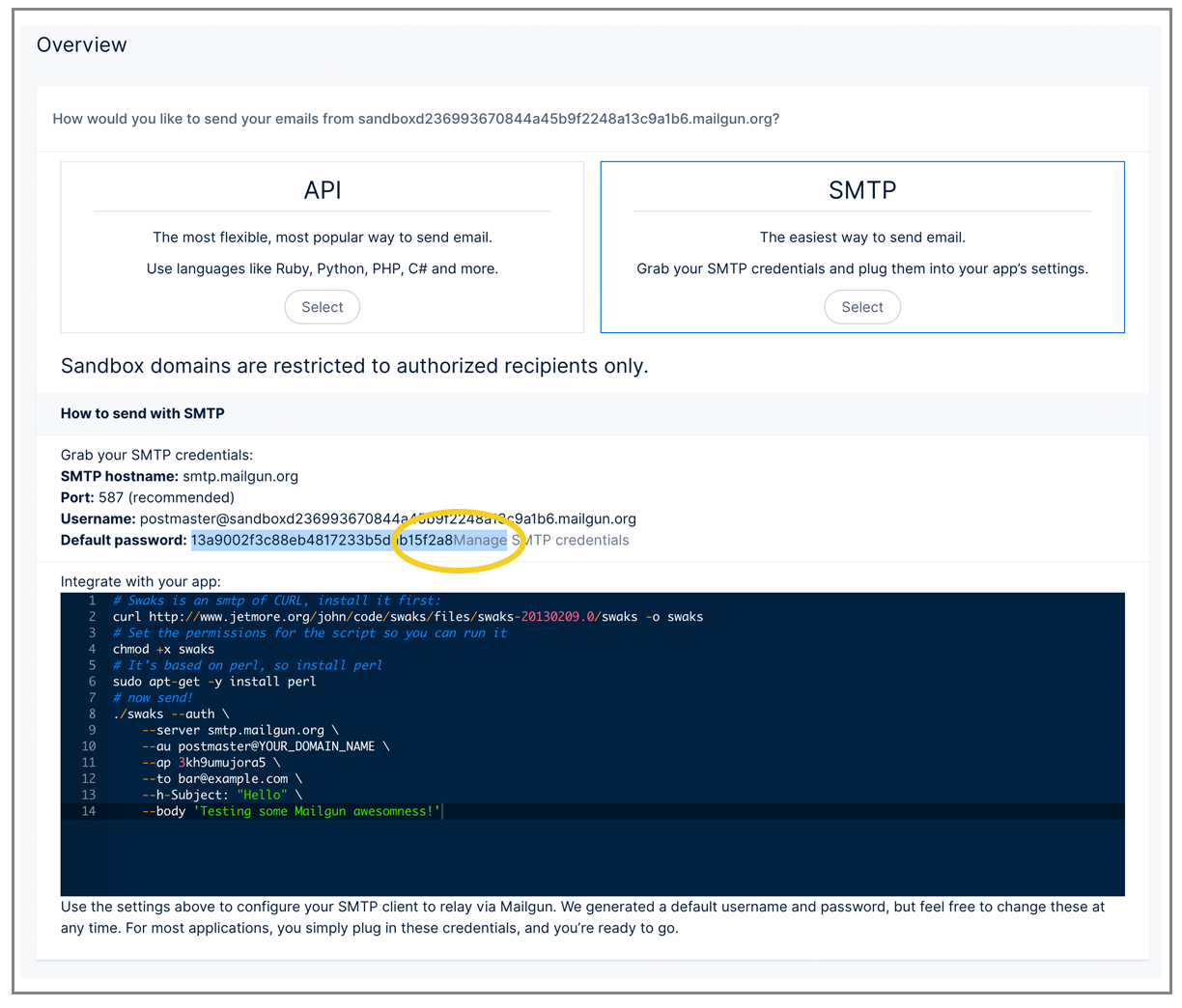
There’s no space between the password and a link to the right, so when I click to highlight it, it highlights extra text. Sloppy sloppy.
Here’s a weird UI quirk:
Basically, Mailgun doesn’t let you use its own domain in the MAIL-FROM or DKIM signature, transferring all the domain reputation responsibility to me, the customer.
Another weird UI quirk:
Another weird UI thing. It’s hard to get to your actual SMTP credentials, unlike in mailjet. here you have to go to yoru domain, go to “domain settings”, and then you’ll find it. SMTP settings should be a main nav item
Why is the green X in the middle?
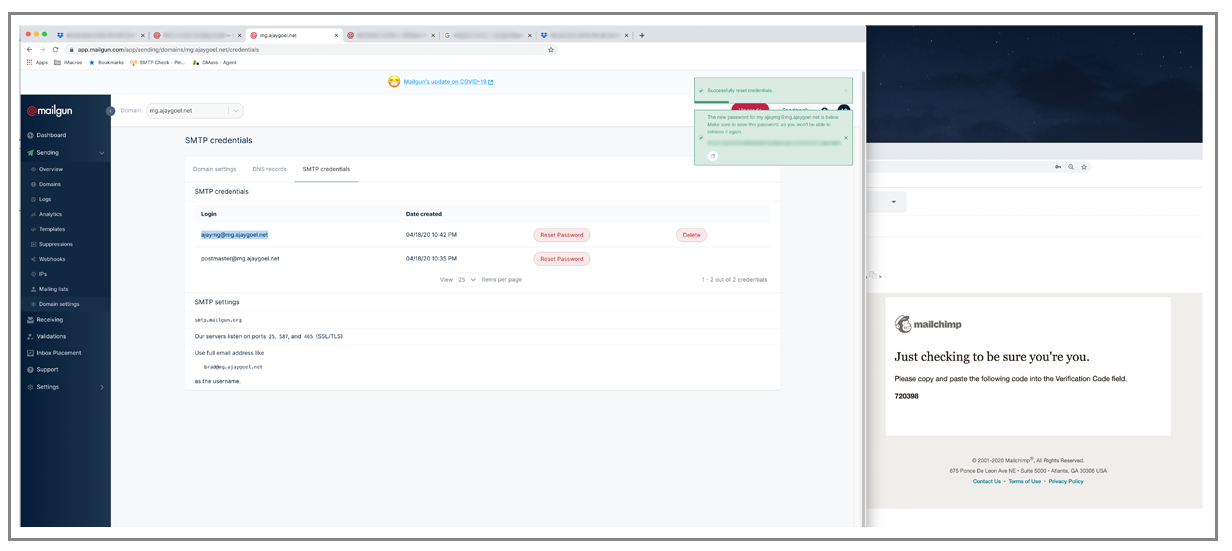
Sending
Using my sandbox credentials to test sending an email doesn’t get me very far:
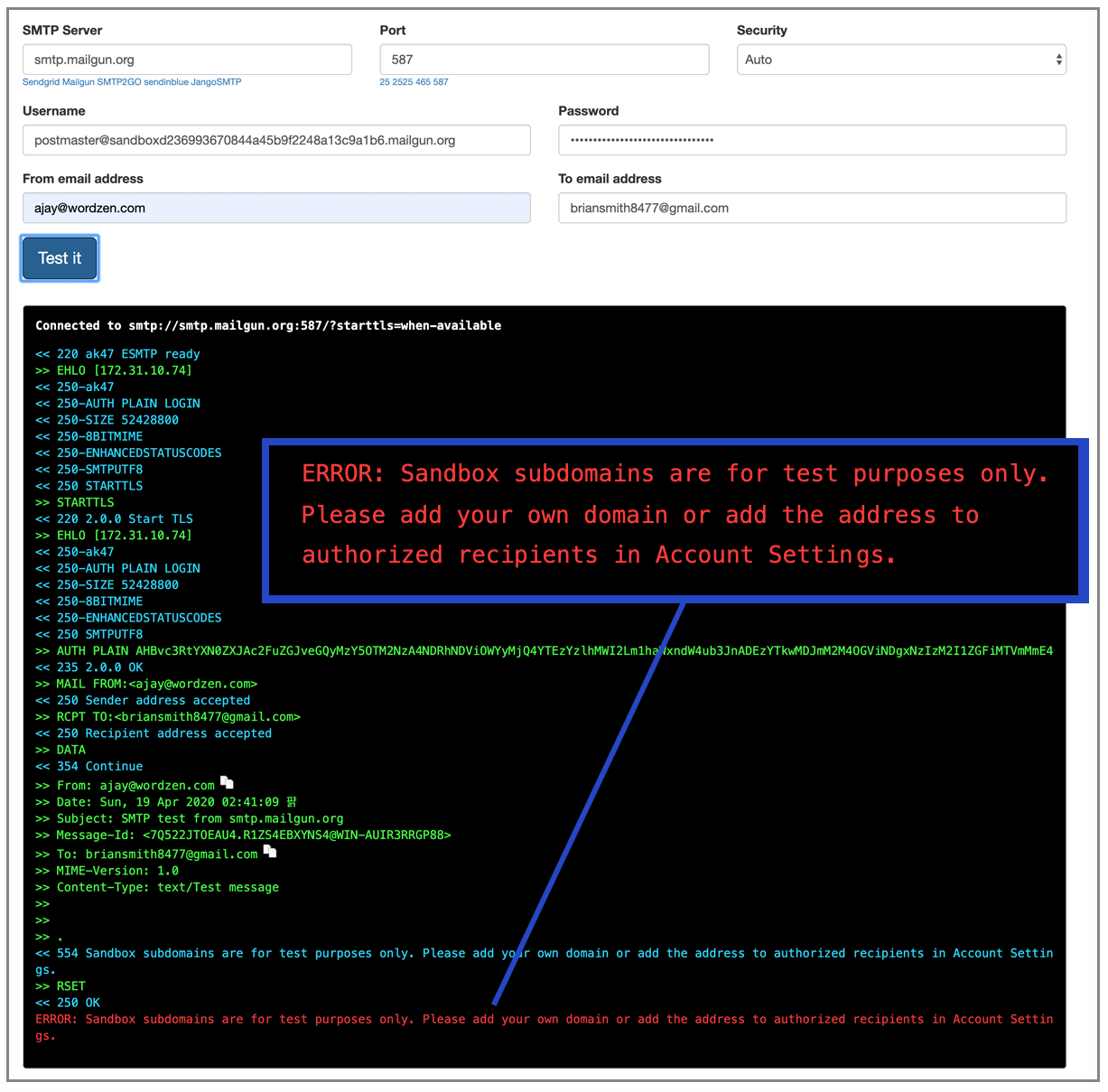
Just like SendInBlue, I’m told my sending limit is 100 emails/hour. At least that’s a nice round number, compared to SendInBlue’s 78 emails/hour restriction:
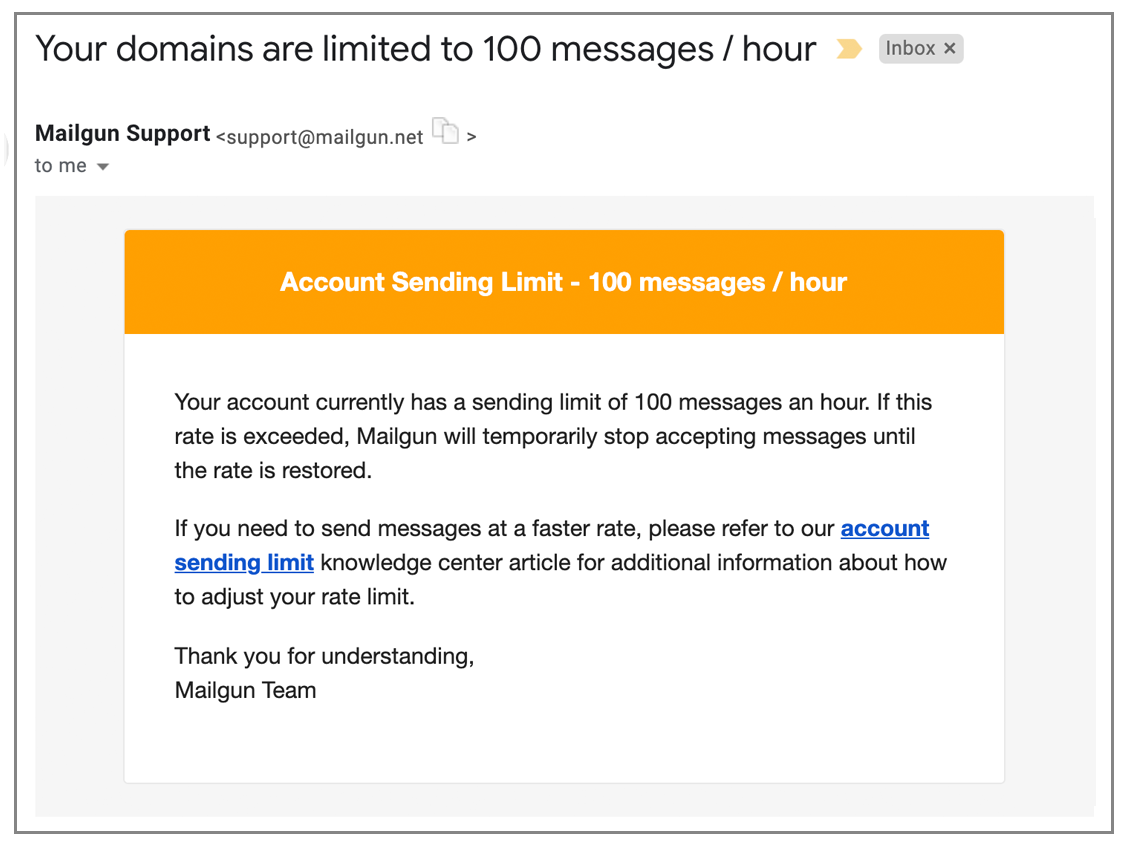
This is how Mailgun sends the email:
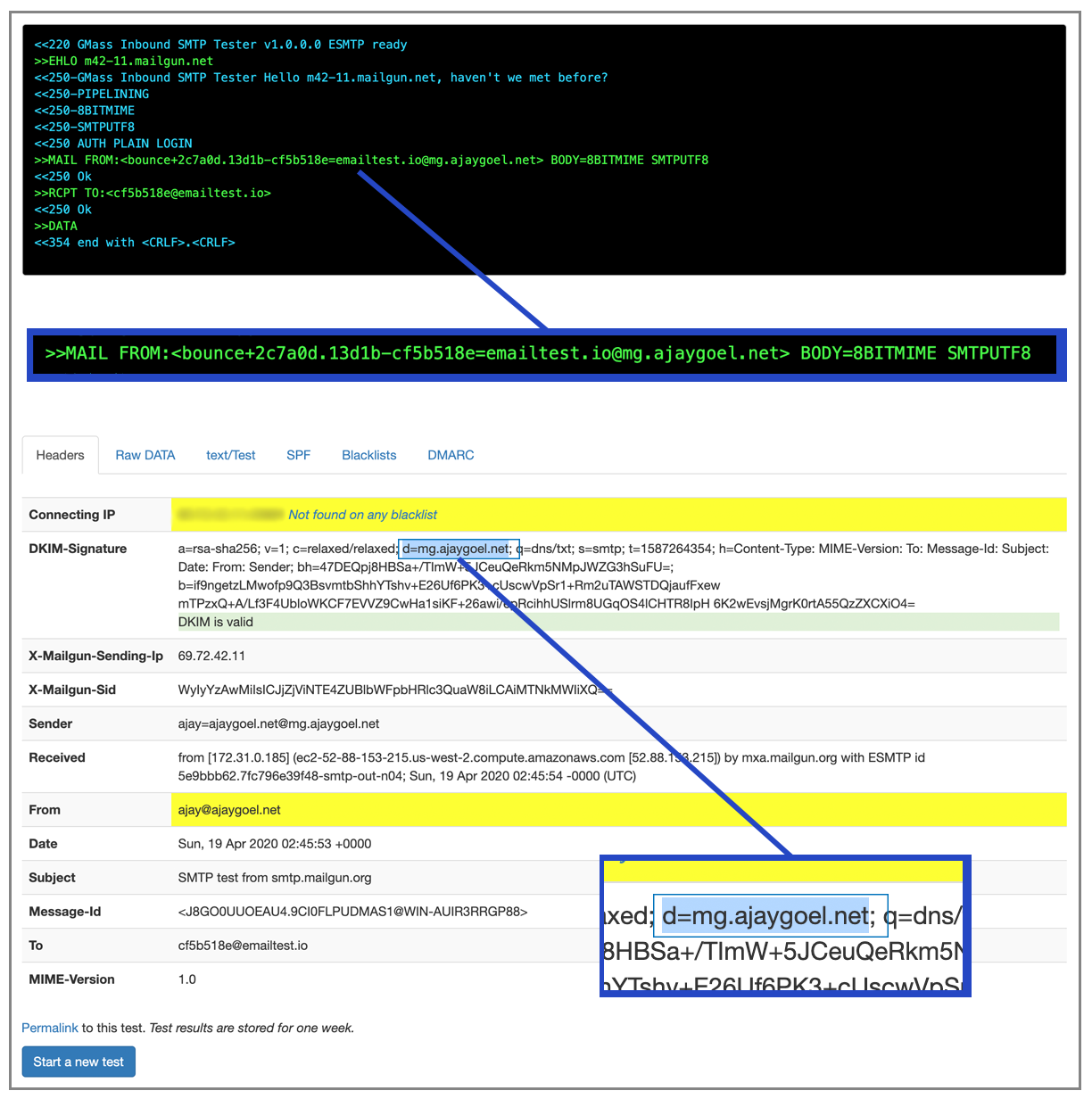
The MAIL-FROM is based on @mg.ajaygoel.net. The DKIM sig is signed using mg.ajaygoel.net. That’s appropriate since it made me set up SPF and DKIM DNS records for it.
Another odd quirk is that SMTP credentials are broken down by your domain. So for each domain you set up, you get a different set of SMTP credentials. I don’t understand why one username/password can’t be used for the whole account and every domain set up inside it. The organization by “domains” with Mailgun is almost as strange as SocketLabs’ organization by “server”.
Makes you set up your own domain, and correctly it recommends that you set up a sub-domain, because this is how it will probably DKIM sign and what it will put in mail-from header
The one piece of good news is that now that we have our domain set up, which is used for the MAIL-FROM and DKIM, we can actually send “from” anybody to anybody.
Here I use the mg.wordzen.com domain to send from [email protected] even though I haven’t verified silicomm.com at all.
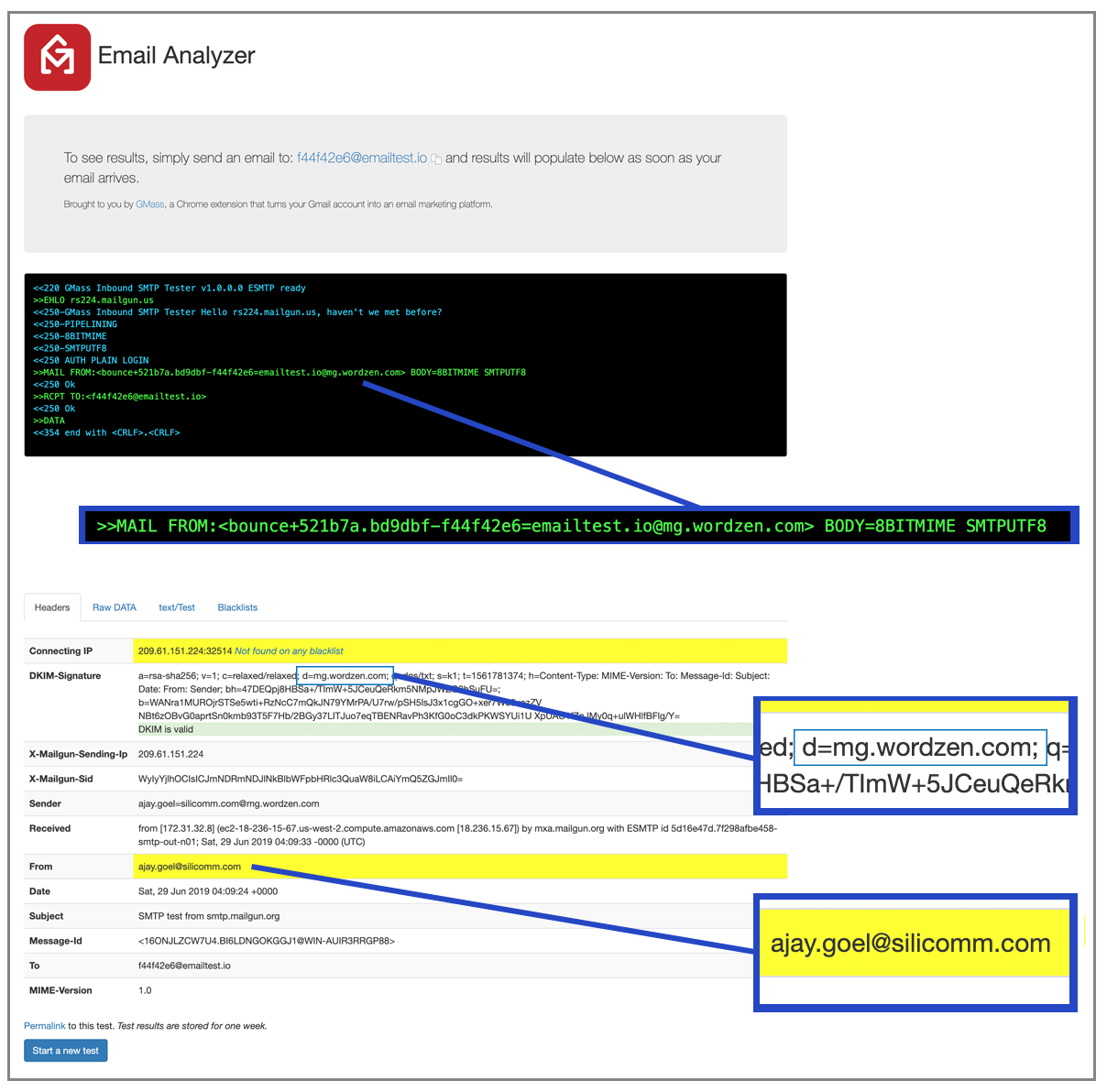
One good thing, I’m able to send “from” [email protected] without having set up silicomm.com as a domain. It just signs it the domain I did set up, ajaygoel.net.
Hopefully I’ve made it clear by now that an email can be signed by any domain, and doesn’t necessarily have to be the domain in the “from” or MAIL-FROM line. A DMARC policy, however, can change this.
Sending “from” silicomm.com:
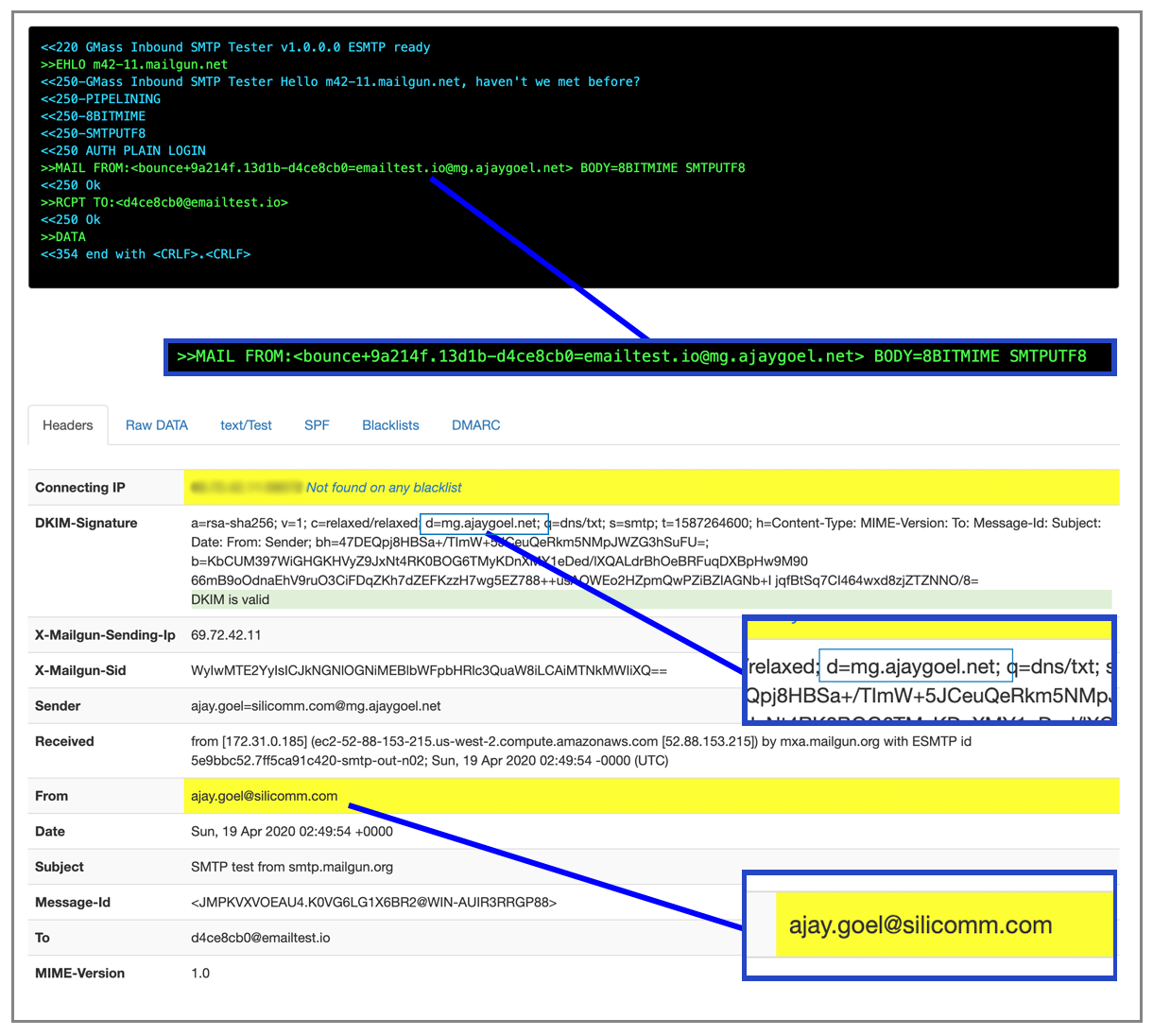
And it allows me to send “from” [email protected] too
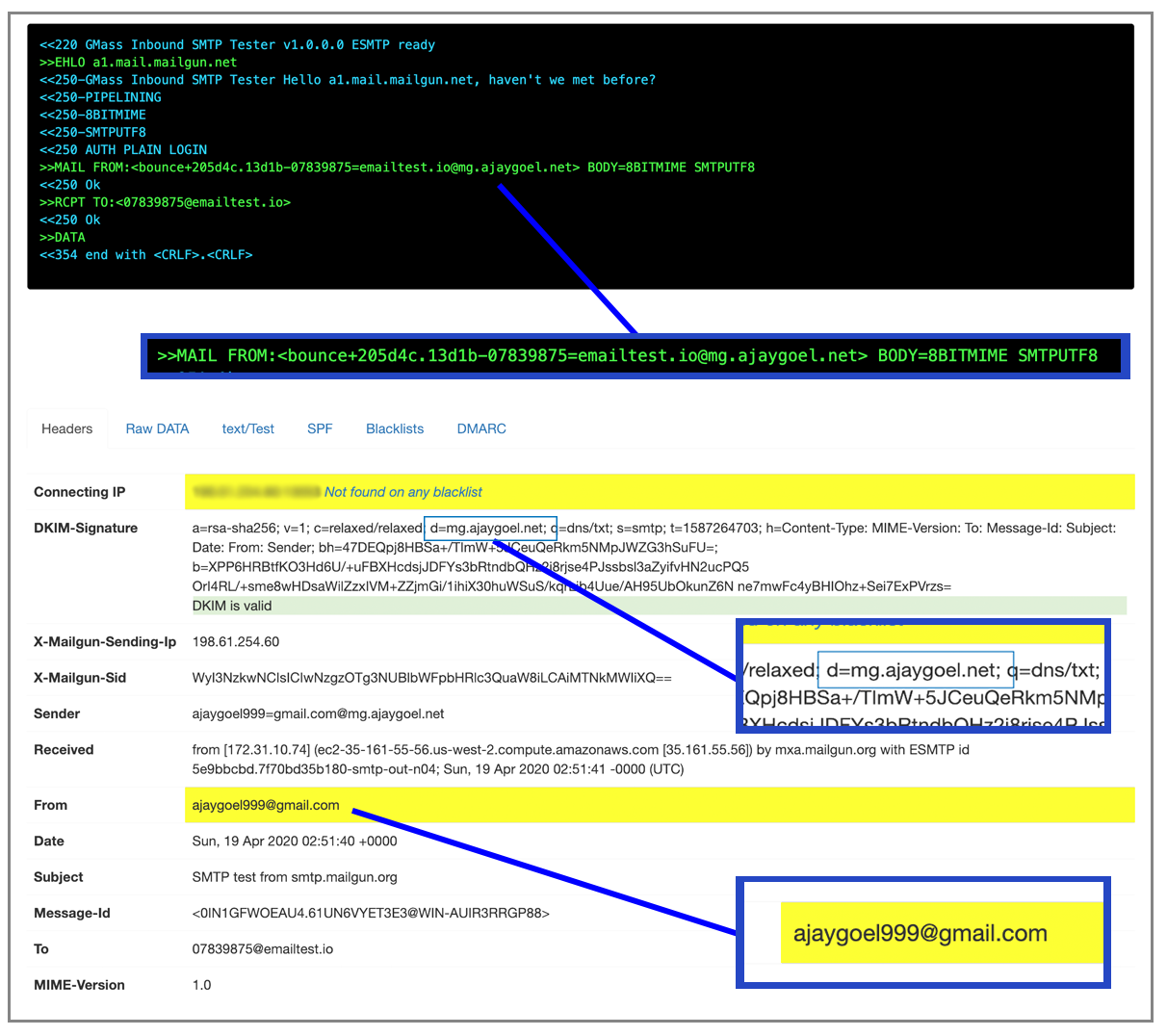
Here are their instructions to lift that 100 email/hour quota:
https://help.mailgun.com/hc/en-us/articles/115001124753-Account-Sending-Limitation
So they employ manual human review, because their automated systems aren’t smart enough?
The skinny on Mailgun
The worst UI in the business. You have to upgrade to be able to send OUTSIDE of authorized recipients, so really no testing to your real list for free.
You have to set up a domain for SPF and DKIM signing before you can send any real emails.. Mailgun doesn’t ever sign anything with its own domain or use its own domain even in the MAIL-FROM, so they’re entirely passing the buck to the user. The saving grace is that once you set up any domain, you can send “from” any address you want, including @gmail.com addresses.
Grade: C-
Read my non-technical Mailgun review.
SMTP2GO
(back to top)
Easy sign up, and I can send right away! My test email went to INBOX even with an SPF failure. Why did SPF fail out of the box? Because this is a pass-through SMTP service.
Meaning if I send an email “from” [email protected], then the MAIL-FROM when delivering the email is also set to [email protected].
Note that its pass-through in the MAIL-FROM but it does DKIM sign with its own domain, smtpcorp.com, so out of the box SPF will fail but DKIM will pass.
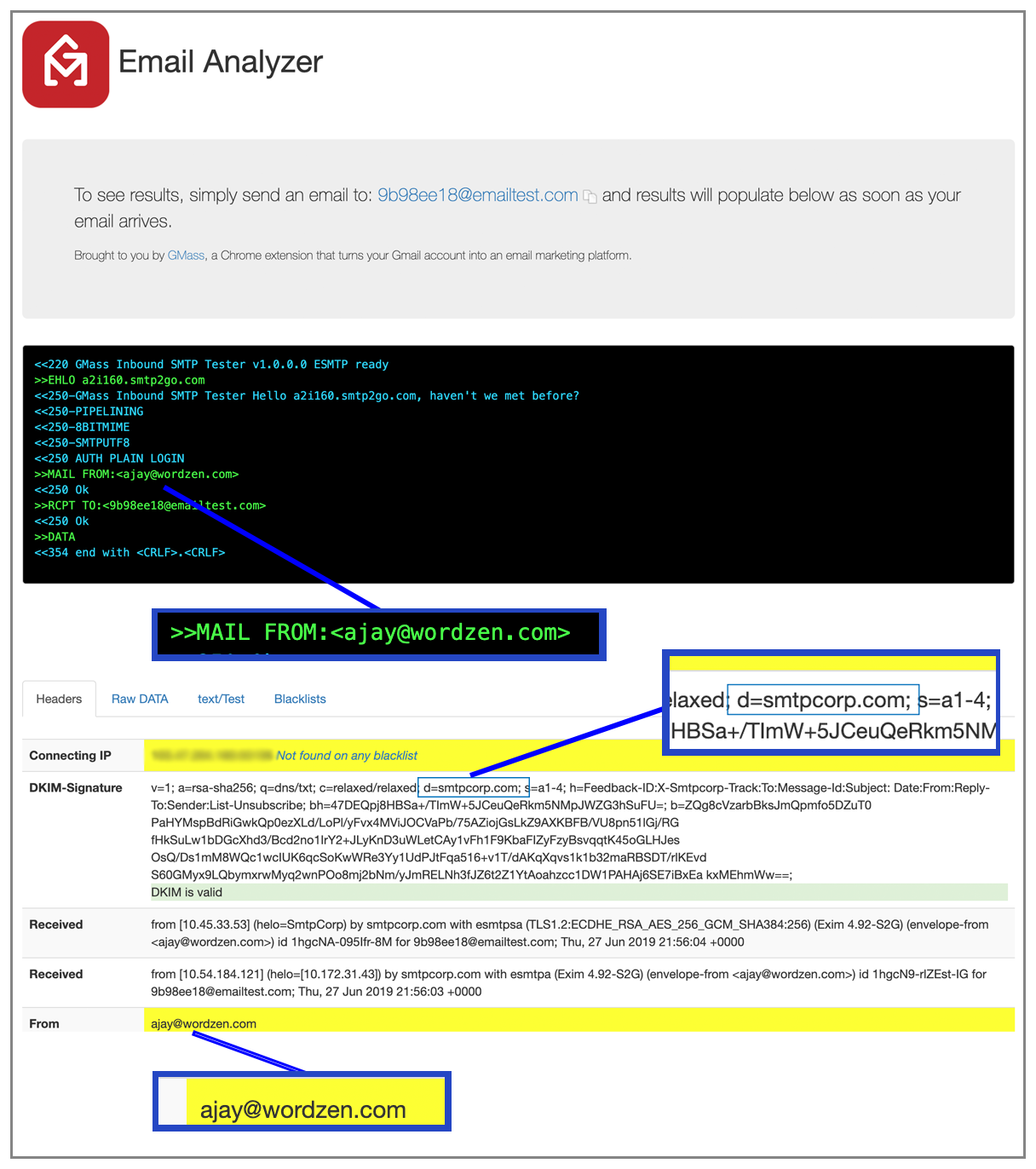
You can add a “sender domain”:
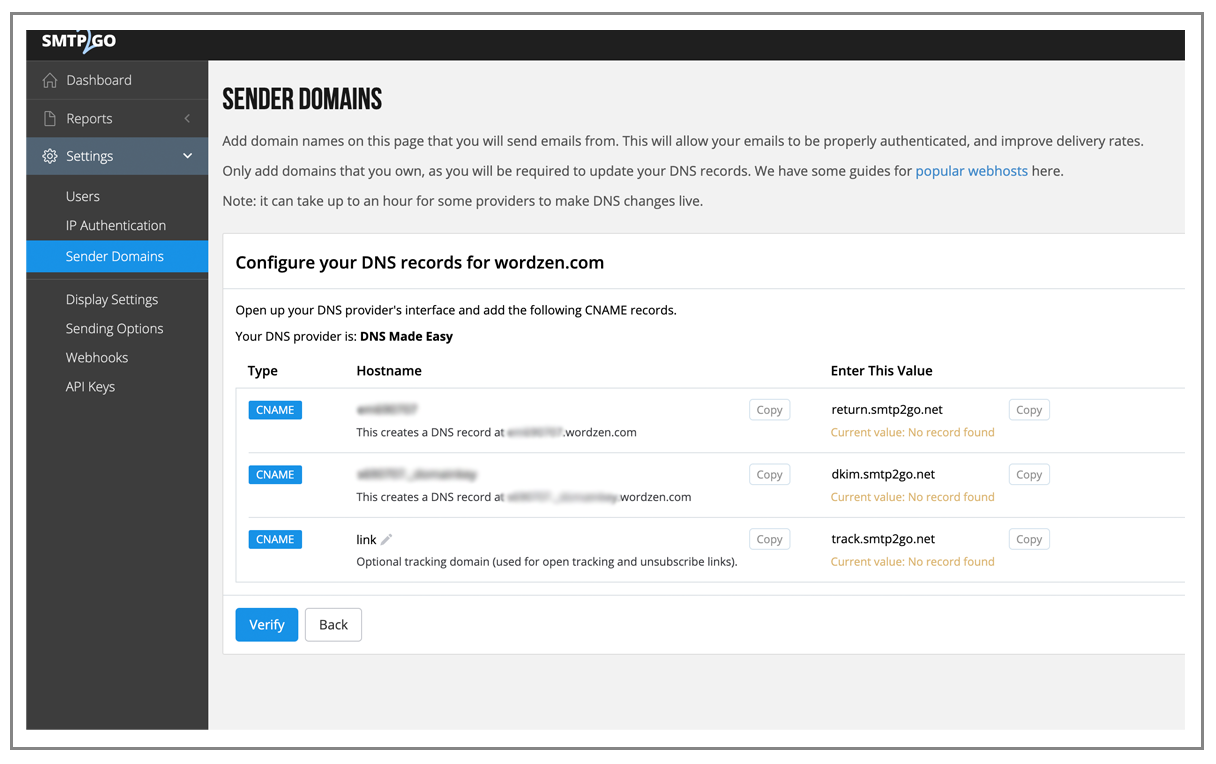
The docs say that this will then DKIM sign your emails based on your own domain, but what will the MAIL-FROM then be when it sends an email? TBD.
By default it does NOT restrict who you can send “from”:
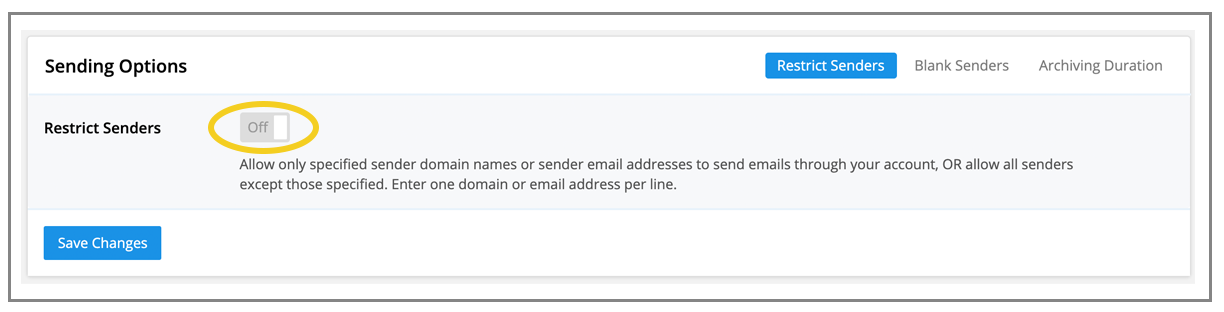
This is nice – the reporting shows all recipients and subject lines:
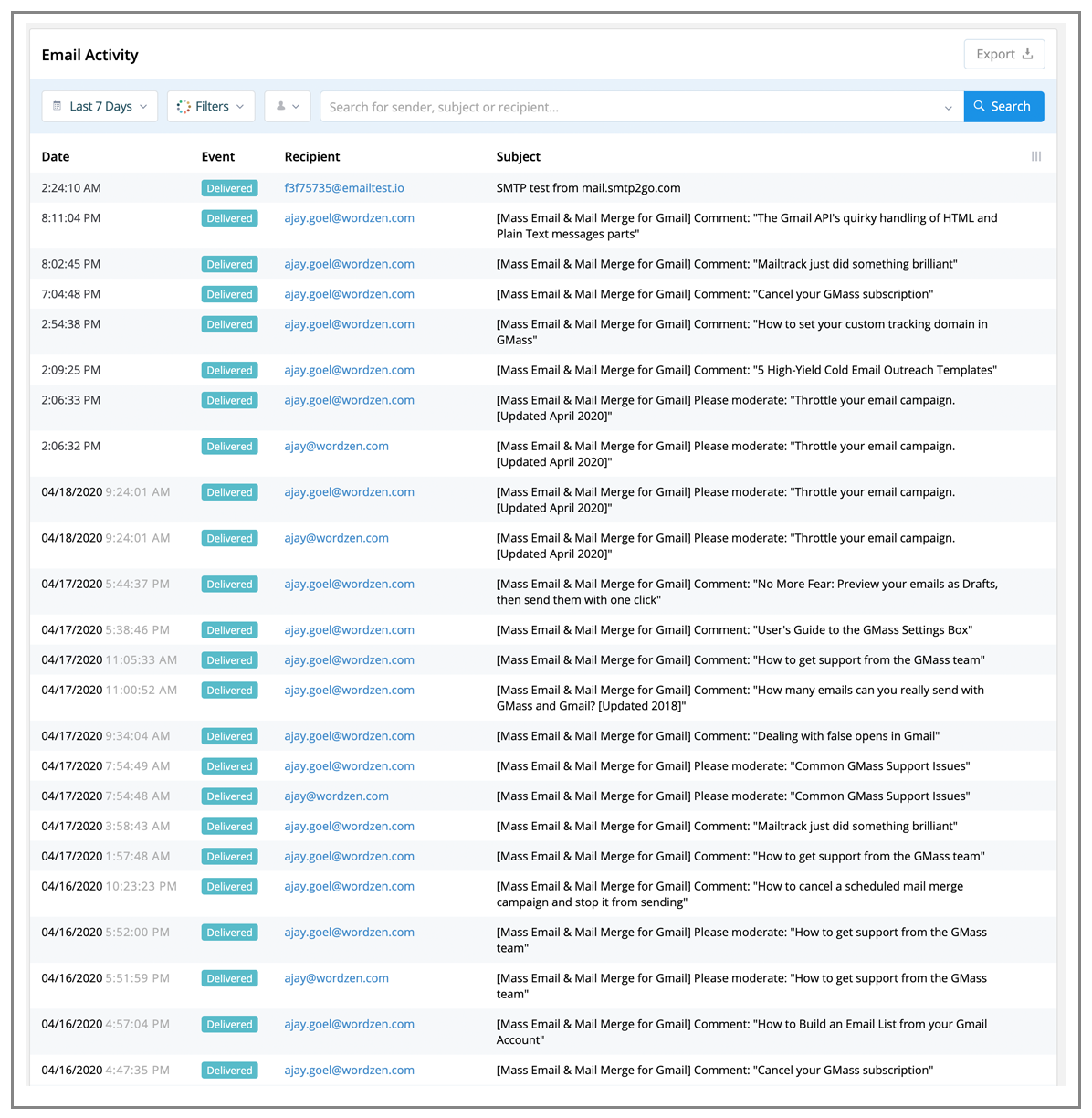
…although it would be nice if it included the “from” address too.
Because it’s a pass through provider by default, when you first send from [email protected], the email is actually sent like this:
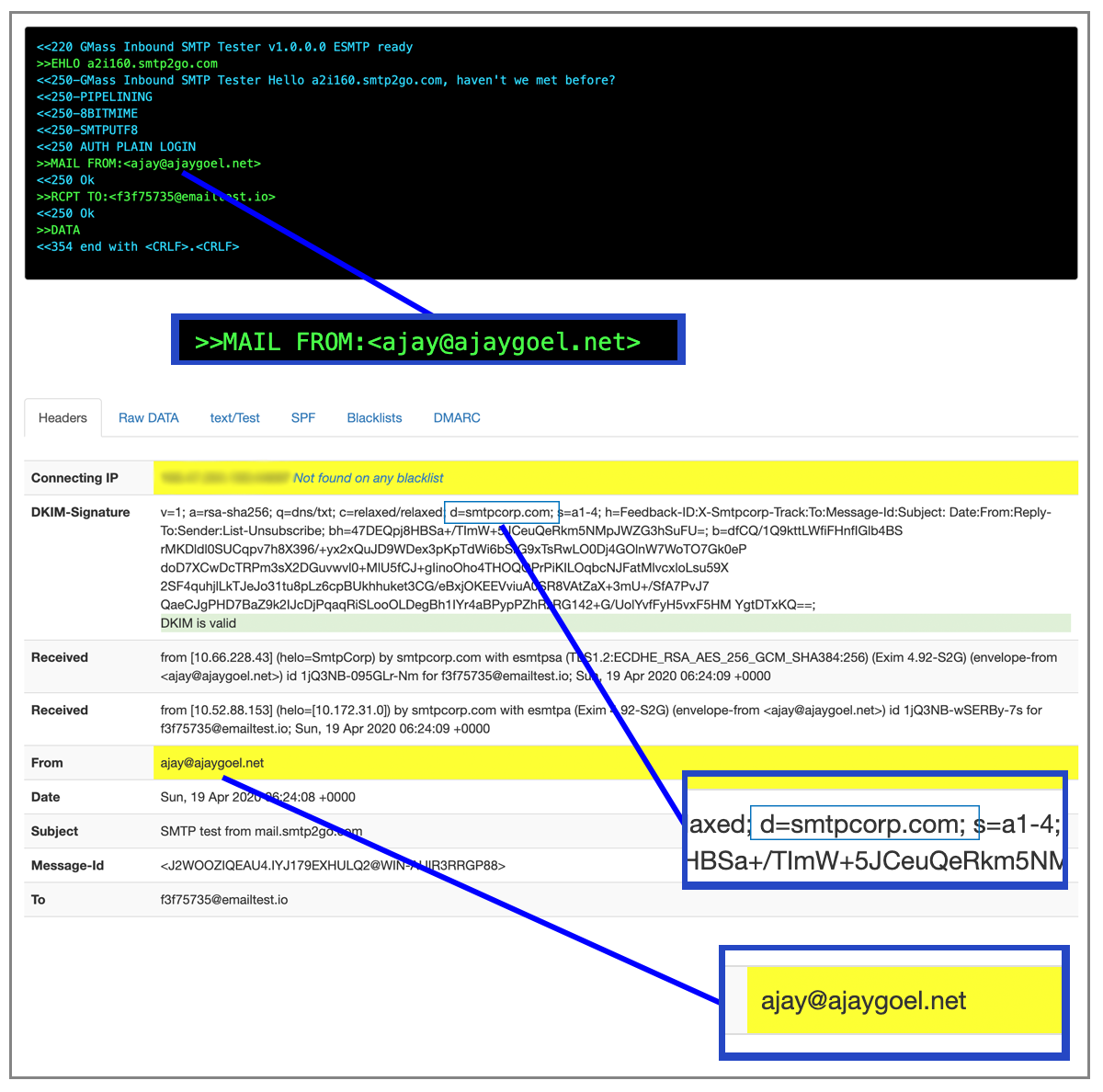
But in both cases, it does sign DKIM with its own domain, smtpcorp.com
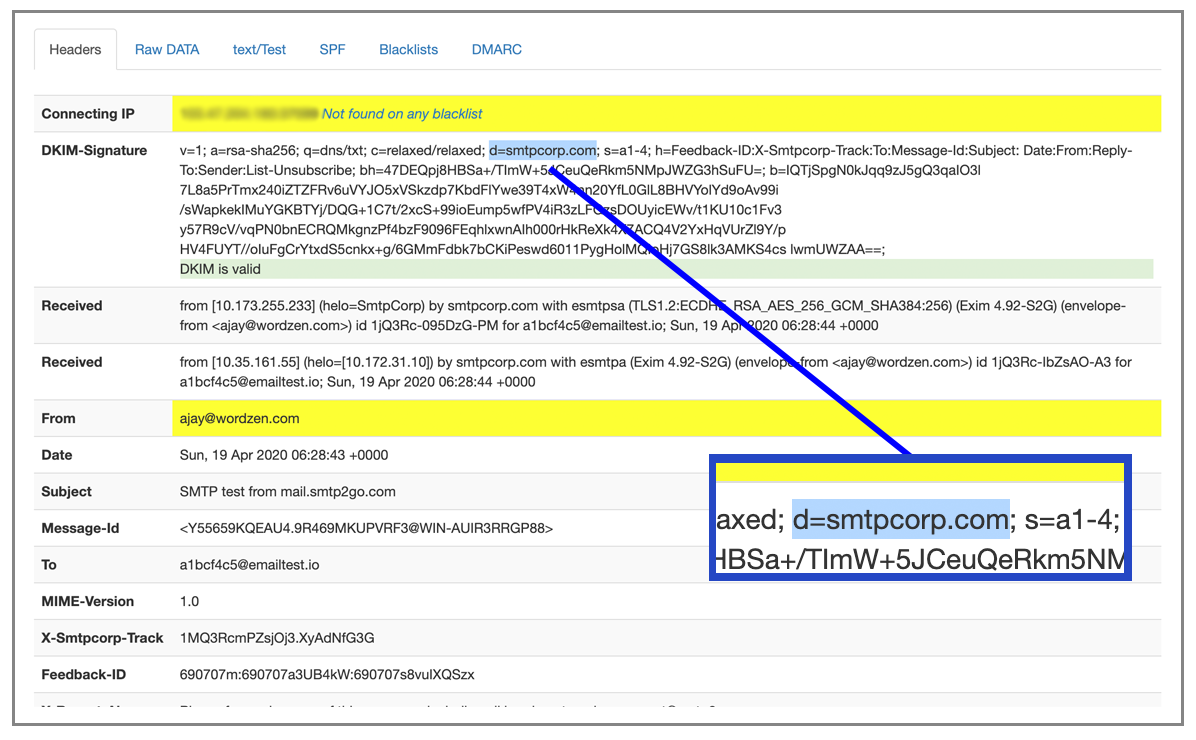
After setting up my domain, ajaygoel.net, this is what changes. The MAIL-FROM is now modified to be [email protected] instead of the raw [email protected], and DKIM signing now includes both smtpcorp.com AND my domain ajaygoel.net
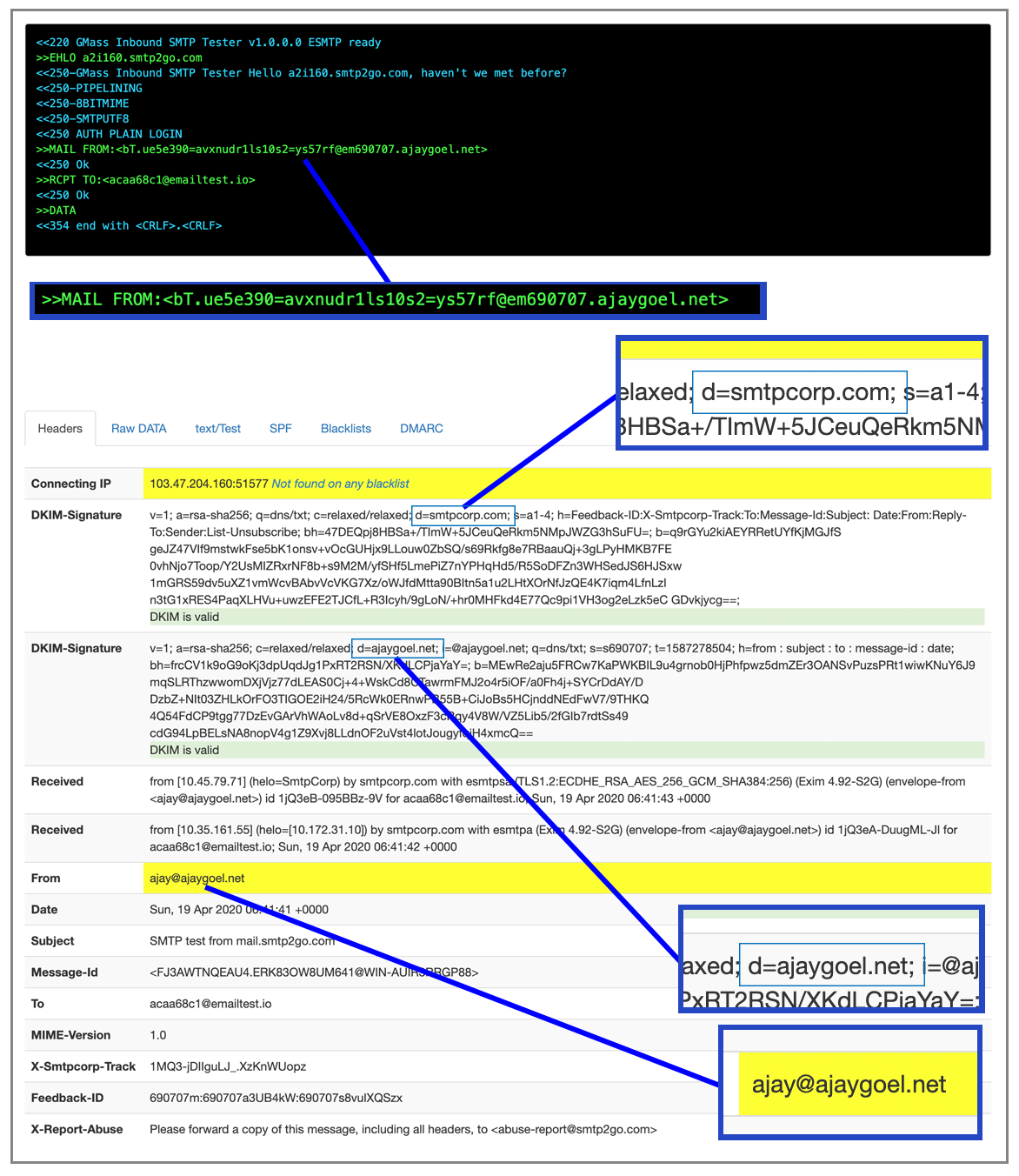
What’s the meaning of that weird MAIL-FROM? Well now it’s SPF compliant, and I didn’t have to modify my own SPF records, since they’re using the CNAME technique.
The CNAME records I entered:
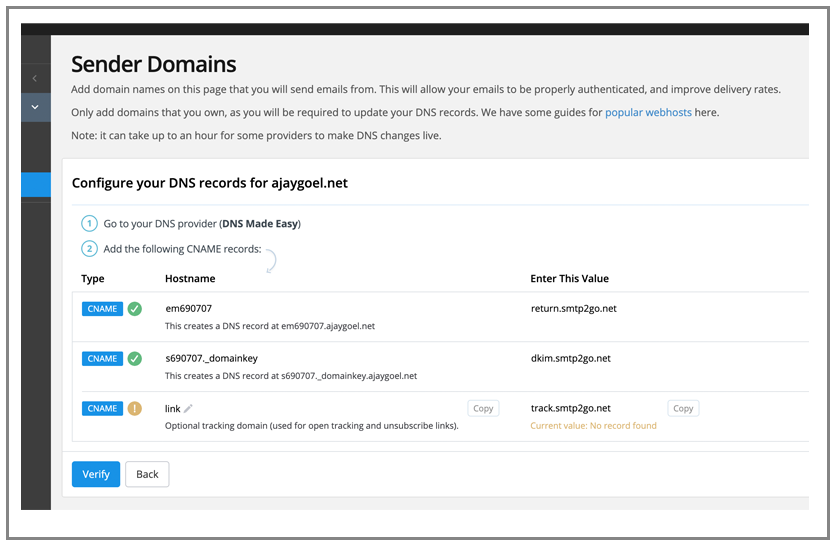
and eventually it verifies their presence. I’ve noticed that sometimes a provider will ask to set up CNAME records versus others will ask to set up TXT records. What’s the difference? By having me set up a CNAME record on a sub-domain, they can control the final result of the domain lookup, since the CNAME is just an alias to their domain. So if anything changes down the road, like their IP pool or their DKIM keys, they can make that change for me without asking me to update my own TXT records.
The skinny on SMTP2GO
An easy, clean service that lets you send out of the box without setting up a domain or verifying much. It’s the only pass-through service I’ve tested, which might mean that it’s normally meant for people who need a simple SMTP relay for their person to person email, like the kind they’re sending from their Outlook client or personal Gmail accounts. The service may not have been designed to send commercial transactional email, although their website and pricing seem to indicate they can handle high volume loads just fine. Even though SMTP2GO is a lesser known service amongst the ones I tested, it gets a high grade for being simple, offering the flexibility I need, and stating all over its website that it won’t throttle my email flow.
Grade: B
Read my non-technical SMTP2GO review.
SendGrid
(back to top)
SendGrid used to be my favorite SMTP and transactional email service, because you could sign up, and start sending from any address and to any address without verifying anything, and SendGrid would make sure your emaails were SPF and DKIM compliant by using a MAIL-FROM of @sendgrid.net and DKIM signing with @sendgrid.net also. That was a rare thing to be so open and flexible. This all changed though on April 6, 2020, when SendGrid started mandating the use of what they call “sender identities”, which is just their term for having to verify every address or “domain” you send “from”.
So basically, if you created your account before 4/6, you were grandfathered in and you don’t have to do this, although it seems that some accounts still required this. Luckily, I have a handful of SendGrid accounts created before 4/6 where this doesn’t apply.
So after April 6, out of the box, if you create a SendGrid account and attempt to relay right away, this is what happens. So out of the box, you are denied unless you create a sender identity:
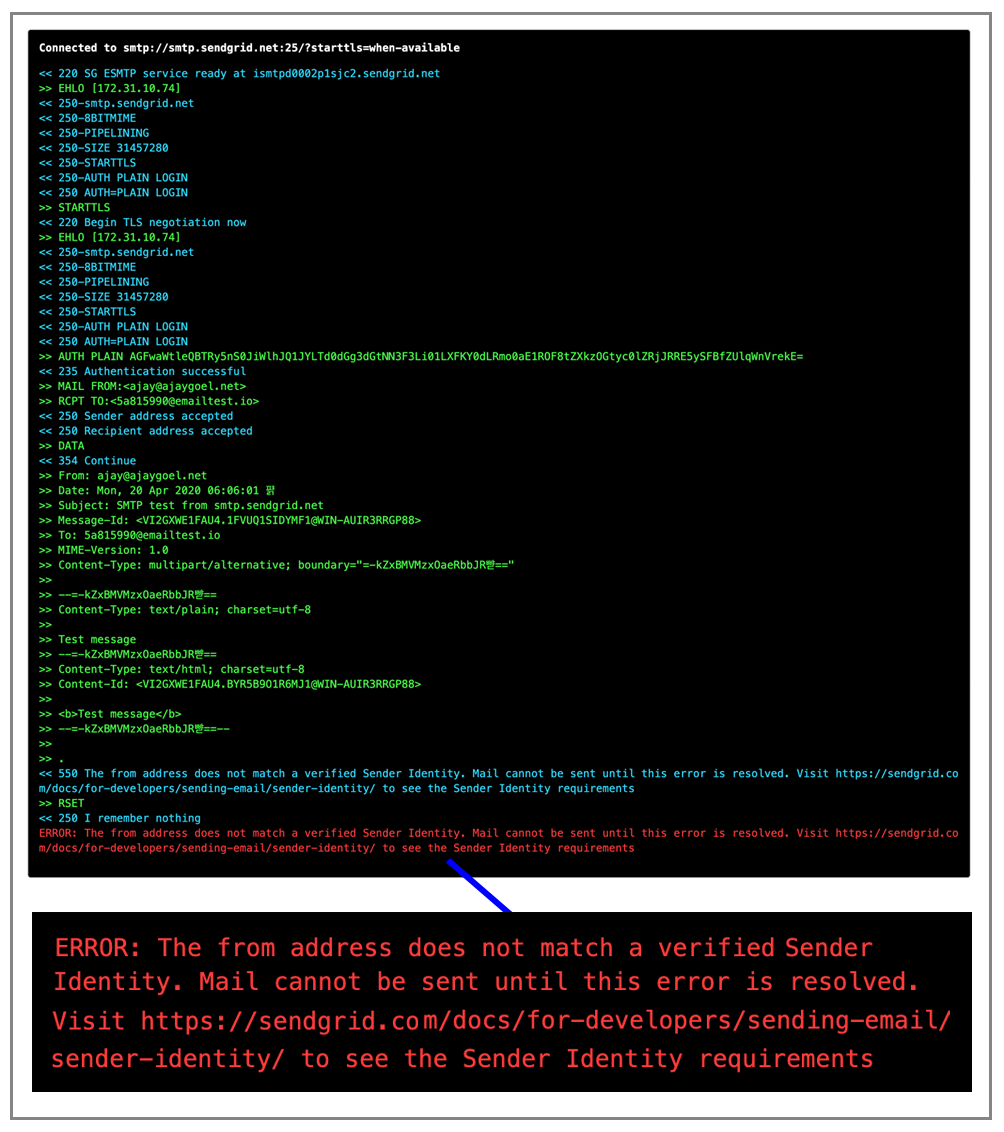
Silly elements of their UI
Their UI, while modern and slick, is designed in a silly fashion. Take the stuff under Settings. The items are listed alphabetically. That doesn’t do anybody any good, unless you know that for “Authentication” which is a typical section that an experienced emailer would seek in a new service, you should look under “S” because it’s actually “Sender Authentication”.
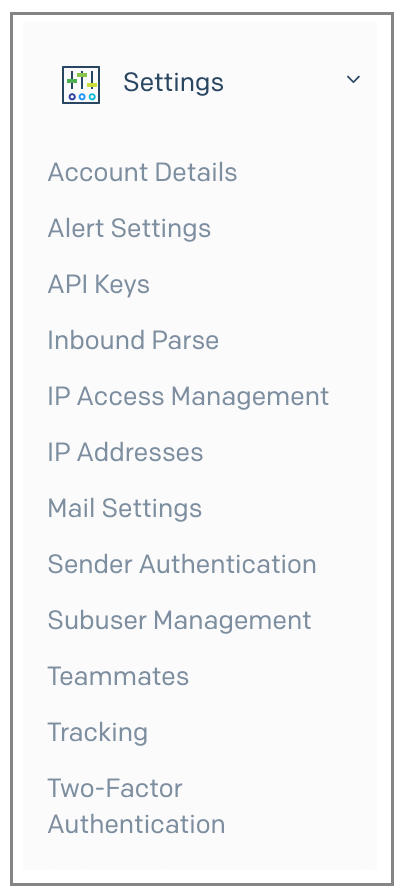
And, now that I’ve logged in, I immediately want my SMTP relay settings. Where on the left is it?
Send from an @gmail.com address?
Can you create a @gmail.com “sender identity”?
Yes, you can, but this exposes another element of bad UI design and bad back-end programming. Like Mailjet, the link to click to verify requires you to log in, if you’re not already. Why can’t intelligent web developers people generate verification links that contain a key to login?
Test Send
I verify [email protected] as a “sender identity” and my first email “from” [email protected] has been relayed but hasn’t arrived. Proof that I sent it:
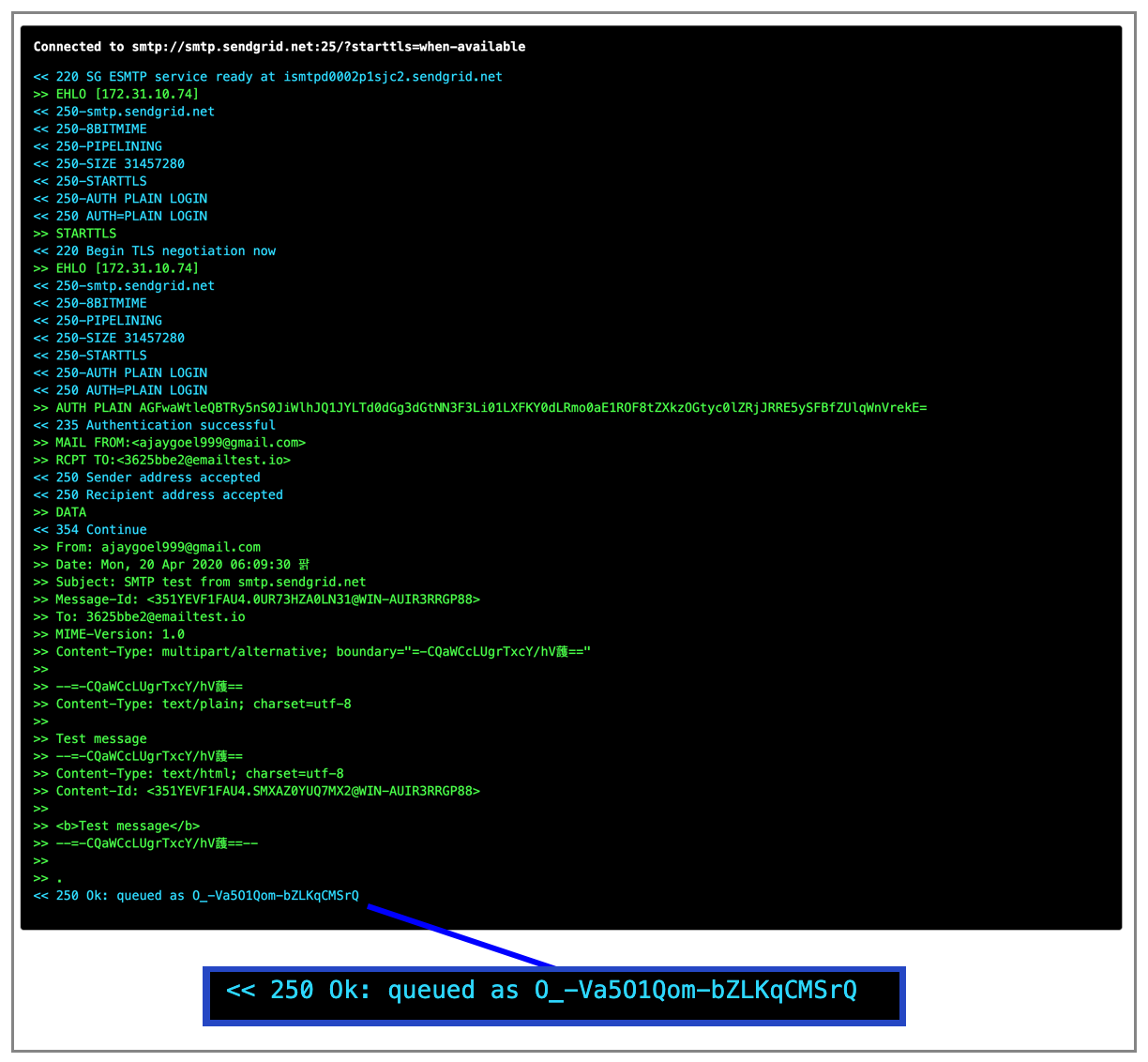
It shows up under Activity a few MINUTES later, and as “Processing”. Why isn’t a single email in a brand new account delivered right away?
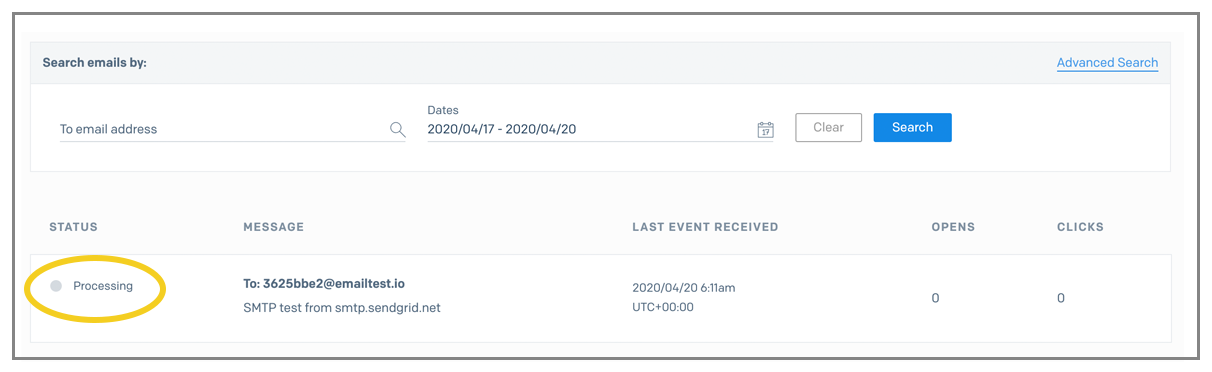
And finally, I see the SendGrid errors:
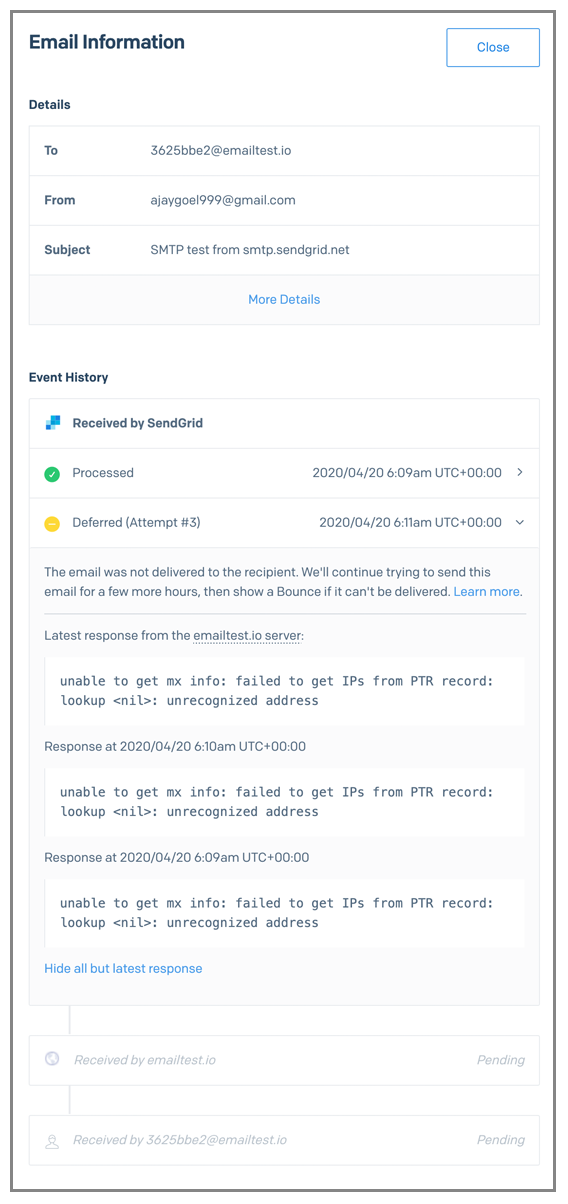
But these are the strangest errors and indicate a flaw in SendGrid’s processing. I happen to know that PTR records have NOTHING to do with email sending to a particular address. The only thing that is relevant is the MX records of the domain, and the MX records exist. See?
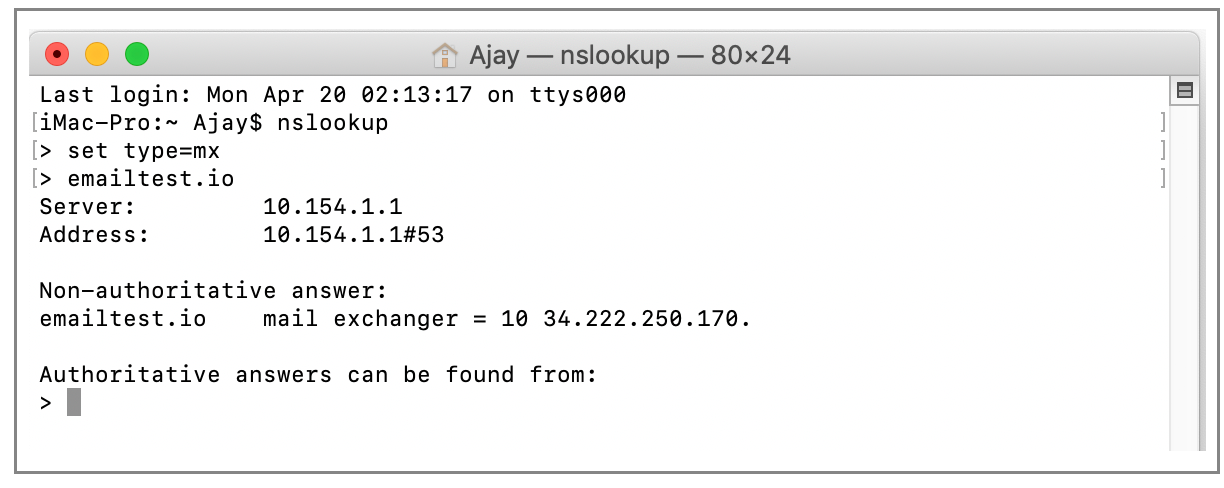
More proof from MXToolbox:
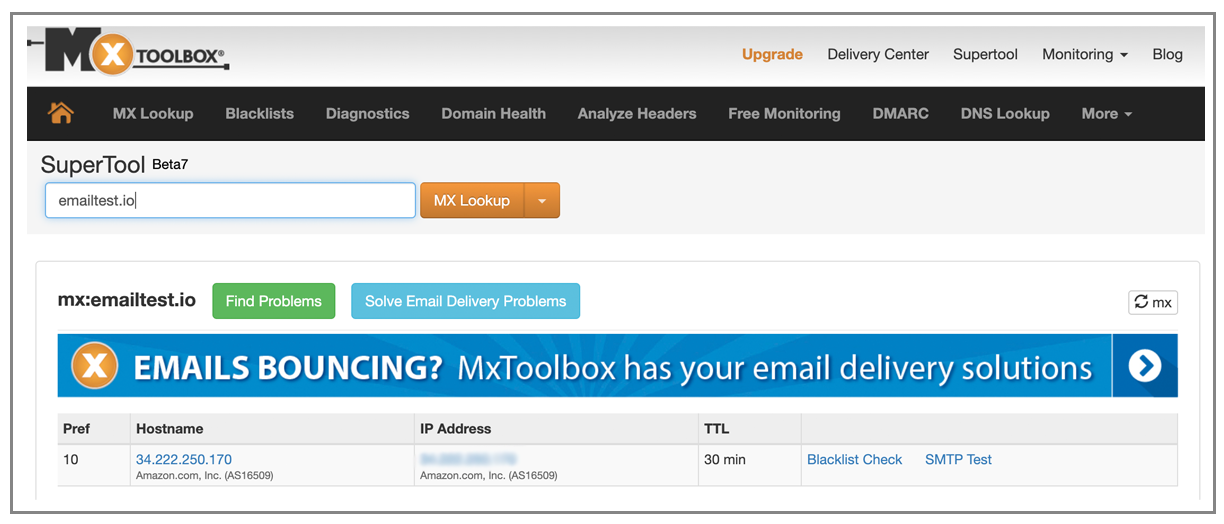
After its last try, it tells me that MY SERVER BLOCKED the email:
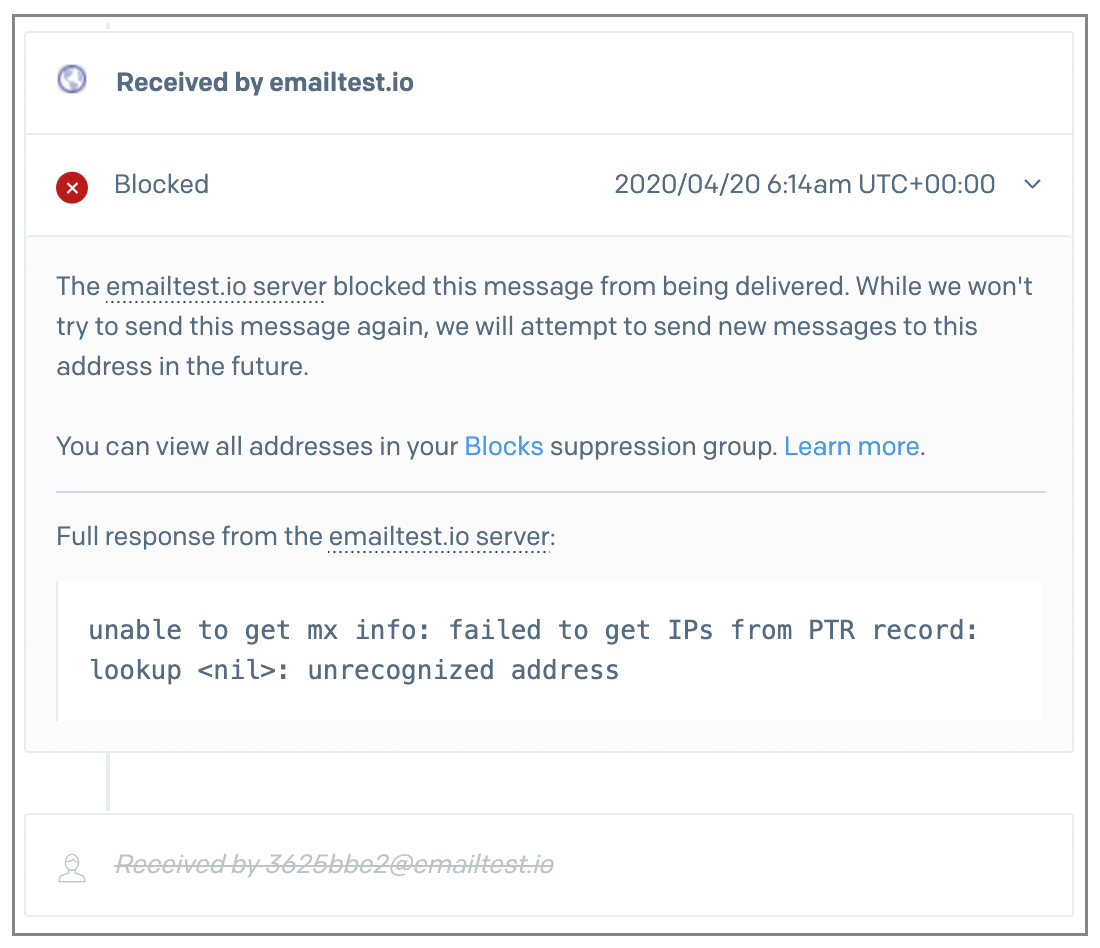
But we know that’s not true. Alright let’s just try sending to my @wordzen.com email address
Note that there’s a lag between the time an email is relayed and when it will appear in the UI under Activity Feed. For a company with the size and scale of SendGrid, I’m surprised this is the case. How long of a lag?
I relayed this email at 06:17
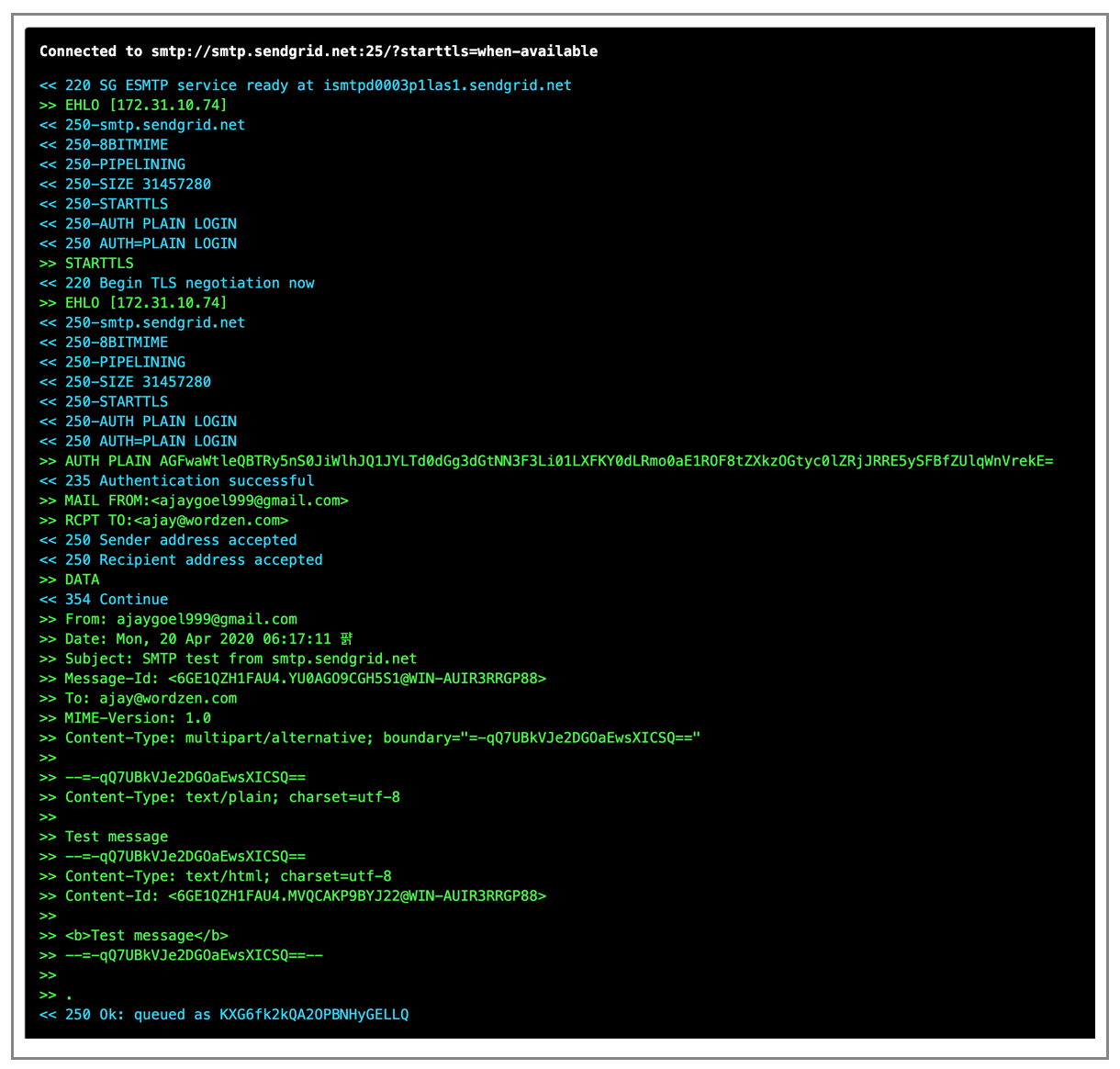
And it finally shows up in the UI not at 6:18, 19, or even 20, but at 06:21.
The email itself does arrive instantly though, and here’s what we notice. The MAIL-FROM domain and the domain for DKIM-signing is sendgrid.net:
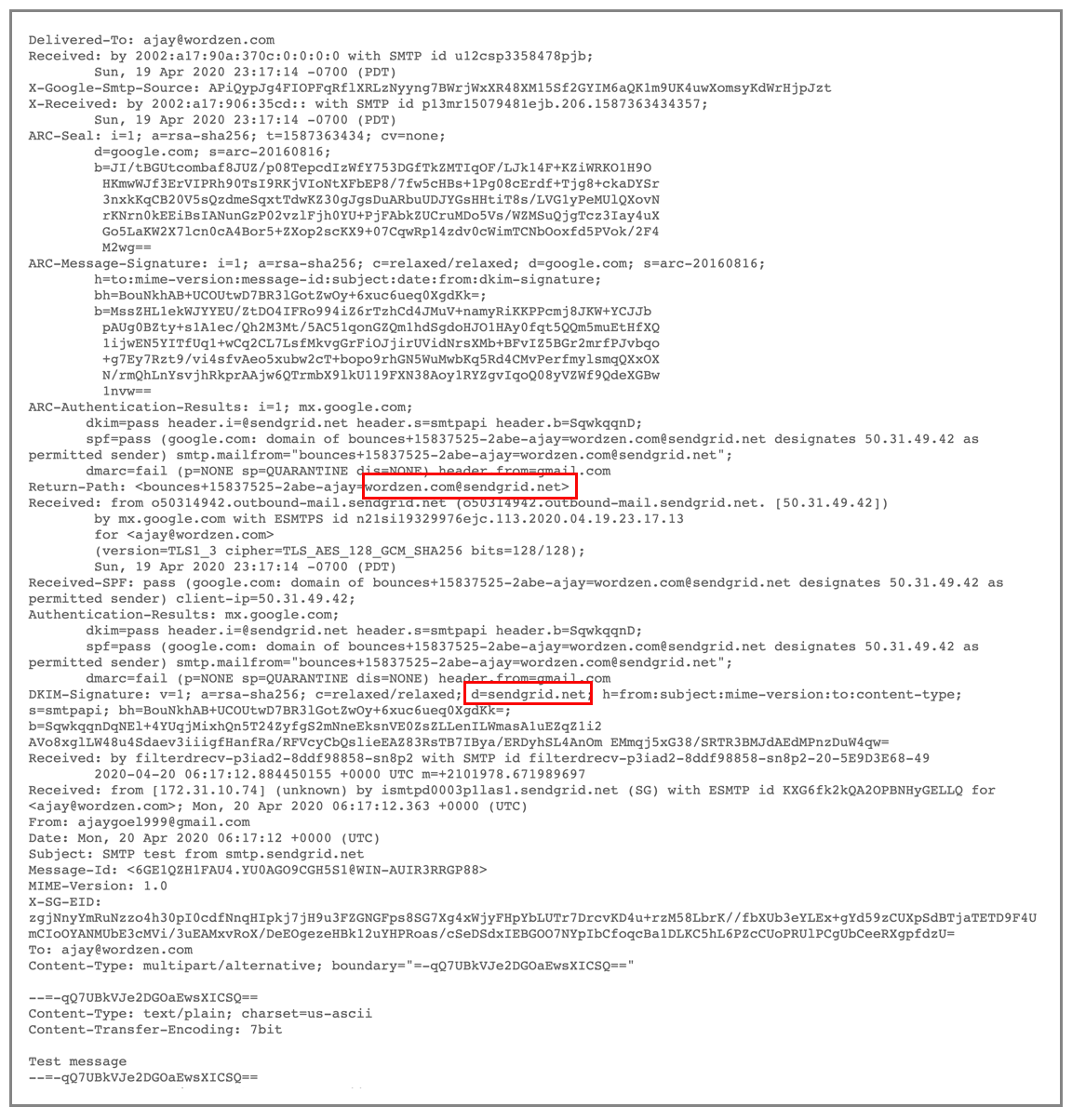
Now what happens if we add a whole domain. First, in their explanation of Domain Authentication, there’s a bit of BS:
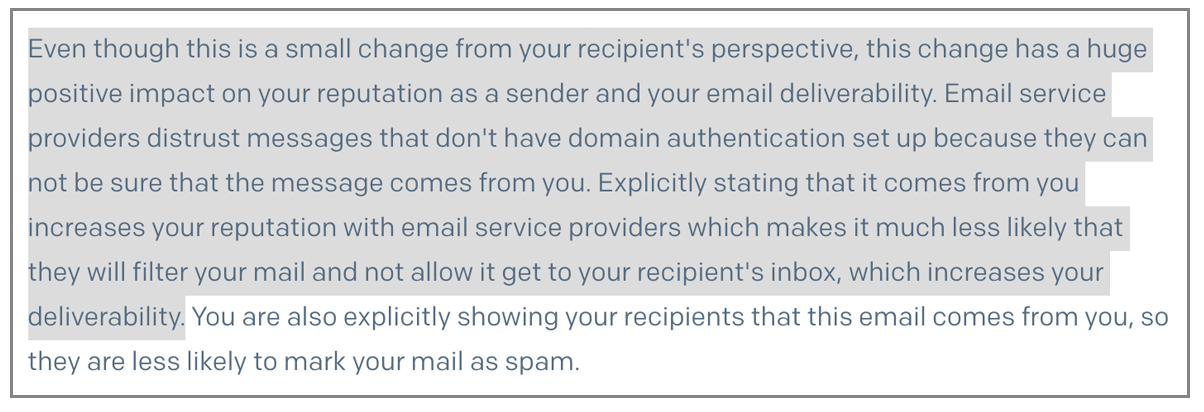
The likely reason SendGrid encourages you to do this is so that their domain, sendgrid.net, isn’t affected by YOUR mailings. The only way a typical recipient would know that the email wasn’t DKIM signed by the same domain is if they paid attention to theGmail “via” line, and if anything it would say “sendgrid.net” which is fairly well-known and trusted. People mark email spam because of the content, not because of the domain from where it originated.
Sloppy UI testing. I chose “I’m not sure” for my DNS provider, and look at what it tells me:
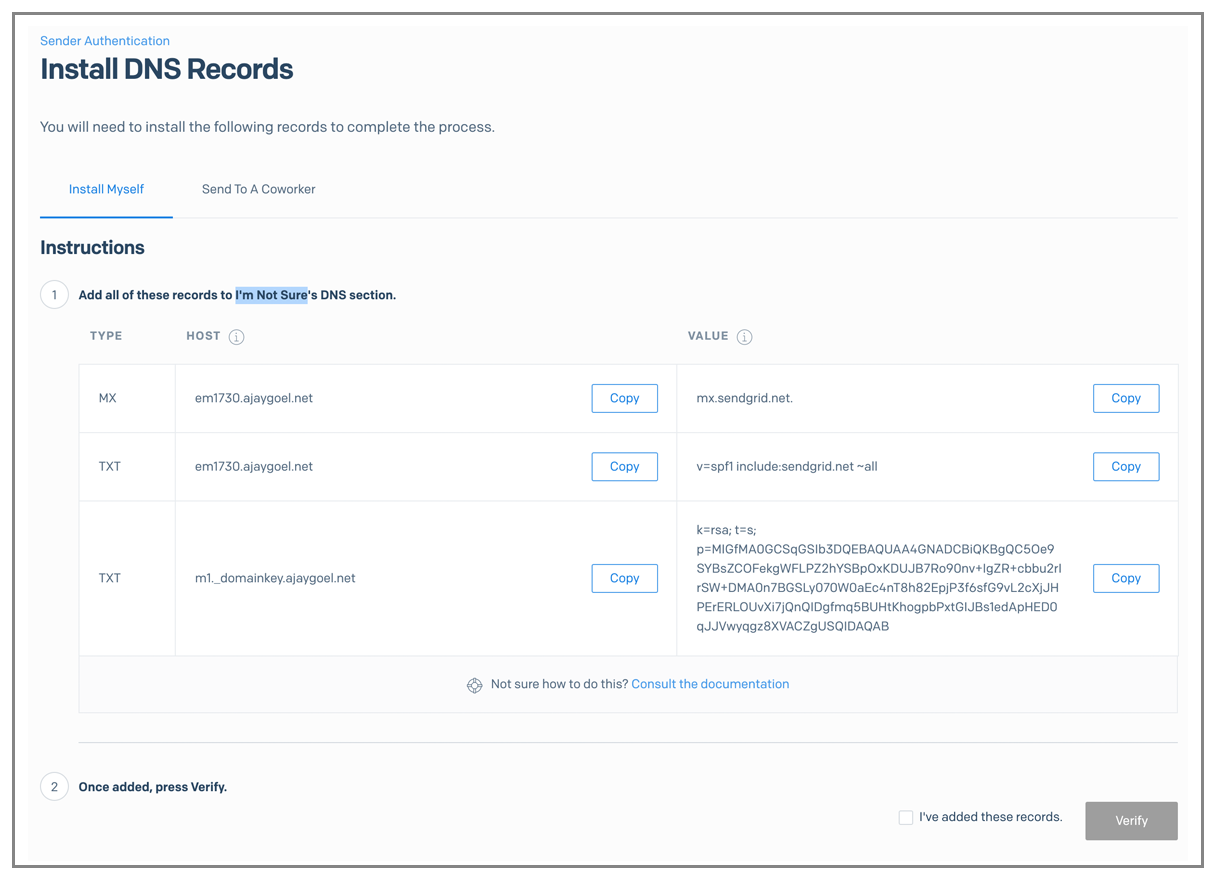
I go to set up ajaygoel.net and uncheck all the options, because I want the most basic of all basic setups, I just want to quickly send “from” ajaygoel.net and be done.
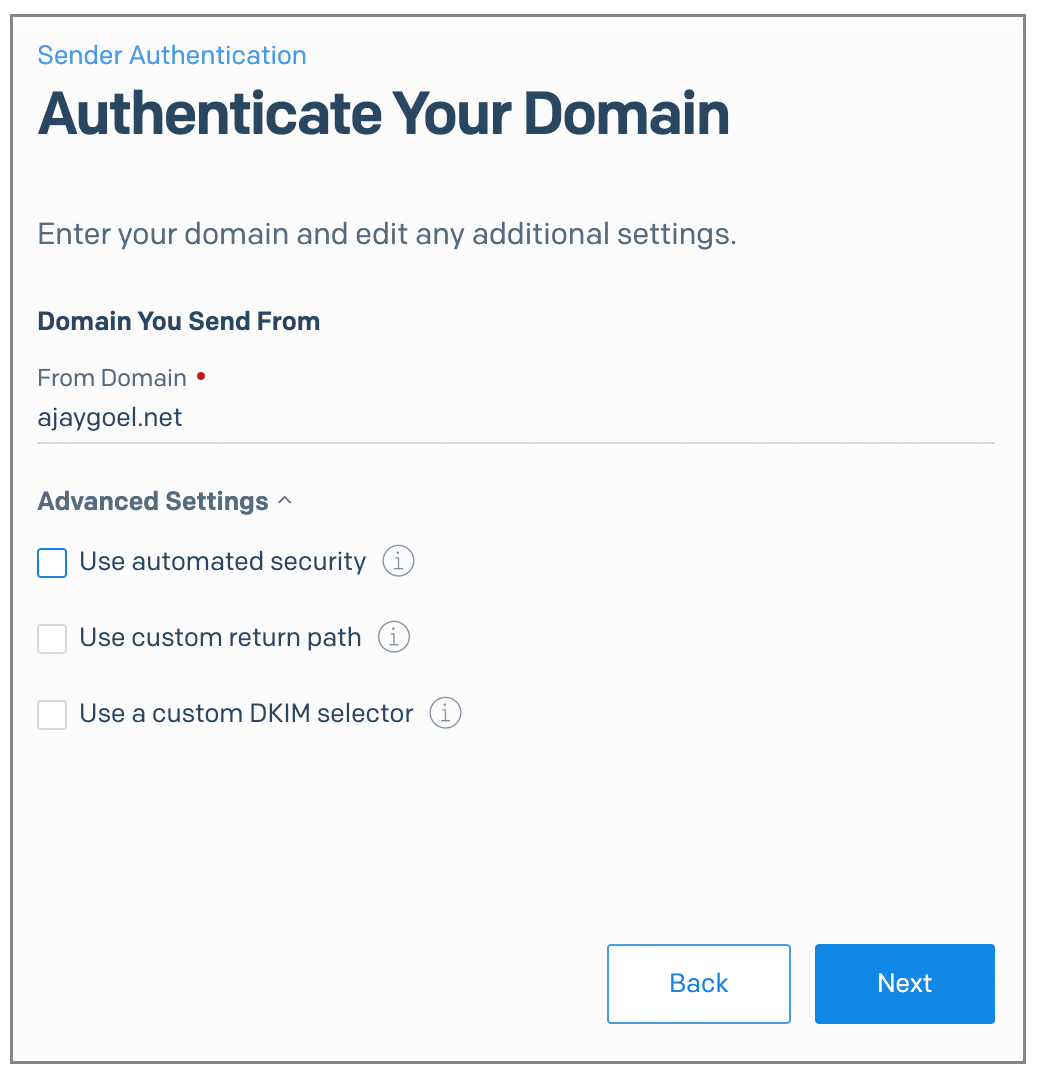
Alright I”ll play along and set up these DNS records.
So I do, and as expected, the MAIL-FROM is now ajaygoel.net and the DKIM signature uses it too:
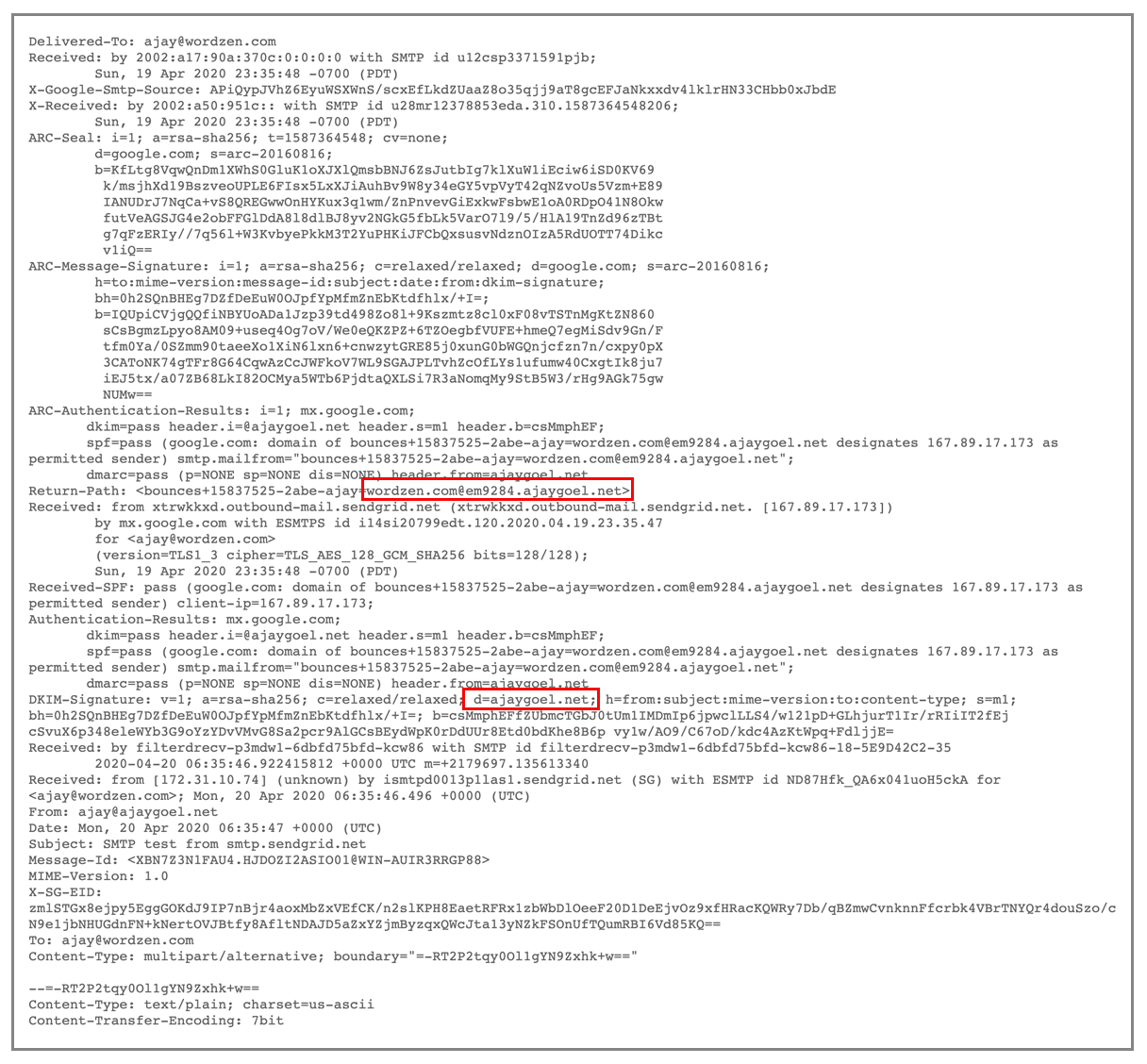
Another UI mistake: there’s a SAVE button that doesn’t do anything, but if I uncheck and check the “default” option, it does actually get saved, so why is there a Save button that won’t activate?
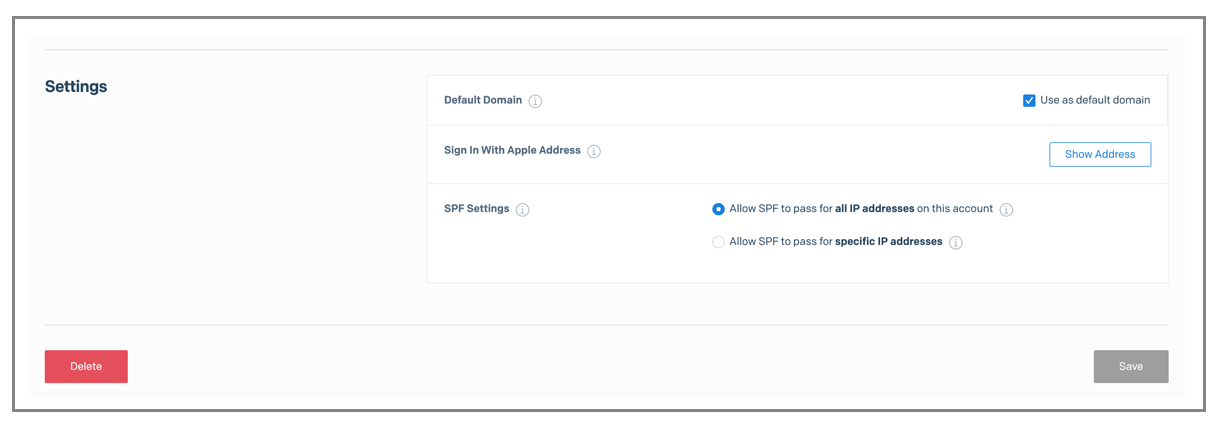
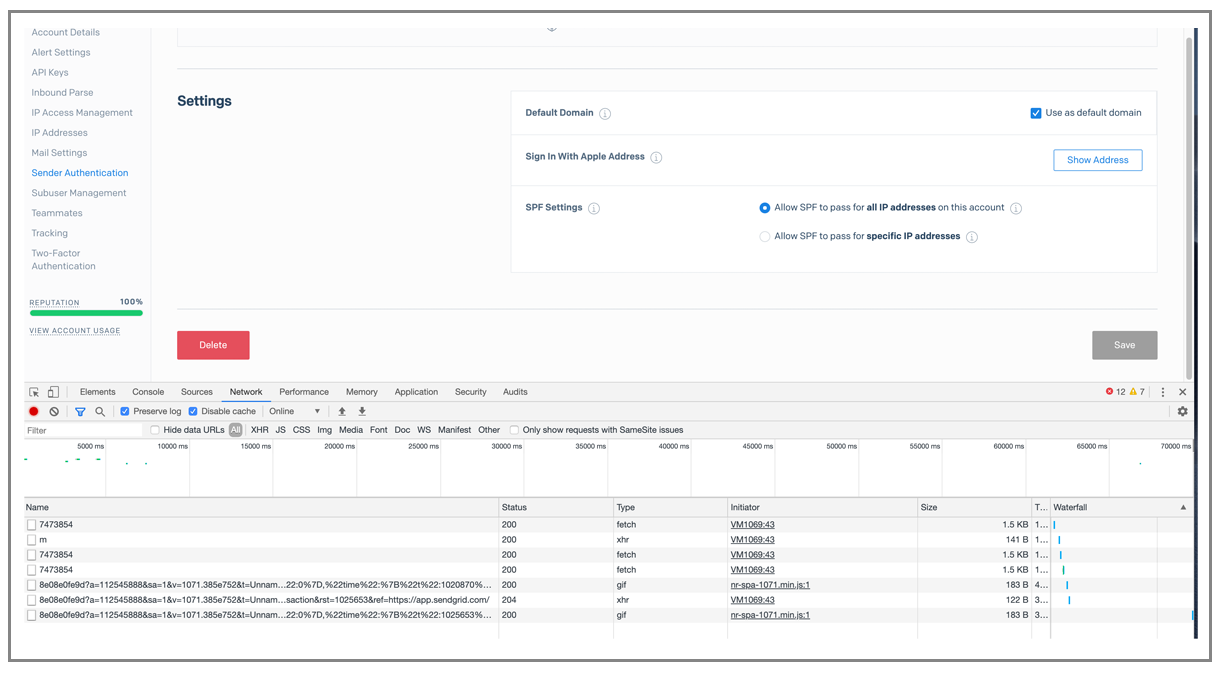
The “default” option is a beneficial setting that I haven’t seen with any other service. It lets me use the MAIL-FROM and DKIM signing of this domain for every email I send, even the ones that aren’t “from” this domain. For example, if I know send “from” [email protected]
Boom, it worked. It just didn’t take effect right away, had to wait 2-3 minutes
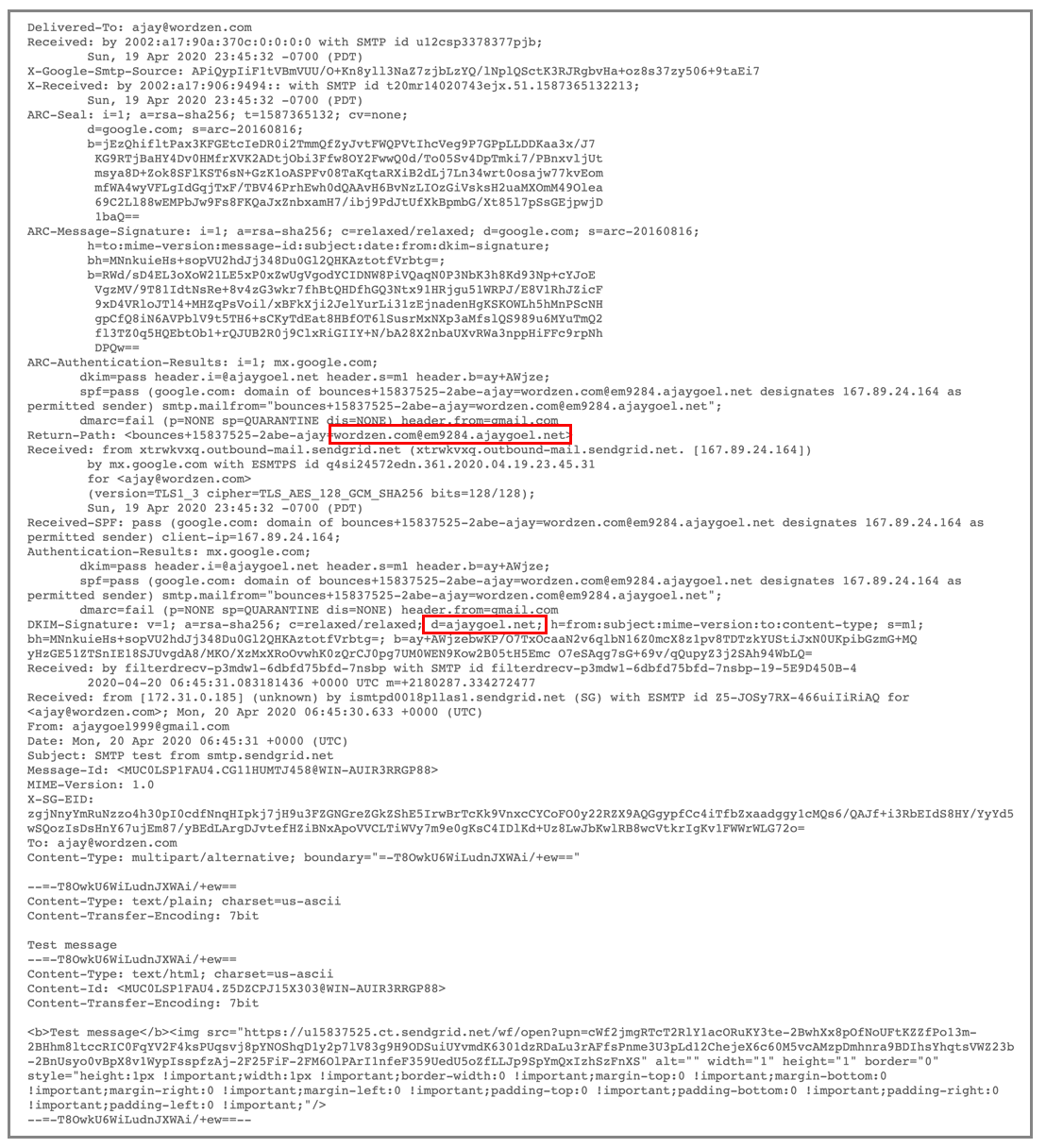
In case my explanation is unclear, here’s their explanation for the default domain:
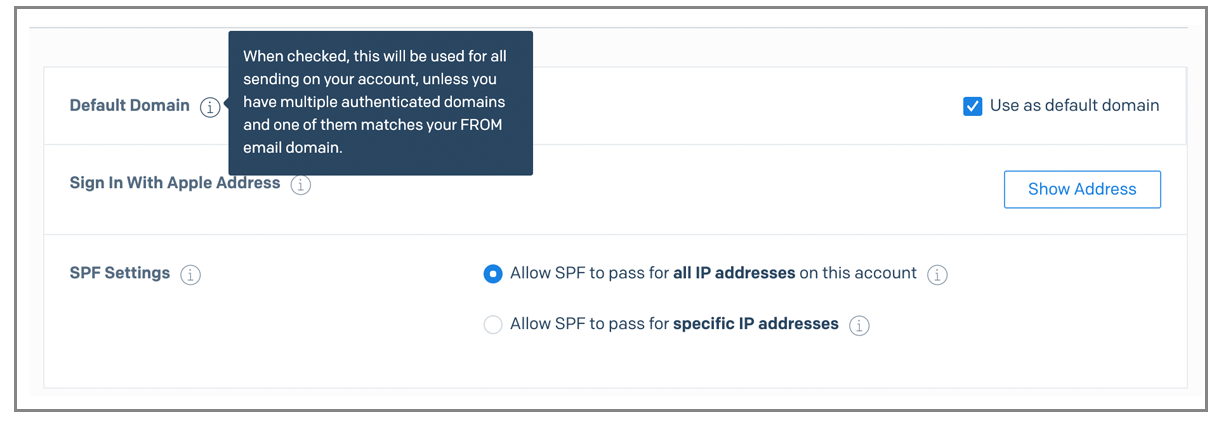
Another weird UI quirk. I set up the domain “ajaygoel.net” but it shows me the domain as em9284.ajaygoel.net…but that’s just the sub-domain it chose at random to use as the MAIL FROM domain (dkim is still signed with pure ajaygoel.net).
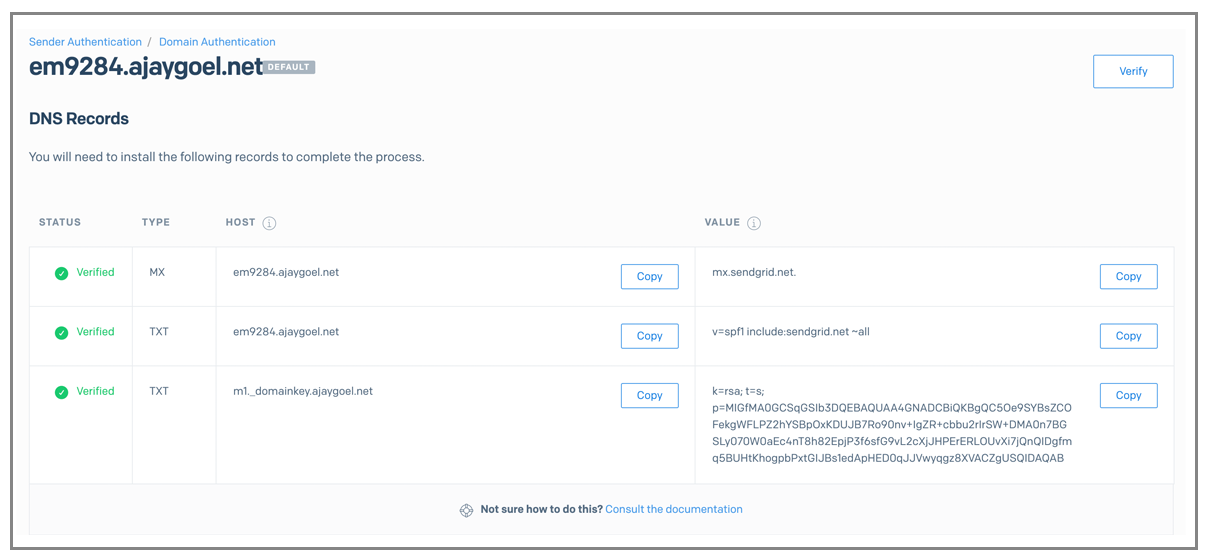
The skinny on SendGrid
Assuming you set up your SendGrid account after April 6, 2020:
- Lots of UI quirks
- To send anything, you have to verify an address or a domain, and verification does require an email link to be clicked on
- If you just verify an address, SendGrid will use its own domain for MAIL-FROM and DKIM signing, which is nice because it makes you pass both out-of-the-box without any DNS records.
- If you verify a whole domain, you’ll be forced to enter DNS records which means your MAIL-FROM and DKIM will be based on your own domain.
- So if you want stake your deliverability on SendGrid’s domain reputation and not your own, don’t verify a whole domain, just verify individual addresses @ the domain
- A useful feature i haven’t seen elsewhere (except for Amazon SES), is the ability to set a default domain once you verify a domain so that ALL MAIL, EVEN FROM @GMAIL.COM WILL BE SIGNED AND USE MAIL FROM OF THAT DOMAIN. except for any other domain that you’ve verified individually
Grade: B
Their sending infrastructure is really nice, and they have the best automated systems to review accounts and make sure you’re sending legit mail. You don’t have to tell some support human what you’re sending so that they let you send the limits on your plan. They are such a big company now that their huge dev team is likely a hindrance. Their web UI team isn’t as good as their SMTP infrastructure team and perhaps they don’t work well together, which is the reason for all the UI quirks. Their back-end has some bugs, as evidenced by the irrelevant PTR record error when trying to send to my domain @emailtest.io.
SendGrid punishes you for sending high volume email over their shared IPs. How? Their “Essentials 100K” plan lets you send 100,000 emails for $30/month, which comes to 3/100 of a penny per email. In other words, for one cent, you can send about 30 emails. But if you go over 100,000 emails, it’s $0.0023 per email, which means 0.23 cents per email, and .23 cents is about 8x the original rate you pay. Now that’s an overage rate if I’ve ever seen one. I personally send about 4,000,000 emails through their shared IPs, on behalf of my users, every month. This results in a bill of over $4,000 every month. You would think that the price per email goes down as volume increases, but with their shared IP plan, the opposite is true.
Read my non-technical SendGrid review.
Amazon SES
(back to top)
Before you can send anything with SES, you have to verify a new domain and get yourself out of their “sandbox”, otherwise you get this:
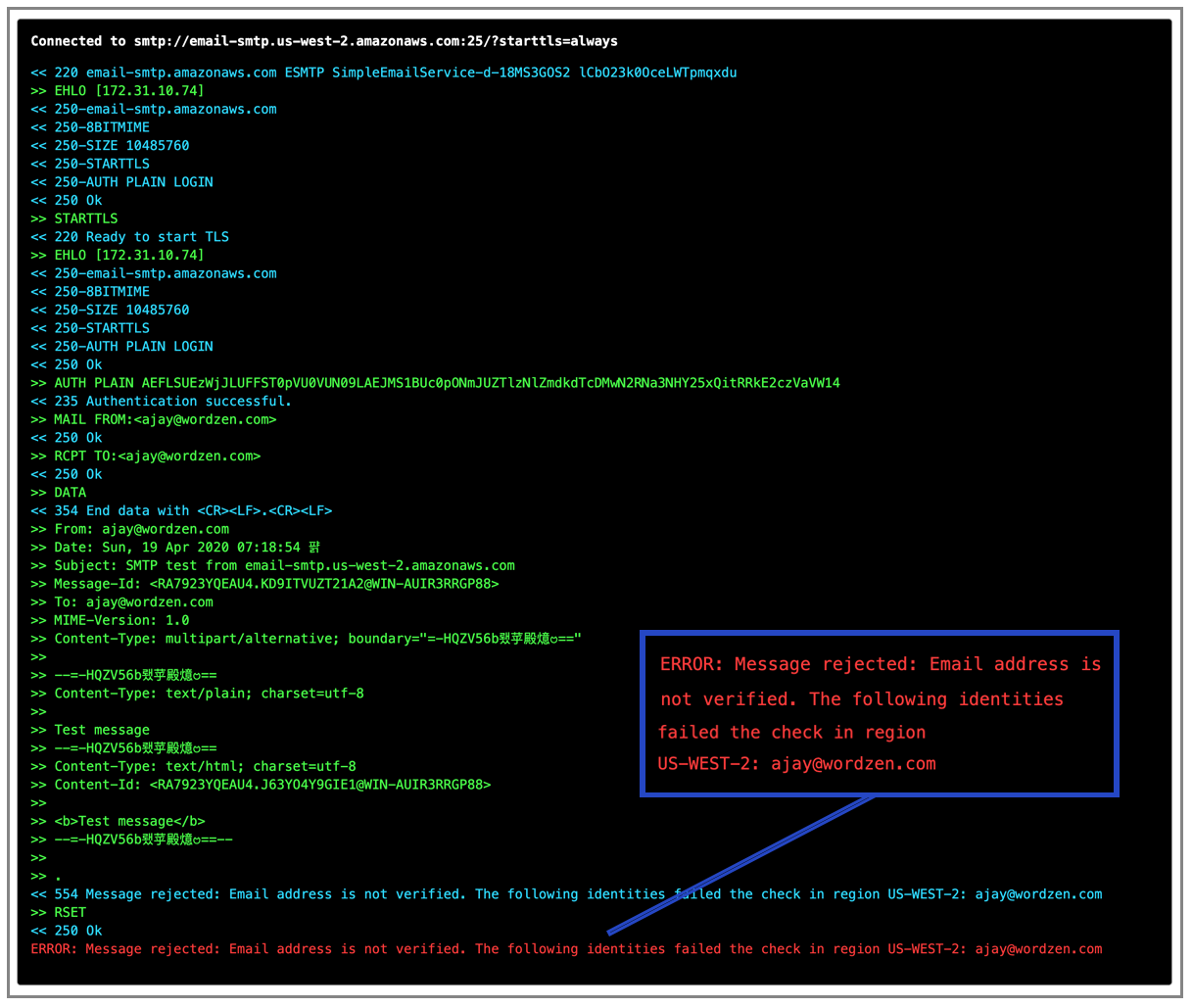
So I’ll go ahead and verify “ajaygoel.net”
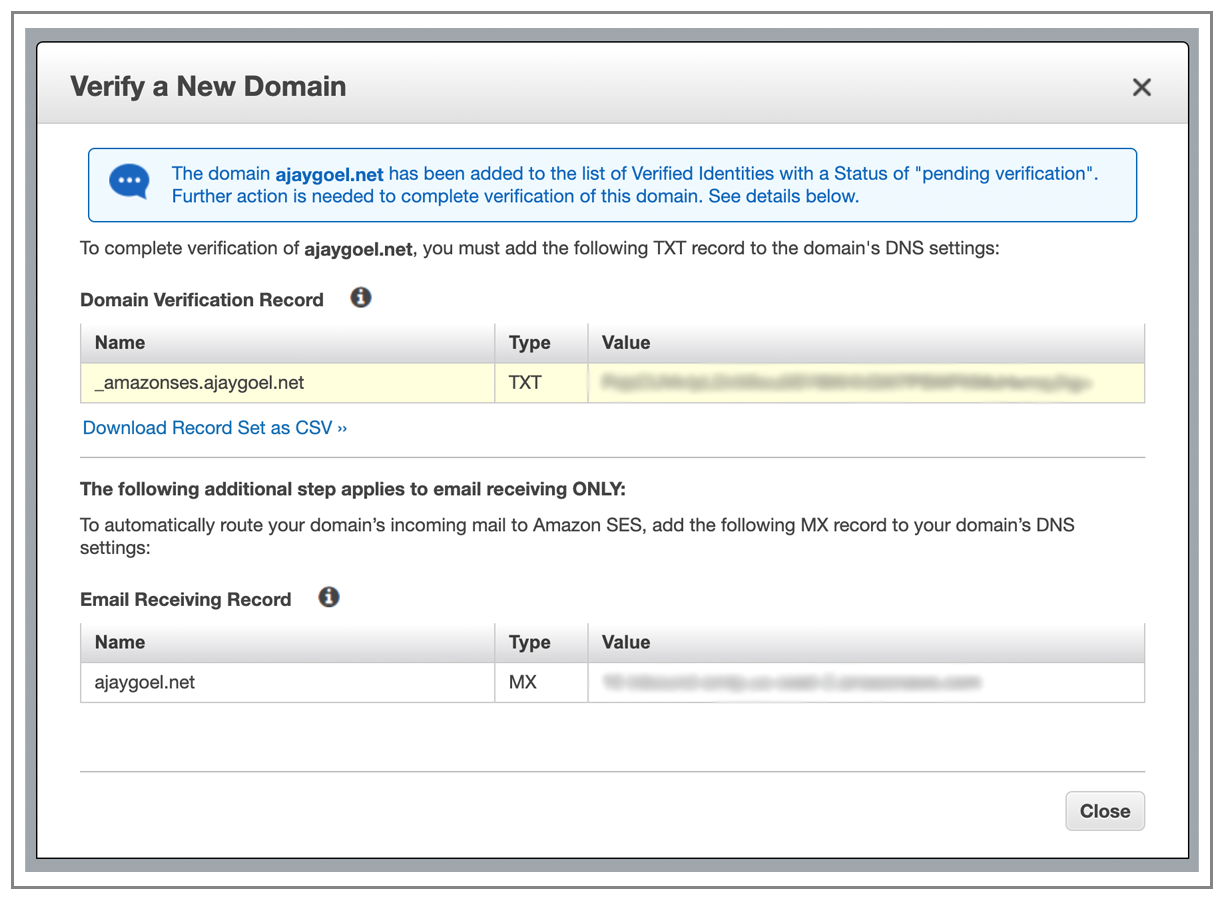
Turns out that recipients have to be verified too, when you first start out, because you start out in Amazon SES Sandbox mode
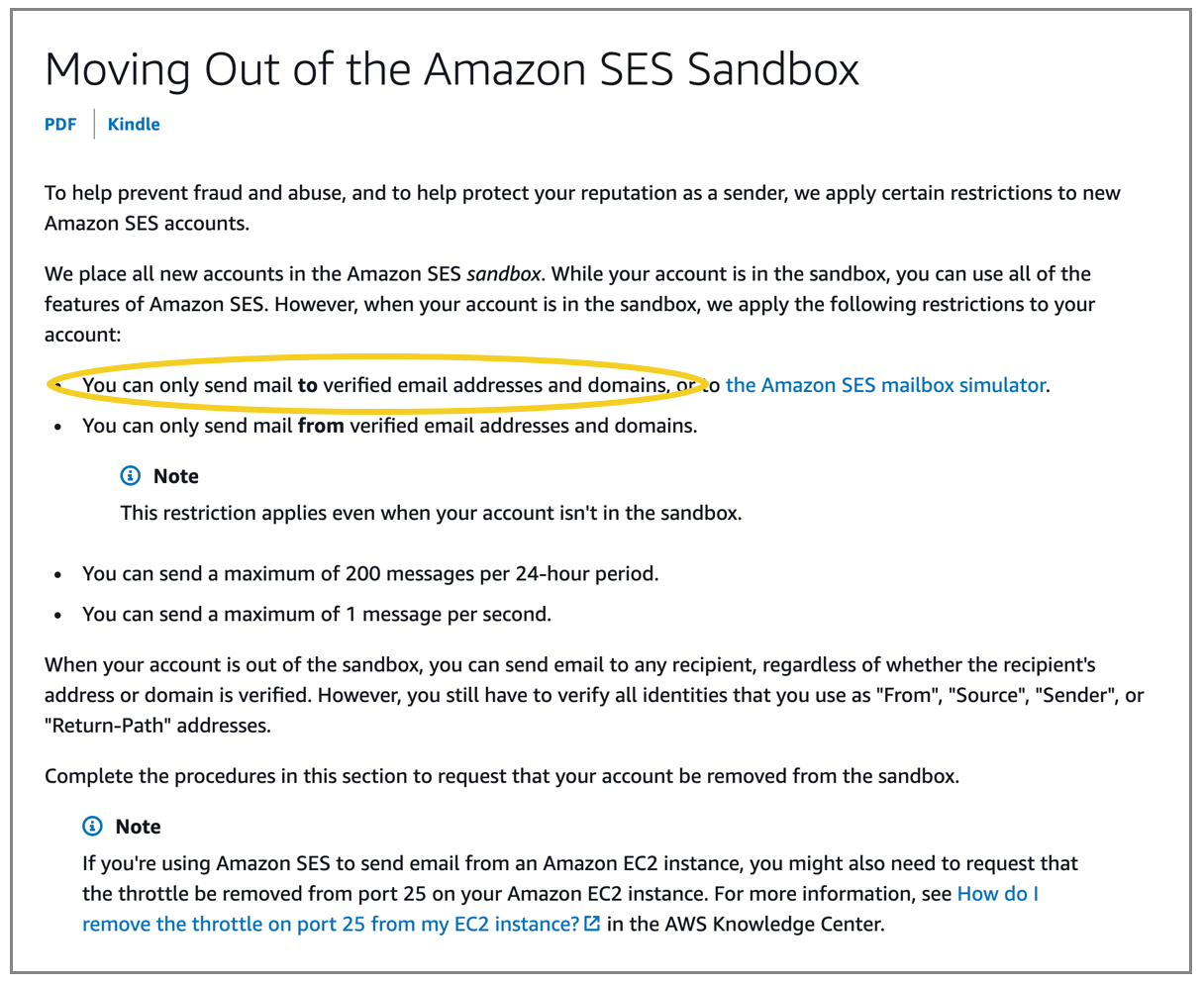
Can’t do much testing until I get out of the sandbox. IT took less than 24 hours to get out of the Sandbox. In fact, I had only asked for 1,000 emails/day, and to my pleasant surprise, I was granted 50,000 emails/day.
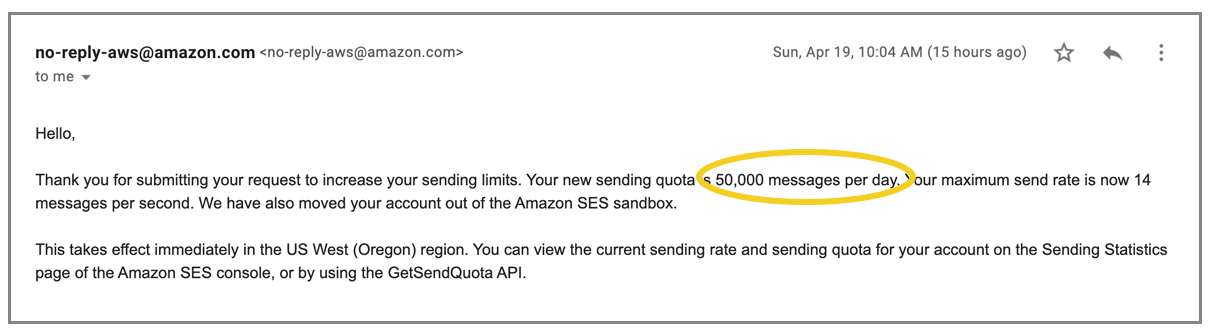
Now let’s explore the service.
Looks like even out of the sandbox, every address/domain you want to send “from” has to be verified. If you don’t, you get this error. Here’s me sending “from” [email protected].
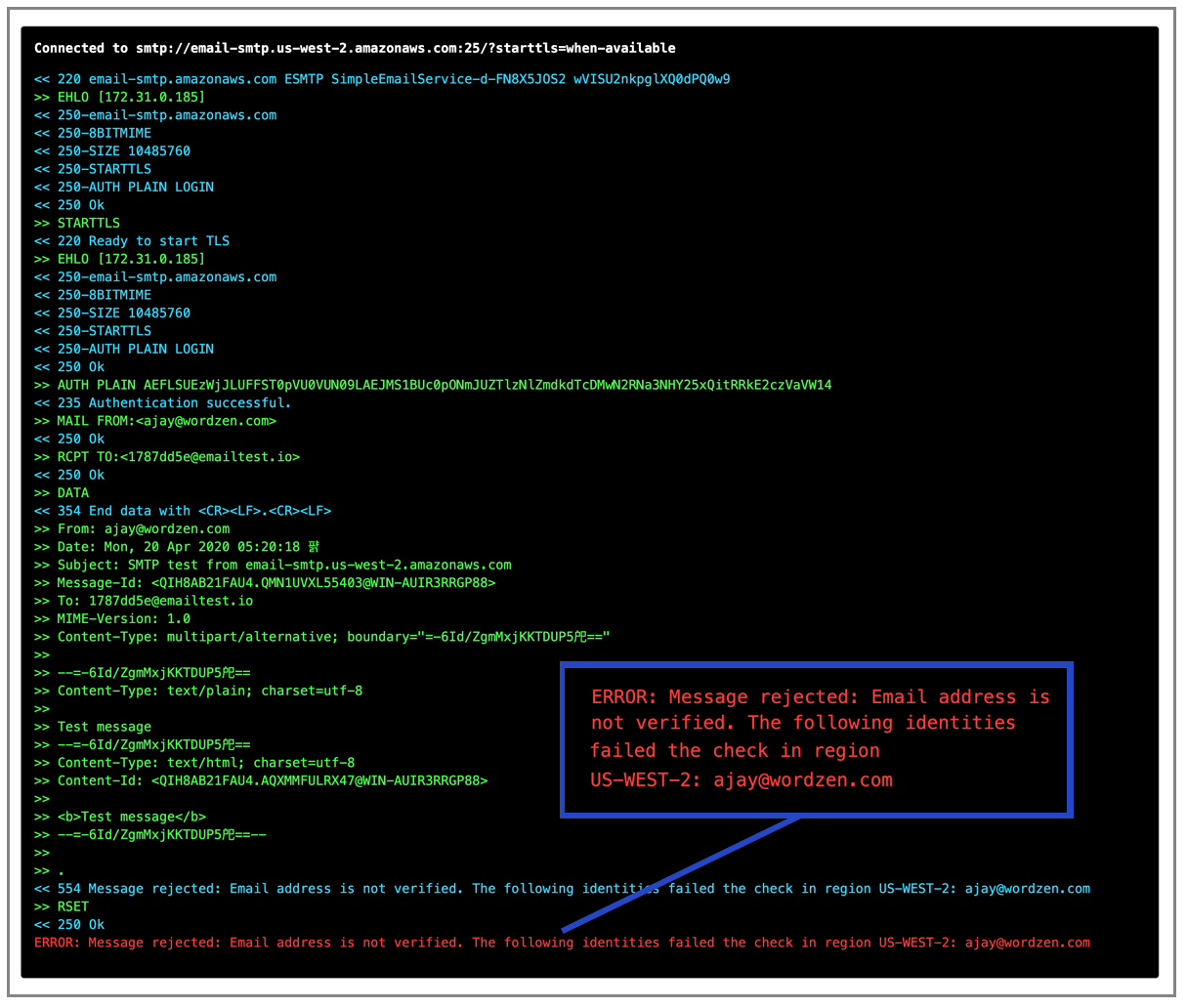
And here’s me trying to send “from” an @gmail.com account:
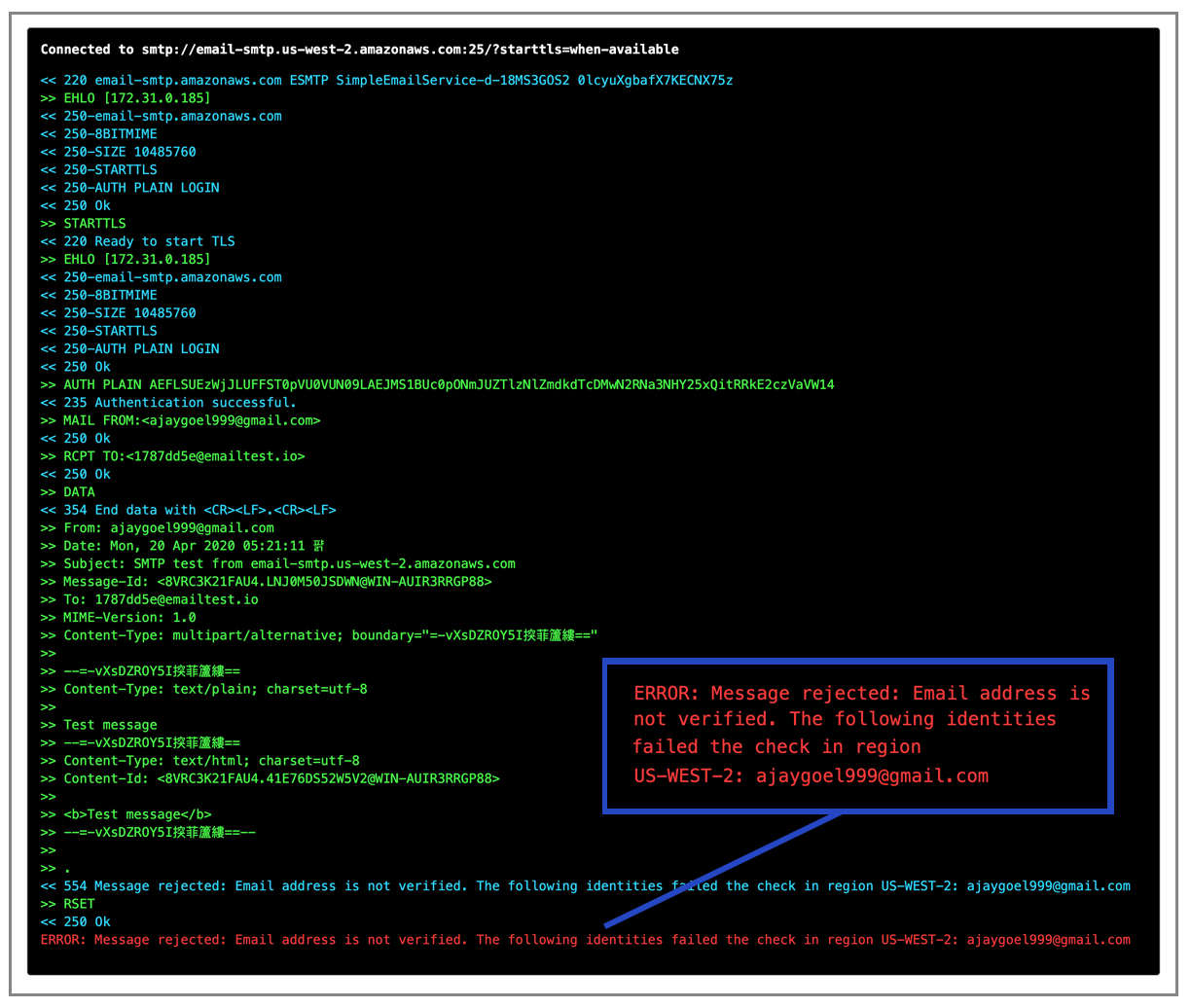
Weird quirk — when I go to verify an individual email address, it sends a verification email to that address, BUT it states that it may take up to an hour. An hour? Amazon operates its own darn transactional email service, and it can’t send its own transactional email right away? weird.
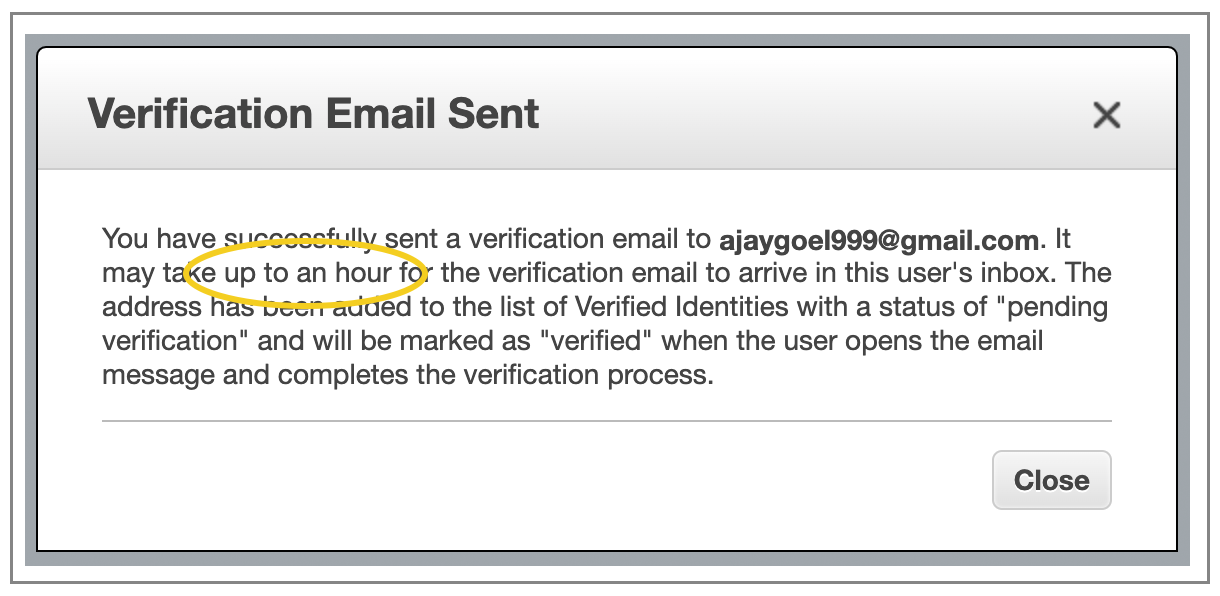
In reality, the email arrived right away, so that makes the message all the more strange.
After verification, I CAN send from my @gmail.com account.
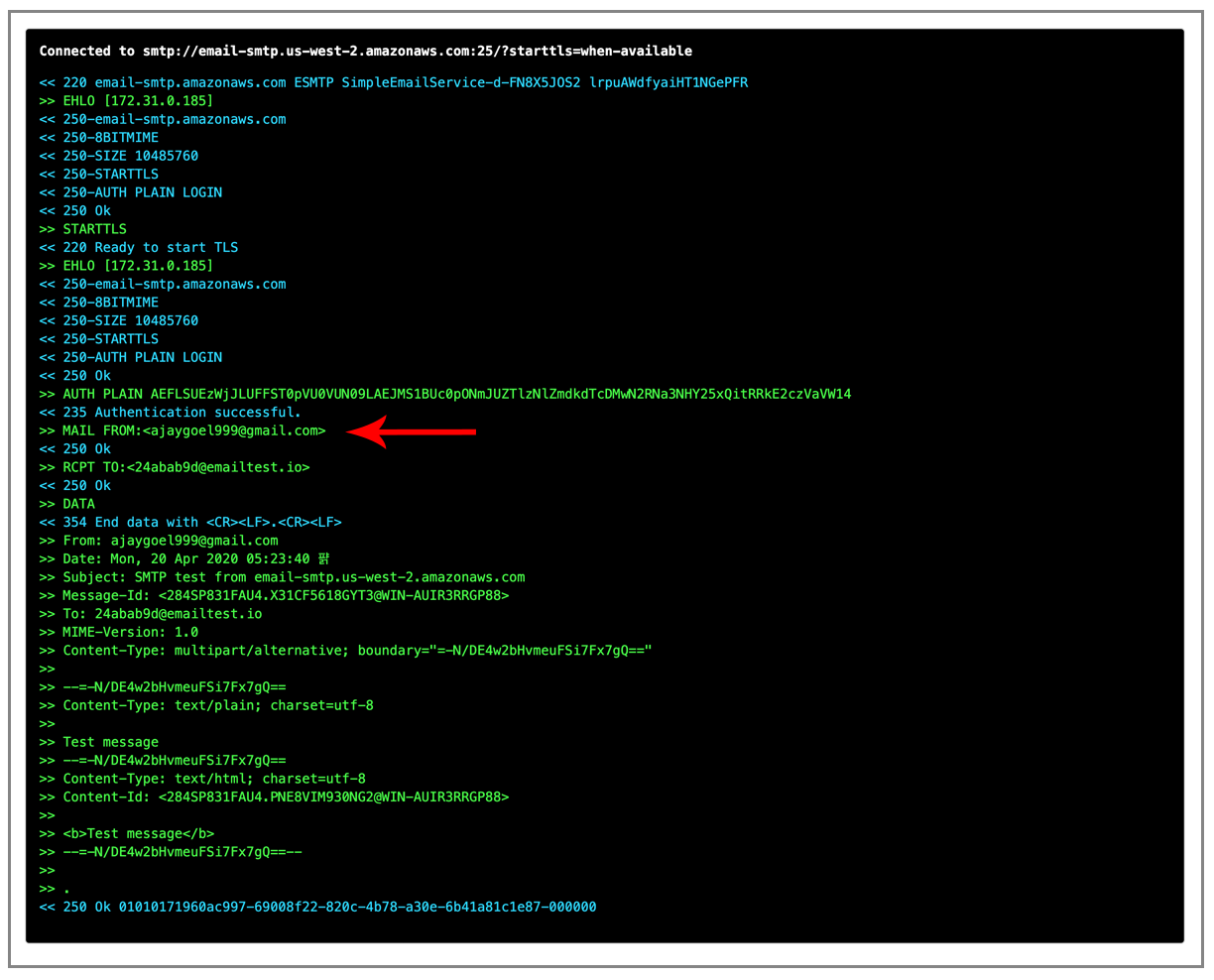
And the resulting email is DKIM signed by the domain amaoznses.com with a MAIL FROM of @us-west-2.amazonses.com
This is to be expected, since with a from address that is gmail.com, there’s no way for DKIM or MAIL-FROM to be off my own domain, unless it let me set a general domain for DKIM signing and MAIL-FROM for these purposes that isn’t gmail.com.
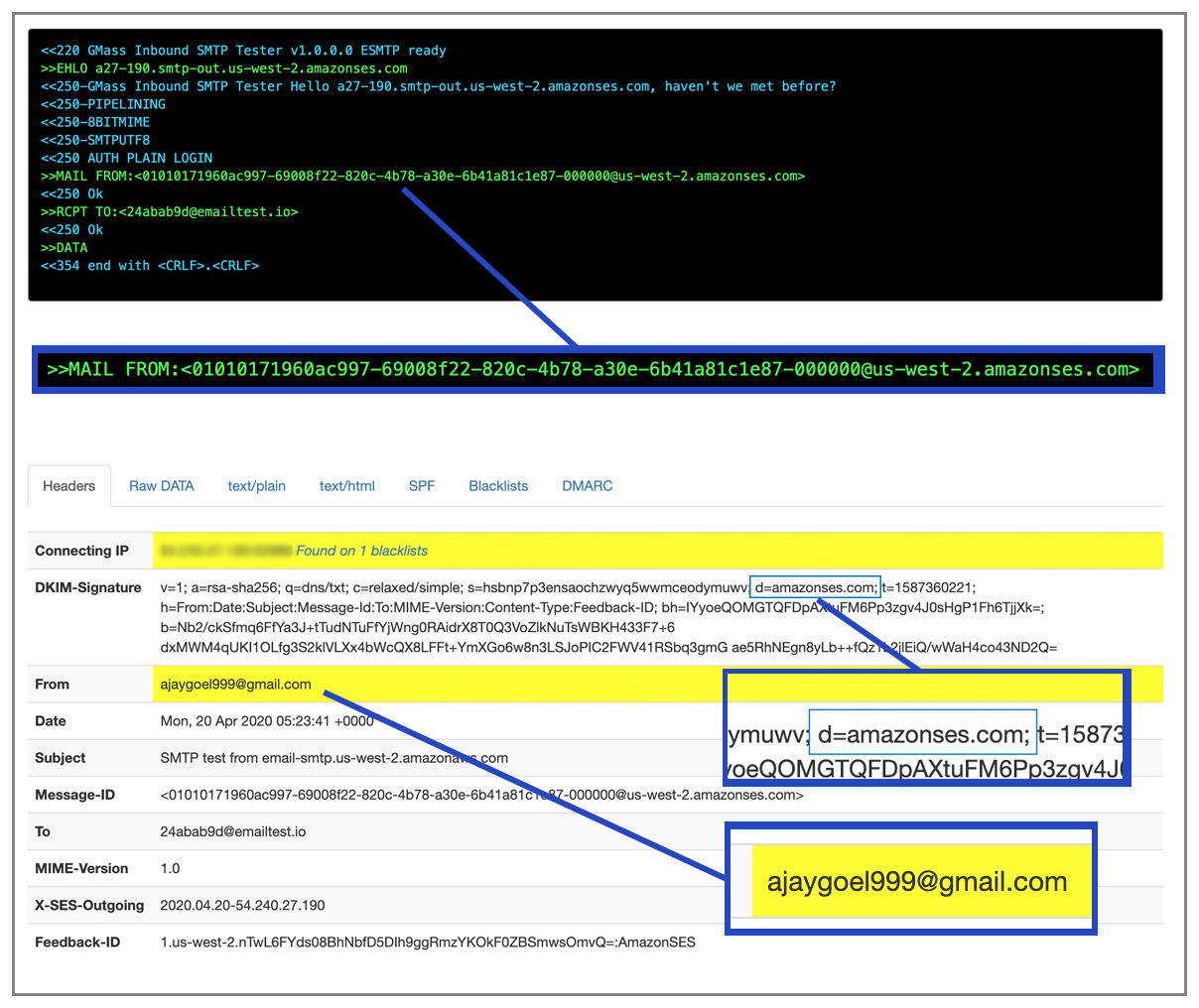
Anyway, I have also verified ajaygoel.net, so what does that look like?
Simply verifying it just allows me to send the email, and doesn’t alter the MAIL-FROM OR the DKIM signing away from amazonses.com.
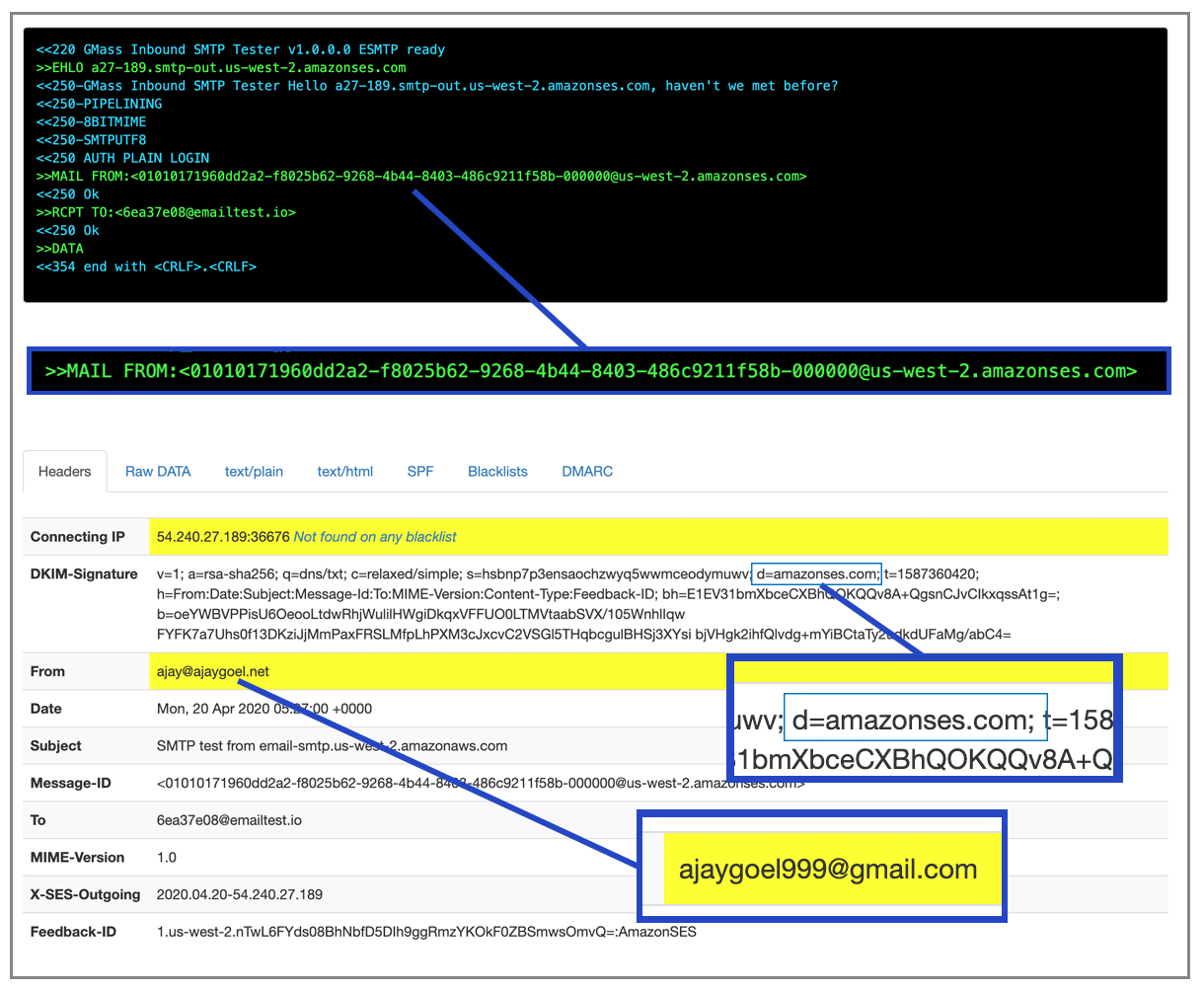
How can I control the MAIL-FROM and DKIM signing?
It walks you through a process of controlling the MAIL-FROM:
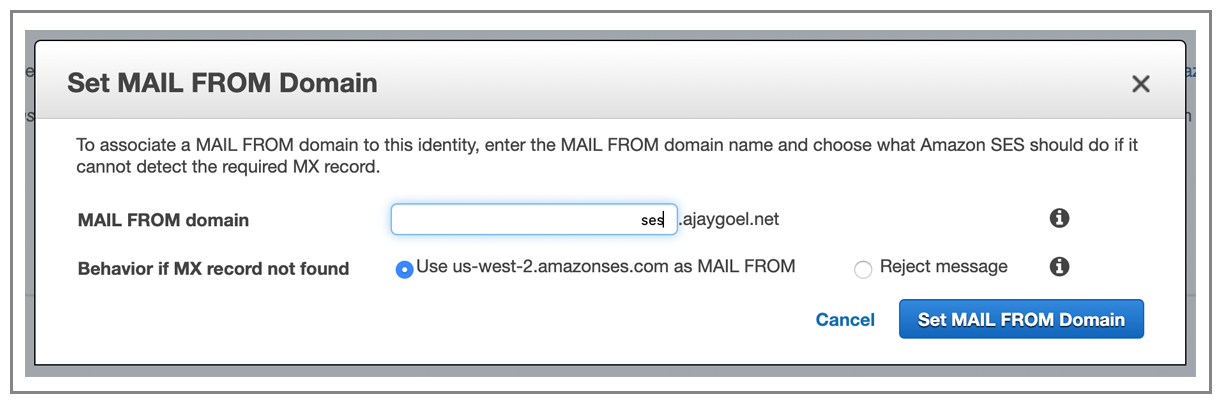
Interestingly though, it doesn’t let you use your ROOT domain as your MAIL-FROM, YOU HAVE TO create a sub domain. I’ll choose ses.ajaygoel.net
You add the MX and click the “verify” link and you’re all set.
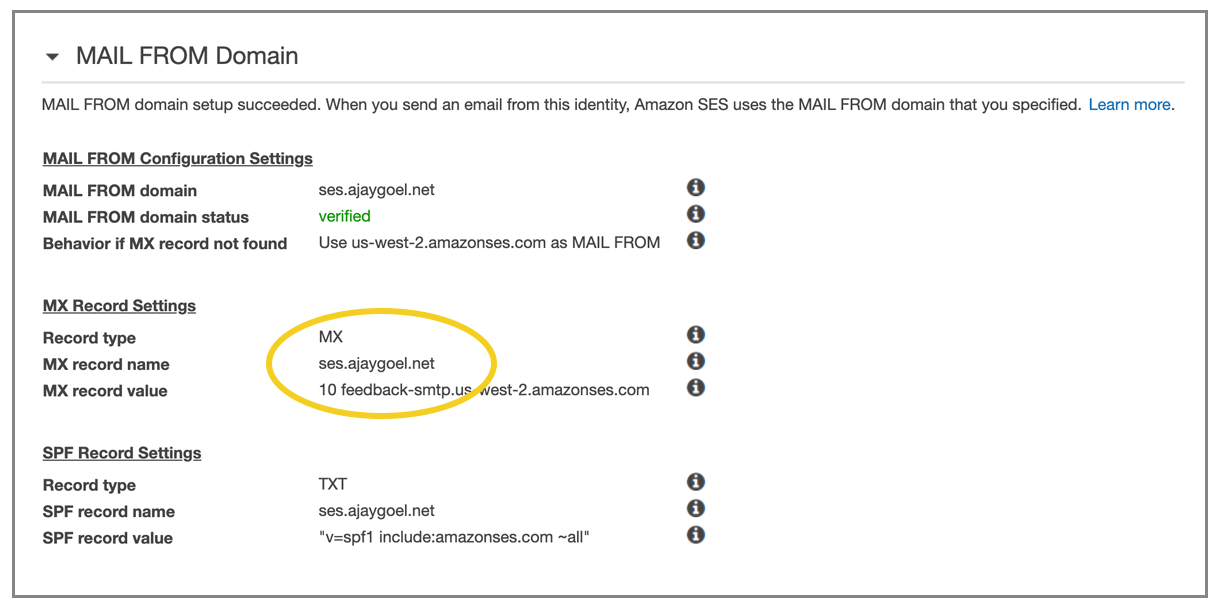
Now let’s test again.
Now the MAIL-FROM IS ajaygoel.net
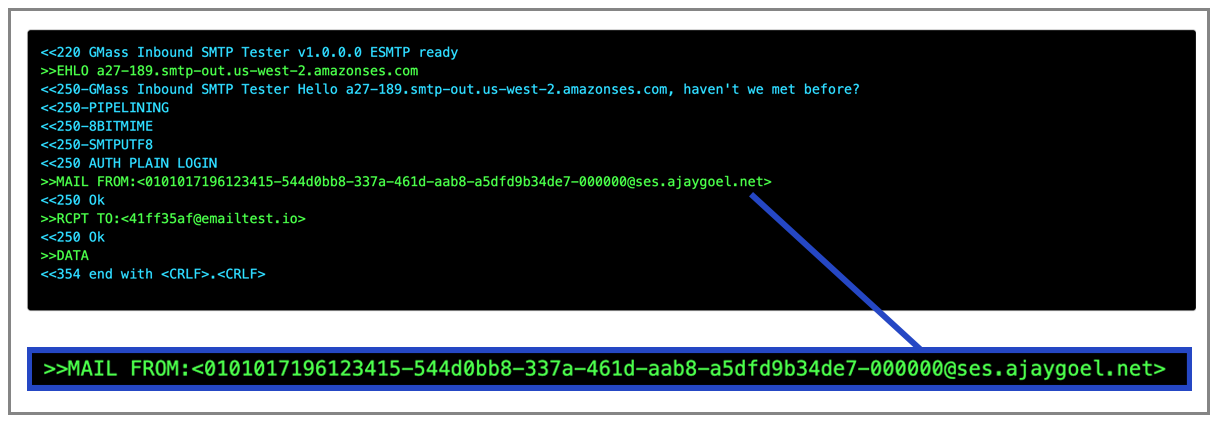
It’s still DKIM-signed by amazonses.com, but we can fix that.
Fixed, and now, SES signs it twice, once with its own domain of amazonses.com and once with my domain ajaygoel.net
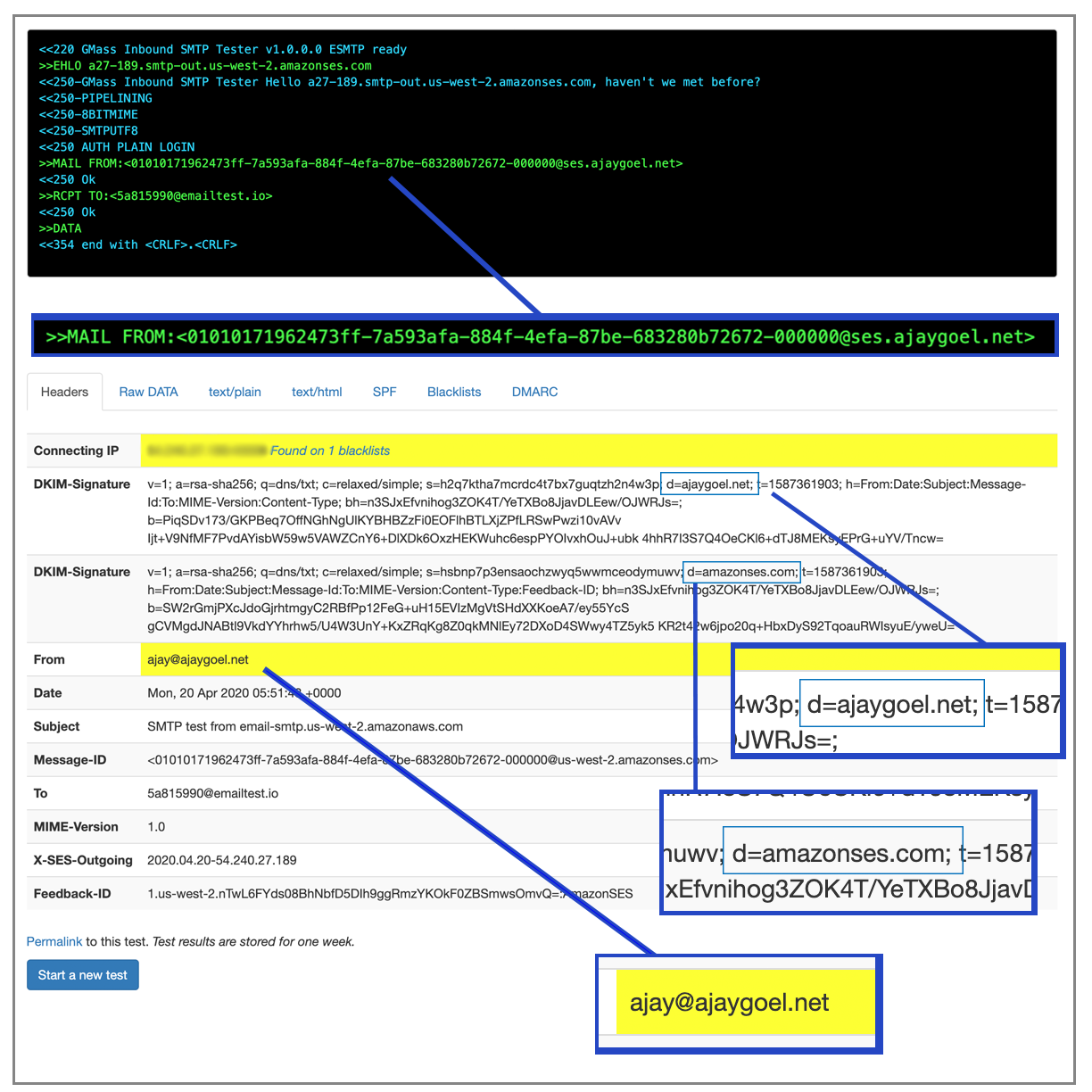
So, pretty good overall. Once verified, you can send “from” anybody, and SES will use its own MAIL-FROM and DKIM sign using its own domain.
For the DKIM setup, it gives you 3 CNAME records to enter;
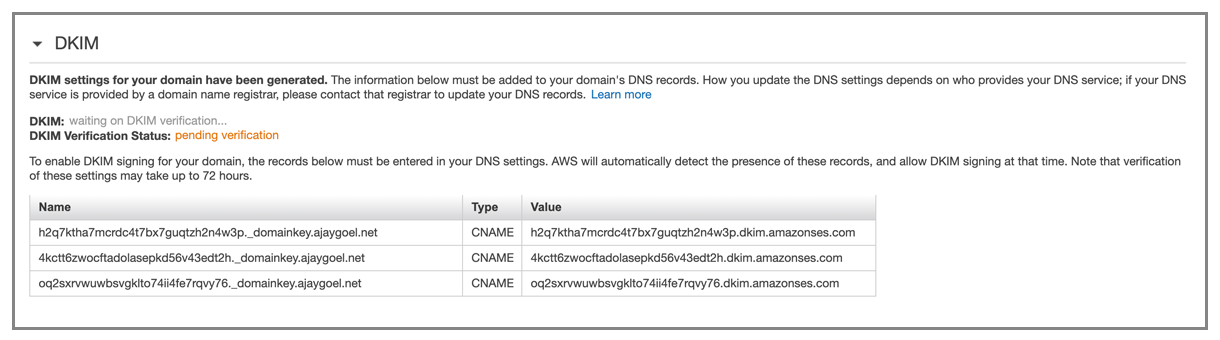
I wish:
- I could send “from” without the complex verification procedures per email address and per domain.
- I could sign all my emails with any domain that I’ve verified with them, even if the “from” isn’t for that domain.
The UI is fantastic, as is most of AWS
Weird though that you can’t copy/paste those DNS records, like you can easily copy/paste IP addresses and and other identifiers throughout aWS.
Grade: B
See why GMass has 300k+ users and 7,500+ 5-star reviews
Email marketing. Cold email. Mail merge. Avoid the spam folder. Easy to learn and use. All inside Gmail.
TRY GMASS FOR FREE
Download Chrome extension - 30 second install!
No credit card required
Ajay is the founder of GMass and has been developing email sending software for 20 years.
Love what you're reading? Get the latest email strategy and tips & stay in touch.